在Python中,获取字符串子串的起始位置可以使用字符串的内置方法find()
、index()
和正则表达式等。 find()
和index()
方法在功能上非常相似,但在处理子串未找到的情况时有所不同。find()
方法在子串未找到时会返回-1,而index()
方法则会抛出一个ValueError异常。接下来,我们将详细讨论这两种方法的使用,并介绍一些实用的技巧和注意事项。
一、使用find()方法
find()
方法是最常用的查找子串位置的方法之一。它返回子串在字符串中第一次出现的位置,如果未找到子串,则返回-1。
string = "Hello, welcome to the world of Python"
substring = "welcome"
position = string.find(substring)
print("子串的起始位置是:", position)
1.1 find()
方法的基本使用
find()
方法有三个参数:子串、开始位置和结束位置。默认情况下,它会从字符串的起始位置开始查找,直到字符串的结尾。
string = "Hello, welcome to the world of Python"
substring = "welcome"
从字符串的第7个字符到结尾查找
position = string.find(substring, 7)
print("子串的起始位置是:", position)
二、使用index()方法
index()
方法与find()
方法类似,但在子串未找到时会抛出一个ValueError异常,而不是返回-1。
string = "Hello, welcome to the world of Python"
substring = "welcome"
try:
position = string.index(substring)
print("子串的起始位置是:", position)
except ValueError:
print("未找到子串")
2.1 index()
方法的基本使用
与find()
方法类似,index()
方法也可以接受开始位置和结束位置的参数。
string = "Hello, welcome to the world of Python"
substring = "welcome"
从字符串的第7个字符到结尾查找
try:
position = string.index(substring, 7)
print("子串的起始位置是:", position)
except ValueError:
print("未找到子串")
三、使用正则表达式
正则表达式是处理字符串的强大工具。在Python中,可以使用re
模块来查找子串的位置。
import re
string = "Hello, welcome to the world of Python"
substring = "welcome"
match = re.search(substring, string)
if match:
print("子串的起始位置是:", match.start())
else:
print("未找到子串")
3.1 re.search()
方法的基本使用
re.search()
方法返回一个匹配对象,如果未找到子串,则返回None
。
import re
string = "Hello, welcome to the world of Python"
substring = "welcome"
match = re.search(substring, string)
if match:
print("子串的起始位置是:", match.start())
else:
print("未找到子串")
四、总结
在Python中,获取字符串子串的起始位置有多种方法,如find()
、index()
和正则表达式。find()
方法在子串未找到时返回-1、index()
方法在子串未找到时抛出ValueError异常、正则表达式提供了更强大的匹配功能。 通过选择合适的方法,可以更高效地处理字符串操作。
相关问答FAQs:
如何在Python中查找子串的起始位置?
在Python中,可以使用字符串的find()
和index()
方法来获取子串的起始位置。find()
方法返回子串首次出现的位置,如果未找到,则返回-1;而index()
方法在未找到子串时会引发一个异常。示例代码如下:
string = "Hello, welcome to the world of Python"
substring = "welcome"
position = string.find(substring) # 使用find方法
print(position) # 输出: 7
是否可以指定搜索的起始和结束位置?
是的,find()
和index()
方法允许你指定搜索的起始和结束位置。你只需在方法中添加相应的参数即可。示例:
position = string.find(substring, 5, 15) # 在索引5到15之间查找
print(position) # 输出: 7
如何处理多个相同子串的情况?
如果字符串中存在多个相同的子串,可以使用循环结合find()
方法来获取所有匹配的起始位置。示例代码如下:
string = "Python is great. Python is powerful."
substring = "Python"
start = 0
positions = []
while start < len(string):
start = string.find(substring, start)
if start == -1:
break
positions.append(start)
start += len(substring) # 更新起始位置,避免无限循环
print(positions) # 输出: [0, 20]
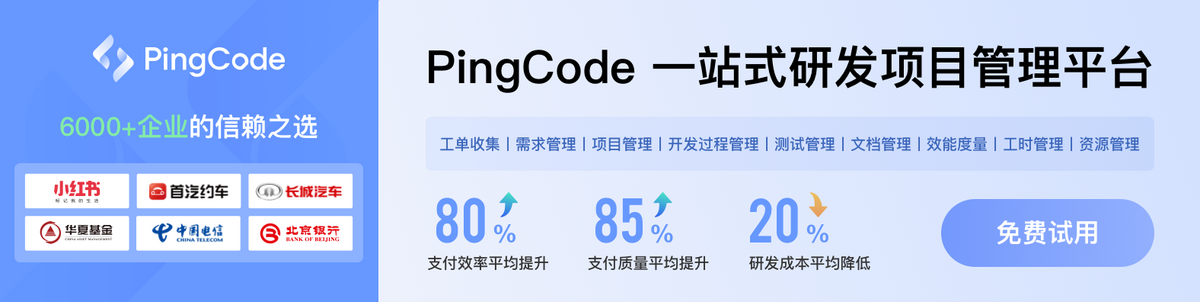