Python读取txt文件中的某一行的常用方法有几种,包括使用文件对象的方法、行迭代和内置函数等。以下是几种常见的方法:使用文件对象的readlines()
方法、通过行迭代器逐行读取、使用linecache
模块。 其中,推荐使用linecache
模块,因为它提供了一种简单、快捷的方法来读取特定行,且性能较优。
一、使用文件对象的readlines()
方法
使用文件对象的readlines()
方法可以将文件的所有行读取到一个列表中,然后通过索引访问某一行。以下是具体步骤:
- 打开文件:使用Python的内置函数
open()
来打开文件。 - 读取所有行:使用
readlines()
方法将文件的所有行读取到一个列表中。 - 访问特定行:通过列表索引访问特定行。
def read_specific_line(file_path, line_number):
with open(file_path, 'r', encoding='utf-8') as file:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1].strip()
else:
raise IndexError("Line number out of range")
file_path = 'example.txt'
line_number = 3
try:
print(read_specific_line(file_path, line_number))
except IndexError as e:
print(e)
二、通过行迭代器逐行读取
如果文件较大,直接读取所有行可能会占用大量内存。此时,可以逐行读取文件,并在达到所需行时停止。以下是具体步骤:
- 打开文件:使用Python的内置函数
open()
来打开文件。 - 逐行读取:使用
for
循环逐行读取文件内容。 - 判断行号:在循环中判断当前行号是否为所需行号,如果是,则返回该行内容。
def read_specific_line(file_path, line_number):
with open(file_path, 'r', encoding='utf-8') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
raise IndexError("Line number out of range")
file_path = 'example.txt'
line_number = 3
try:
print(read_specific_line(file_path, line_number))
except IndexError as e:
print(e)
三、使用linecache
模块
linecache
模块专门用于从文本文件中读取特定行。它的优势在于读取速度快,并且可以缓存文件内容,适用于多次读取同一文件的情况。以下是具体步骤:
- 导入模块:导入Python标准库中的
linecache
模块。 - 读取特定行:使用
linecache.getline()
方法读取特定行。
import linecache
def read_specific_line(file_path, line_number):
line = linecache.getline(file_path, line_number).strip()
if line:
return line
else:
raise IndexError("Line number out of range")
file_path = 'example.txt'
line_number = 3
try:
print(read_specific_line(file_path, line_number))
except IndexError as e:
print(e)
四、性能比较与最佳实践
对于小文件,使用readlines()
方法较为简单直观,但对于大文件可能会占用较多内存。逐行读取方法适用于内存受限的场景,但实现稍显复杂。linecache
模块在性能和易用性方面兼顾,适用于多次读取同一文件的情况。
1. 内存占用
readlines()
方法将文件的所有行一次性读取到内存中,适用于小文件。- 逐行读取方法在读取过程中只保留当前行,适用于大文件。
2. 性能
linecache
模块提供了较高的读取速度,并且可以缓存文件内容,适用于多次读取同一文件的场景。
3. 可读性和维护性
readlines()
方法代码简洁直观。- 逐行读取方法代码稍显复杂,但适用于大文件。
linecache
模块使用简便,适用于多次读取同一文件。
五、应用场景
1. 日志分析
在日志分析中,通常需要读取特定行来定位问题。此时,可以根据日志文件大小选择合适的方法:
- 小日志文件:使用
readlines()
方法快速读取。 - 大日志文件:使用逐行读取方法或
linecache
模块。
2. 数据处理
在数据处理过程中,可能需要读取特定行的数据进行分析。例如,在处理大数据文件时,可以使用逐行读取方法或linecache
模块来提高效率。
3. 教学案例
在教学案例中,通常需要读取特定行进行演示。例如,在编写Python教学资料时,可以使用linecache
模块快速读取特定行并展示代码示例。
六、代码示例与完整实现
以下是一个完整的代码示例,展示了如何使用上述三种方法读取txt文件中的某一行:
import linecache
def read_specific_line_readlines(file_path, line_number):
with open(file_path, 'r', encoding='utf-8') as file:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1].strip()
else:
raise IndexError("Line number out of range")
def read_specific_line_iterator(file_path, line_number):
with open(file_path, 'r', encoding='utf-8') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
raise IndexError("Line number out of range")
def read_specific_line_linecache(file_path, line_number):
line = linecache.getline(file_path, line_number).strip()
if line:
return line
else:
raise IndexError("Line number out of range")
file_path = 'example.txt'
line_number = 3
try:
print("Using readlines():", read_specific_line_readlines(file_path, line_number))
print("Using iterator:", read_specific_line_iterator(file_path, line_number))
print("Using linecache:", read_specific_line_linecache(file_path, line_number))
except IndexError as e:
print(e)
通过以上方法,可以有效读取txt文件中的某一行,并根据具体需求选择合适的方法。
相关问答FAQs:
如何在Python中读取特定行的文本文件?
在Python中,读取特定行的文本文件可以通过多种方法实现。一种常见的方法是使用with open()
语句打开文件,然后使用readlines()
方法将文件的所有行读取为列表,接着可以通过索引访问特定的行。例如,lines = file.readlines()
读取所有行,specific_line = lines[line_number]
获取所需行。确保行号从0开始。
读取文件时如何处理异常情况?
在读取文本文件时,可能会遇到一些异常情况,比如文件不存在或权限问题。使用try...except
语句可以有效处理这些问题。例如,可以捕获FileNotFoundError
并在出错时给出友好的提示,确保程序不会崩溃,同时用户能明白发生了什么。
有没有方法可以在不加载整个文件的情况下读取特定行?
确实可以通过逐行读取的方式来避免加载整个文件。这种方法适合处理大型文件,可以使用for
循环逐行读取文件,直到找到目标行。例如,可以使用enumerate()
函数来跟踪当前行号,并在达到所需行时停止读取。这样的方法不仅节省内存,还提高了效率。
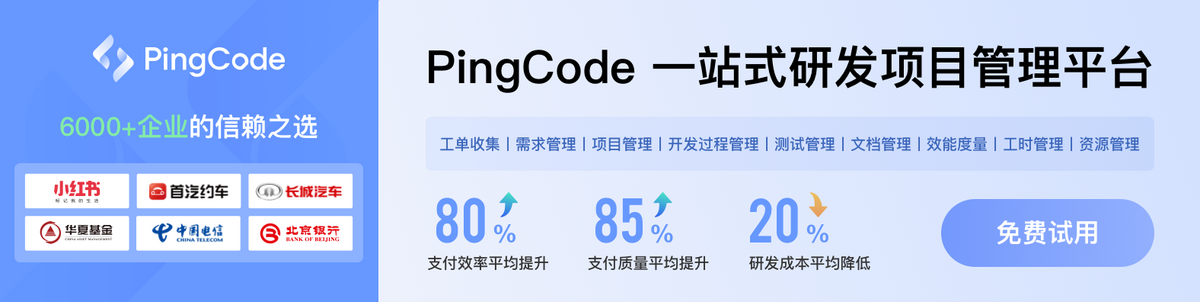