在Python中计算三角形面积的方法有多种,包括使用海伦公式、基于顶点坐标的面积公式和利用底边与高的公式。 常见的方法包括:海伦公式、顶点坐标法、底边与高的公式。接下来,我们将详细介绍这些方法。
一、海伦公式
海伦公式是计算三角形面积的一种常用方法,特别适用于已知三条边长的情况。公式如下:
[ \text{面积} = \sqrt{s(s-a)(s-b)(s-c)} ]
其中,( s ) 是三角形的半周长,公式为 ( s = \frac{a + b + c}{2} ),( a )、( b )、( c ) 是三角形的三条边长。
实现步骤:
- 计算三角形的半周长 ( s )。
- 使用海伦公式计算面积。
import math
def triangle_area_heron(a, b, c):
# Step 1: Calculate the semi-perimeter
s = (a + b + c) / 2
# Step 2: Calculate the area using Heron's formula
area = math.sqrt(s * (s - a) * (s - b) * (s - c))
return area
Example usage:
a, b, c = 3, 4, 5
print(f"Area of the triangle using Heron's formula: {triangle_area_heron(a, b, c)}")
二、顶点坐标法
如果已知三角形的三个顶点坐标,可以使用以下公式计算面积:
[ \text{面积} = \frac{1}{2} \left| x_1(y_2 – y_3) + x_2(y_3 – y_1) + x_3(y_1 – y_2) \right| ]
其中,( (x_1, y_1) )、( (x_2, y_2) )、( (x_3, y_3) ) 是三角形的三个顶点坐标。
实现步骤:
- 将顶点坐标带入公式。
- 计算并取绝对值,再乘以 ( \frac{1}{2} )。
def triangle_area_coordinates(x1, y1, x2, y2, x3, y3):
# Calculate the area using the vertex coordinates formula
area = abs(x1*(y2 - y3) + x2*(y3 - y1) + x3*(y1 - y2)) / 2
return area
Example usage:
x1, y1 = 0, 0
x2, y2 = 4, 0
x3, y3 = 0, 3
print(f"Area of the triangle using vertex coordinates: {triangle_area_coordinates(x1, y1, x2, y2, x3, y3)}")
三、底边与高
当已知三角形的底边长度和对应的高时,可以直接使用以下公式计算面积:
[ \text{面积} = \frac{1}{2} \times \text{底边} \times \text{高} ]
实现步骤:
- 获取底边长度和高。
- 代入公式计算面积。
def triangle_area_base_height(base, height):
# Calculate the area using base and height
area = 0.5 * base * height
return area
Example usage:
base, height = 5, 6
print(f"Area of the triangle using base and height: {triangle_area_base_height(base, height)}")
四、应用场景和选择方法
1、选择合适的方法
在实际应用中,选择计算三角形面积的方法取决于已知的参数:
- 已知三边长度:使用海伦公式。
- 已知顶点坐标:使用顶点坐标法。
- 已知底边和高:使用底边与高的公式。
2、性能和精度
在高精度和大规模计算场景中,选择合适的算法和数据类型(如使用 decimal.Decimal
而不是 float
)是必要的。
from decimal import Decimal, getcontext
Set precision
getcontext().prec = 30
def triangle_area_heron_decimal(a, b, c):
# Use Decimal for higher precision
a, b, c = Decimal(a), Decimal(b), Decimal(c)
s = (a + b + c) / 2
area = (s * (s - a) * (s - b) * (s - c)).sqrt()
return area
Example usage:
a, b, c = 3, 4, 5
print(f"Area of the triangle using Heron's formula with Decimal: {triangle_area_heron_decimal(a, b, c)}")
五、扩展应用
1、结合数据处理与可视化
在数据科学和工程领域,常常需要结合数据处理与可视化工具对三角形进行分析和展示。例如,使用 matplotlib
库绘制三角形并标注其面积。
import matplotlib.pyplot as plt
import numpy as np
def plot_triangle(x1, y1, x2, y2, x3, y3):
# Plot the triangle
plt.figure()
plt.plot([x1, x2], [y1, y2], 'b-')
plt.plot([x2, x3], [y2, y3], 'b-')
plt.plot([x3, x1], [y3, y1], 'b-')
# Annotate vertices
plt.text(x1, y1, f'({x1}, {y1})', fontsize=12, ha='right')
plt.text(x2, y2, f'({x2}, {y2})', fontsize=12, ha='right')
plt.text(x3, y3, f'({x3}, {y3})', fontsize=12, ha='right')
# Calculate and annotate area
area = triangle_area_coordinates(x1, y1, x2, y2, x3, y3)
plt.title(f'Triangle with Area: {area}')
plt.show()
Example usage:
x1, y1 = 0, 0
x2, y2 = 4, 0
x3, y3 = 0, 3
plot_triangle(x1, y1, x2, y2, x3, y3)
2、与几何算法结合
在计算几何领域,计算三角形面积是许多算法的基础,如多边形面积计算、三角剖分等。
def polygon_area(vertices):
# Calculate the area of a polygon using the shoelace formula
n = len(vertices)
area = 0.0
for i in range(n):
x1, y1 = vertices[i]
x2, y2 = vertices[(i + 1) % n]
area += x1 * y2 - x2 * y1
return abs(area) / 2
Example usage:
polygon_vertices = [(0, 0), (4, 0), (4, 3), (0, 3)]
print(f"Area of the polygon: {polygon_area(polygon_vertices)}")
六、总结
在Python中计算三角形面积的方法多种多样,选择合适的方法取决于已知的参数和具体应用场景。无论是使用海伦公式、顶点坐标法还是底边与高的公式,都可以帮助我们准确地计算三角形面积。 通过结合数据处理与可视化工具,我们还可以更好地理解和展示几何特性。在实际应用中,考虑精度和性能也是至关重要的。
相关问答FAQs:
如何在Python中计算三角形面积?
在Python中,可以使用不同的方法来计算三角形的面积。最常见的公式是使用底边和高的公式:面积 = 0.5 * 底 * 高。你只需定义底边和高的值,然后用简单的数学运算来计算面积。例如:
底 = 5
高 = 10
面积 = 0.5 * 底 * 高
print(面积)
此外,你也可以使用海伦公式,当已知三角形的三边长度时,面积可以通过以下步骤计算:先计算半周长,然后利用半周长和三边长度来计算面积。
有哪些不同的方法可以计算三角形的面积?
除了底边和高的公式,计算三角形面积的其他方法还包括使用海伦公式、坐标法以及使用三角函数。海伦公式适用于已知三边的情况,而坐标法则适用于已知三角形三个顶点的坐标。具体来说,海伦公式为:首先计算半周长s = (a + b + c) / 2,然后面积 = √(s * (s – a) * (s – b) * (s – c))。
如何处理输入数据以计算三角形面积?
在编写计算三角形面积的程序时,处理用户输入数据非常重要。可以使用input()
函数获取用户输入的底边和高,或者三边长度。在获取输入后,确保将字符串转换为浮点数,以便进行数学计算。同时,可以添加异常处理来确保用户输入的是有效的数值,避免程序因输入错误而崩溃。
try:
底 = float(input("请输入三角形的底边长度: "))
高 = float(input("请输入三角形的高: "))
面积 = 0.5 * 底 * 高
print("三角形的面积是:", 面积)
except ValueError:
print("请输入有效的数字")
在Python中计算三角形面积时是否需要考虑精度问题?
在计算三角形面积时,通常不需要过于担心精度问题,因为使用浮点数进行基本的数学运算已经能够满足大多数需求。然而,如果要进行高精度计算或处理非常小或非常大的数值,可以考虑使用decimal
模块,它提供了更高的精度。使用decimal.Decimal
可以有效地避免浮点数运算中的精度损失。
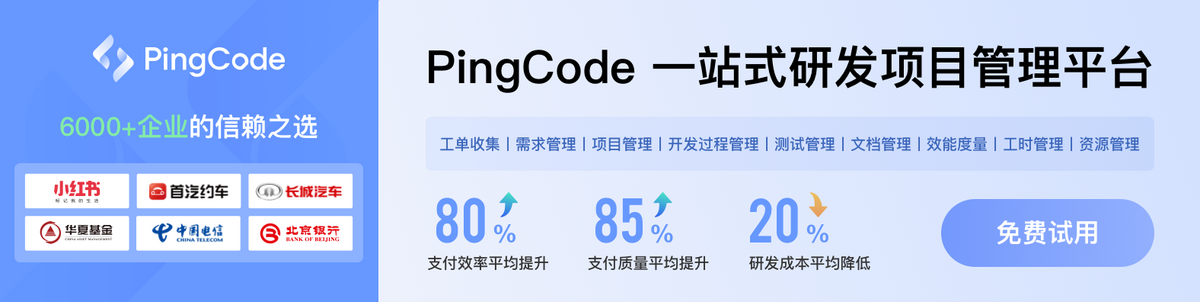