使用Python一次读取一行数据有多种方法,包括input()
函数、文件读取方法readline()
、以及使用pandas
库。最常用的方法是使用内置的input()
函数读取用户输入、readline()
读取文件中的特定行、以及pandas
库处理大型数据集。其中,使用readline()
方法从文件中读取特定行是最常见和高效的方式。
一、使用input()函数读取用户输入
input()
函数是Python内置的函数,主要用于从用户获取输入数据。它在控制台等待用户输入并将其作为字符串返回。
user_input = input("Please enter a line of data: ")
print(f"You entered: {user_input}")
二、使用readline()方法读取文件中的一行
readline()
是文件对象的一个方法,用于从文件中读取一行数据。它非常适合于处理较大的文件,因为它一次只读取一行,避免了内存占用过多的问题。
with open('example.txt', 'r') as file:
first_line = file.readline()
print(f"The first line of the file is: {first_line}")
三、使用pandas读取特定行数据
pandas
库提供了强大的数据处理功能,可以方便地读取和处理大型数据集。虽然读取单行数据不是其主要用途,但仍然可以使用它的强大功能来处理。
import pandas as pd
df = pd.read_csv('example.csv')
first_row = df.iloc[0]
print(f"The first row of the CSV file is:\n{first_row}")
四、文件读取的更多技巧
1、读取多行数据
有时我们可能需要读取多行数据,可以使用循环或者readlines()
方法。
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
2、逐行处理大型文件
对于非常大的文件,一次性读取整个文件会导致内存不足的问题。可以使用生成器逐行处理文件。
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
for line in read_large_file('large_file.txt'):
process(line) # 自定义的处理函数
五、处理不同格式的文件
不同格式的文件需要不同的处理方法。例如,JSON文件可以使用json
库,Excel文件可以使用pandas
的read_excel
方法。
import json
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
df = pd.read_excel('data.xlsx')
print(df.head())
六、处理错误和异常
在读取文件时,可能会遇到文件不存在或者读取失败的情况。需要使用异常处理来确保程序的健壮性。
try:
with open('non_existent_file.txt', 'r') as file:
content = file.readline()
except FileNotFoundError:
print("The file does not exist.")
except IOError:
print("An error occurred while reading the file.")
七、提高文件读取效率
对于大型文件,可以使用mmap
模块进行内存映射,以提高读取效率。
import mmap
with open('large_file.txt', 'r+') as file:
mmapped_file = mmap.mmap(file.fileno(), 0)
line = mmapped_file.readline()
print(line.decode('utf-8'))
mmapped_file.close()
八、总结
无论是通过input()
函数获取用户输入、使用readline()
读取文件中的一行、还是使用pandas
库处理大型数据集,Python提供了灵活多样的方式来读取一行数据。根据具体的需求和应用场景,选择合适的方法是高效处理数据的关键。
通过上述方法,可以有效地解决Python一次读取一行数据的问题。希望这些方法能够帮助你更好地理解和应用Python进行数据处理。
相关问答FAQs:
如何在Python中读取一行数据?
在Python中,可以使用内置的input()
函数从用户那里读取一行数据。调用该函数后,程序会暂停并等待用户输入,直到按下回车键为止。示例代码如下:
data = input("请输入一行数据:")
print("您输入的数据是:", data)
Python中如何处理文件中的一行数据?
如果需要从文件中读取一行数据,可以使用文件对象的readline()
方法。打开文件后,可以调用该方法来读取文件的当前行。以下是一个示例:
with open('data.txt', 'r') as file:
line = file.readline()
print("文件中的一行数据是:", line)
如何在Python中处理多行输入并提取特定行?
如果希望从用户输入中获取多行数据,可以使用循环来实现。在输入完成后,可以通过索引访问特定的行。以下是示例代码:
lines = []
while True:
line = input("输入一行数据(输入'结束'以停止):")
if line == "结束":
break
lines.append(line)
print("您输入的第二行数据是:", lines[1] if len(lines) > 1 else "没有第二行数据。")
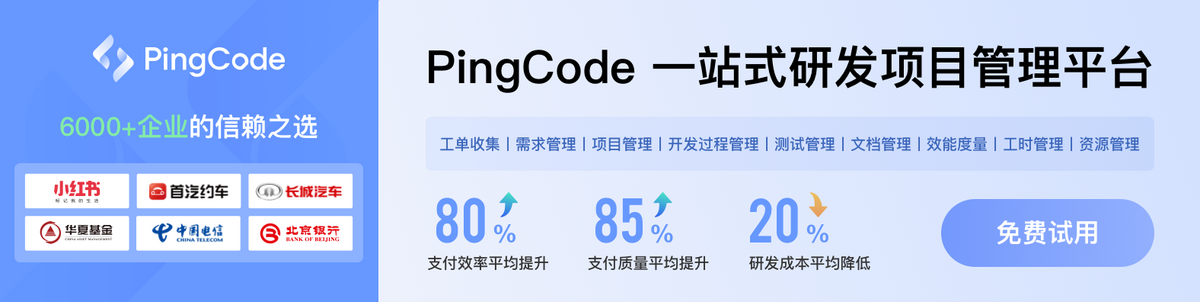