在Python中,将客户的数据一直保存的核心方法包括:使用文件系统、数据库、云存储、缓存。
其中,使用数据库是一种常见且有效的方法,能够保证数据的持久化、安全性和可扩展性。数据库如SQLite、MySQL、PostgreSQL等,提供了丰富的数据管理功能,适用于不同规模的应用。通过SQLAlchemy、Django ORM等ORM工具,可以简化数据库操作,使得开发过程更加便捷。
一、文件系统
使用文件系统是保存数据的基本方法之一。常见的文件格式包括文本文件、CSV文件、JSON文件等。
1.1 文本文件
文本文件适合保存简单的字符串数据。可以使用Python的内置函数open
进行读写操作。
# 写入数据
with open('customer_data.txt', 'w') as file:
file.write('Customer Name: John Doe\n')
file.write('Customer Email: john.doe@example.com\n')
读取数据
with open('customer_data.txt', 'r') as file:
data = file.read()
print(data)
1.2 CSV文件
CSV文件适合保存结构化的表格数据,可以使用csv
模块进行操作。
import csv
写入数据
with open('customer_data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Email'])
writer.writerow(['John Doe', 'john.doe@example.com'])
读取数据
with open('customer_data.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
1.3 JSON文件
JSON文件适合保存复杂的嵌套数据结构,可以使用json
模块进行操作。
import json
写入数据
customer_data = {
'name': 'John Doe',
'email': 'john.doe@example.com'
}
with open('customer_data.json', 'w') as file:
json.dump(customer_data, file)
读取数据
with open('customer_data.json', 'r') as file:
data = json.load(file)
print(data)
二、数据库
使用数据库可以更高效地管理和查询数据。常见的数据库包括SQLite、MySQL、PostgreSQL等。
2.1 SQLite
SQLite是一个轻量级的嵌入式数据库,适合小型应用。可以使用Python的sqlite3
模块进行操作。
import sqlite3
连接到数据库
conn = sqlite3.connect('customer_data.db')
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS customers (
id INTEGER PRIMARY KEY,
name TEXT,
email TEXT
)
''')
插入数据
cursor.execute('''
INSERT INTO customers (name, email)
VALUES ('John Doe', 'john.doe@example.com')
''')
查询数据
cursor.execute('SELECT * FROM customers')
rows = cursor.fetchall()
for row in rows:
print(row)
关闭连接
conn.commit()
conn.close()
2.2 MySQL
MySQL适合中大型应用,需要先安装MySQL数据库和mysql-connector-python
模块。
import mysql.connector
连接到数据库
conn = mysql.connector.connect(
host='localhost',
user='yourusername',
password='yourpassword',
database='yourdatabase'
)
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS customers (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255),
email VARCHAR(255)
)
''')
插入数据
cursor.execute('''
INSERT INTO customers (name, email)
VALUES ('John Doe', 'john.doe@example.com')
''')
查询数据
cursor.execute('SELECT * FROM customers')
rows = cursor.fetchall()
for row in rows:
print(row)
关闭连接
conn.commit()
conn.close()
2.3 PostgreSQL
PostgreSQL是一个功能强大的开源数据库,适合大规模应用。需要先安装PostgreSQL数据库和psycopg2
模块。
import psycopg2
连接到数据库
conn = psycopg2.connect(
host='localhost',
user='yourusername',
password='yourpassword',
dbname='yourdatabase'
)
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS customers (
id SERIAL PRIMARY KEY,
name VARCHAR(255),
email VARCHAR(255)
)
''')
插入数据
cursor.execute('''
INSERT INTO customers (name, email)
VALUES ('John Doe', 'john.doe@example.com')
''')
查询数据
cursor.execute('SELECT * FROM customers')
rows = cursor.fetchall()
for row in rows:
print(row)
关闭连接
conn.commit()
conn.close()
三、云存储
云存储适合需要高可用性和可扩展性的应用,可以使用AWS S3、Google Cloud Storage、Azure Blob Storage等。
3.1 AWS S3
需要先安装boto3
模块,并配置AWS凭证。
import boto3
创建S3客户端
s3 = boto3.client('s3')
上传数据
s3.put_object(Bucket='your-bucket', Key='customer_data.txt', Body='Customer Name: John Doe\nCustomer Email: john.doe@example.com')
下载数据
response = s3.get_object(Bucket='your-bucket', Key='customer_data.txt')
data = response['Body'].read().decode('utf-8')
print(data)
3.2 Google Cloud Storage
需要先安装google-cloud-storage
模块,并配置GCP凭证。
from google.cloud import storage
创建存储客户端
client = storage.Client()
bucket = client.bucket('your-bucket')
上传数据
blob = bucket.blob('customer_data.txt')
blob.upload_from_string('Customer Name: John Doe\nCustomer Email: john.doe@example.com')
下载数据
data = blob.download_as_string().decode('utf-8')
print(data)
3.3 Azure Blob Storage
需要先安装azure-storage-blob
模块,并配置Azure凭证。
from azure.storage.blob import BlobServiceClient
创建Blob服务客户端
blob_service_client = BlobServiceClient.from_connection_string('your_connection_string')
container_client = blob_service_client.get_container_client('your-container')
上传数据
blob_client = container_client.get_blob_client('customer_data.txt')
blob_client.upload_blob('Customer Name: John Doe\nCustomer Email: john.doe@example.com', overwrite=True)
下载数据
data = blob_client.download_blob().readall().decode('utf-8')
print(data)
四、缓存
缓存适合需要快速访问的数据,可以使用Redis、Memcached等。
4.1 Redis
需要先安装Redis数据库和redis-py
模块。
import redis
连接到Redis
r = redis.Redis(host='localhost', port=6379, db=0)
保存数据
r.set('customer_name', 'John Doe')
r.set('customer_email', 'john.doe@example.com')
获取数据
name = r.get('customer_name').decode('utf-8')
email = r.get('customer_email').decode('utf-8')
print(f'Customer Name: {name}, Customer Email: {email}')
4.2 Memcached
需要先安装Memcached数据库和pymemcache
模块。
from pymemcache.client import base
连接到Memcached
client = base.Client(('localhost', 11211))
保存数据
client.set('customer_name', 'John Doe')
client.set('customer_email', 'john.doe@example.com')
获取数据
name = client.get('customer_name').decode('utf-8')
email = client.get('customer_email').decode('utf-8')
print(f'Customer Name: {name}, Customer Email: {email}')
总之,选择合适的数据存储方法取决于应用的需求和规模。对于简单的应用,文件系统可能足够;对于复杂的应用,数据库或云存储是更好的选择;而对于需要快速访问的数据,缓存是理想的选择。在实际开发中,通常会结合使用多种方法,以满足不同的需求。
相关问答FAQs:
如何在Python中选择合适的数据库来保存客户数据?
在Python中,有多种数据库可供选择,如SQLite、MySQL、PostgreSQL等。SQLite适合小型项目,易于设置和使用,而MySQL和PostgreSQL更适合处理大型数据集和复杂查询。根据项目需求和规模,可以选择合适的数据库。通常,使用ORM(对象关系映射)库如SQLAlchemy,可以简化数据库操作,使得数据的存储和检索更加高效和方便。
如何确保客户数据的安全性和隐私保护?
保护客户数据安全至关重要。可以采取多种措施来确保数据的安全性,如加密存储、使用安全的网络传输协议(如HTTPS)、定期备份数据等。此外,遵循GDPR等相关法律法规,确保客户的隐私得到尊重和保护。实施访问控制机制,确保只有授权人员可以访问敏感数据,也是必要的步骤。
如何实现客户数据的自动备份和恢复?
实现自动备份可以通过编写Python脚本来定期导出数据库数据,存储到安全的云存储或本地备份设备。可以使用定时任务工具,如cron(在Linux系统中)或Windows任务计划程序,来安排备份的执行时间。恢复过程则需要确保备份文件的完整性,通常可以通过编写恢复脚本来实现数据的快速恢复,确保在数据丢失的情况下,业务能够迅速恢复正常运作。
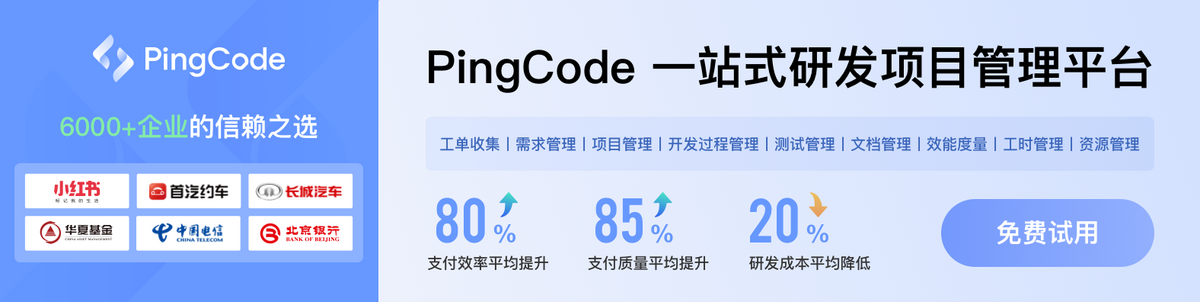