要用Python画一个六角星,可以使用turtle、matplotlib库、PIL库等方法。最简单和直观的方法是使用turtle库,因为它专门用于绘制图形。
turtle库通过简单的命令可以画出各种图形、具有易用性和高可操作性。
下面是关于如何用Python画一个六角星的详细步骤和相关知识。
一、使用turtle库绘制六角星
1、安装和导入turtle库
turtle库通常是Python标准库的一部分,不需要额外安装。可以直接在代码中导入:
import turtle
2、设置绘图环境
在开始绘图之前,我们需要设置画布和画笔的环境。例如,可以调整画笔的颜色、粗细等属性。
turtle.bgcolor("white") # 设置背景颜色
turtle.pensize(2) # 设置画笔粗细
turtle.color("black") # 设置画笔颜色
3、绘制六角星
六角星由两个交错的等边三角形组成。我们可以通过如下步骤绘制:
def draw_hexagon(side_length):
for _ in range(6):
turtle.forward(side_length)
turtle.right(60)
def draw_star(side_length):
for _ in range(6):
turtle.forward(side_length)
turtle.right(144) # 外角为144度
turtle.penup()
turtle.goto(-50, 0) # 移动到起始位置
turtle.pendown()
draw_star(100) # 绘制六角星,边长为100
turtle.done() # 完成绘图
二、使用matplotlib库绘制六角星
1、安装和导入matplotlib库
如果还没有安装matplotlib,可以使用以下命令安装:
pip install matplotlib
然后在代码中导入:
import matplotlib.pyplot as plt
import numpy as np
2、绘制六角星
使用matplotlib可以更灵活地控制图形的属性。下面是一个用matplotlib绘制六角星的示例:
def plot_star(ax, radius, color):
angles = np.linspace(0, 2 * np.pi, 7)
points = np.array([np.cos(angles), np.sin(angles)]).T * radius
star_points = points[::2] + points[1::2]
ax.plot(star_points[:, 0], star_points[:, 1], color=color)
fig, ax = plt.subplots()
ax.set_aspect('equal')
ax.axis('off')
plot_star(ax, 1, 'blue')
plt.show()
三、使用PIL库绘制六角星
1、安装和导入PIL库
PIL(Pillow)库可以处理图像文件,并进行绘图操作。如果还没有安装PIL,可以使用以下命令安装:
pip install Pillow
然后在代码中导入:
from PIL import Image, ImageDraw
2、绘制六角星
使用PIL绘制六角星的示例如下:
def draw_star(image, center, radius, fill_color):
draw = ImageDraw.Draw(image)
angles = np.linspace(0, 2 * np.pi, 7)
points = [(center[0] + np.cos(angle) * radius, center[1] + np.sin(angle) * radius) for angle in angles]
star_points = points[::2] + points[1::2]
draw.polygon(star_points, fill=fill_color)
image = Image.new('RGB', (200, 200), 'white')
draw_star(image, (100, 100), 80, 'blue')
image.show()
四、总结
1、turtle库
turtle库适用于初学者绘制简单图形、直观、易操作。
2、matplotlib库
matplotlib库适用于绘制复杂图形、数据可视化、具有较高的灵活性。
3、PIL库
PIL库适用于处理图像文件、绘制图形、丰富的图像处理功能。
以上三种方法各有优缺点,根据具体需求选择合适的方法。使用这些库绘制图形不仅可以提高编程能力,还能加深对几何图形的理解。
相关问答FAQs:
如何用Python绘制六角星的基本步骤是什么?
要使用Python绘制六角星,您可以使用图形库,例如Turtle或Matplotlib。首先,确保安装了相应的库。接着,通过设置画布和定义六角星的坐标,使用循环绘制六条边。最后,调用绘图命令来展示六角星。
使用Turtle库绘制六角星有什么技巧吗?
在使用Turtle库时,调整速度和颜色能够提高绘图效果。可以通过turtle.speed()
来设置绘图速度,使用turtle.color()
来改变线条颜色。此外,利用turtle.begin_fill()
和turtle.end_fill()
可以填充颜色,使六角星更加美观。
Python中有哪些其他库可以绘制六角星?
除了Turtle,Matplotlib也是一个强大的选择。通过使用Matplotlib的极坐标功能,可以绘制出六角星的外形。此外,Pygame和Pillow等库也可以用来创建更复杂的图形和图像,提供更多的绘图选项和效果。
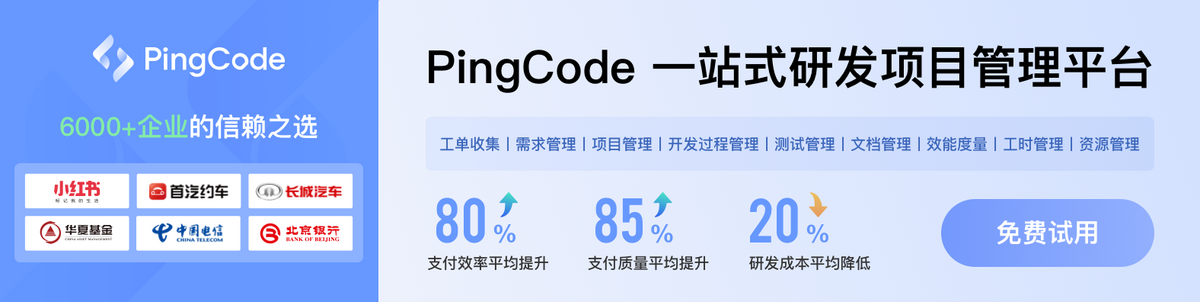