在Python3中使用三角函数非常简单,主要依赖于math
模块。 math
模块提供了一系列的数学函数,包括三角函数,如正弦、余弦和正切函数等。为了使用这些函数,你需要先导入math
模块。你可以通过以下步骤轻松地在Python3中使用三角函数:导入math
模块、使用sin
、cos
、tan
函数、使用弧度和角度转换函数、处理其他相关三角函数。 下面将详细介绍如何使用这些函数。
一、导入math
模块
在Python3中,所有的数学函数,包括三角函数,都在math
模块中定义。要使用这些函数,首先需要导入这个模块。可以通过以下方式导入:
import math
导入math
模块后,你就可以使用其中的函数了。
二、使用sin
、cos
、tan
函数
math
模块提供了计算正弦、余弦和正切值的函数,分别是math.sin
、math.cos
和math.tan
。这些函数的参数和返回值都是以弧度为单位的。
1、计算正弦值
math.sin(x)
函数用于计算x的正弦值,其中x是以弧度为单位的角度。例如:
import math
x = math.radians(30) # 将30度转换为弧度
sin_x = math.sin(x)
print(f"The sine of 30 degrees is: {sin_x}")
2、计算余弦值
math.cos(x)
函数用于计算x的余弦值。例如:
import math
x = math.radians(60) # 将60度转换为弧度
cos_x = math.cos(x)
print(f"The cosine of 60 degrees is: {cos_x}")
3、计算正切值
math.tan(x)
函数用于计算x的正切值。例如:
import math
x = math.radians(45) # 将45度转换为弧度
tan_x = math.tan(x)
print(f"The tangent of 45 degrees is: {tan_x}")
三、使用弧度和角度转换函数
在处理三角函数时,经常需要在角度和弧度之间进行转换。math
模块提供了两个方便的函数:math.radians
和math.degrees
。
1、将角度转换为弧度
math.radians(x)
函数用于将x度的角度转换为弧度。例如:
import math
degrees = 90
radians = math.radians(degrees)
print(f"{degrees} degrees is equal to {radians} radians")
2、将弧度转换为角度
math.degrees(x)
函数用于将x弧度的角度转换为度。例如:
import math
radians = math.pi / 2
degrees = math.degrees(radians)
print(f"{radians} radians is equal to {degrees} degrees")
四、处理其他相关三角函数
除了基本的正弦、余弦和正切函数外,math
模块还提供了一些其他的三角函数,如反三角函数和双曲线函数。
1、反三角函数
反三角函数用于计算一个数的反正弦、反余弦和反正切值,分别是math.asin
、math.acos
和math.atan
。
反正弦
import math
x = 0.5
asin_x = math.asin(x)
print(f"The arcsine of {x} is: {asin_x} radians")
反余弦
import math
x = 0.5
acos_x = math.acos(x)
print(f"The arccosine of {x} is: {acos_x} radians")
反正切
import math
x = 1
atan_x = math.atan(x)
print(f"The arctangent of {x} is: {atan_x} radians")
2、双曲线函数
双曲线函数包括双曲正弦、双曲余弦和双曲正切,分别是math.sinh
、math.cosh
和math.tanh
。
双曲正弦
import math
x = 1
sinh_x = math.sinh(x)
print(f"The hyperbolic sine of {x} is: {sinh_x}")
双曲余弦
import math
x = 1
cosh_x = math.cosh(x)
print(f"The hyperbolic cosine of {x} is: {cosh_x}")
双曲正切
import math
x = 1
tanh_x = math.tanh(x)
print(f"The hyperbolic tangent of {x} is: {tanh_x}")
五、应用实例
在实际应用中,三角函数有很多用途,如在物理学、工程学和计算机图形学中。下面是几个具体的应用实例。
1、计算斜边长度
在直角三角形中,可以使用三角函数计算斜边的长度。例如,已知一个直角三角形的一个角度和一个邻边的长度,计算斜边的长度:
import math
angle_degrees = 30
adjacent_length = 5
angle_radians = math.radians(angle_degrees)
hypotenuse_length = adjacent_length / math.cos(angle_radians)
print(f"The length of the hypotenuse is: {hypotenuse_length}")
2、绘制正弦曲线
正弦曲线在信号处理和图形学中有广泛的应用。以下示例展示了如何使用matplotlib
库绘制正弦曲线:
import math
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 2 * math.pi, 100)
y = np.sin(x)
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('sin(x)')
plt.title('Sine Wave')
plt.show()
3、计算倾斜角度
在物理学中,倾斜角度计算是一个常见问题。例如,已知斜面的高度和底部长度,计算倾斜角度:
import math
height = 3
base_length = 4
angle_radians = math.atan(height / base_length)
angle_degrees = math.degrees(angle_radians)
print(f"The angle of inclination is: {angle_degrees} degrees")
六、注意事项
在使用三角函数时,有几个注意事项需要牢记:
1、单位问题
三角函数的参数和返回值通常以弧度为单位,在使用时需要注意单位的转换。如果你使用的是角度,请确保在使用前转换为弧度。
2、数值精度
由于计算机在表示浮点数时有精度限制,三角函数的计算结果可能会有微小的误差。在需要高精度的场合,可能需要特别处理。
3、定义域和值域
了解每个三角函数的定义域和值域非常重要。例如,正弦和余弦函数的值域是[-1, 1],而正切函数的值域是所有实数。
七、总结
通过math
模块,Python3提供了丰富的三角函数支持,能够满足大多数数学计算需求。导入math
模块、使用sin
、cos
、tan
函数、使用弧度和角度转换函数、处理其他相关三角函数,这些步骤使得在Python中处理三角函数变得非常简单。无论是在学术研究、工程应用还是日常编程中,掌握这些函数都能极大地提高你的工作效率。
相关问答FAQs:
在Python3中,如何导入并使用三角函数库?
在Python3中,可以使用内置的math
模块来访问三角函数。要使用这些函数,首先需要导入该模块。可以通过以下代码导入:
import math
导入后,您可以使用math.sin()
、math.cos()
和math.tan()
等函数来计算三角函数。例如,计算30度的正弦值可以这样写:
import math
result = math.sin(math.radians(30)) # 将角度转换为弧度
Python3中三角函数的输入参数要求是什么?
在Python3的math
模块中,所有的三角函数都以弧度为单位进行计算。这意味着,如果您有角度值,需要使用math.radians()
函数将角度转换为弧度。例如,45度转为弧度可以通过以下方式实现:
angle_in_radians = math.radians(45)
转换后,您就可以将该值传递给三角函数来获取结果。
在Python3中可以使用哪些常用的三角函数?
Python3的math
模块提供了多种常用的三角函数,包括:
math.sin(x)
:返回x的正弦值math.cos(x)
:返回x的余弦值math.tan(x)
:返回x的正切值
此外,模块还提供了反三角函数,如:math.asin(x)
:返回x的反正弦值math.acos(x)
:返回x的反余弦值math.atan(x)
:返回x的反正切值
这些函数可以帮助您在进行各种数学计算时轻松地处理三角关系。
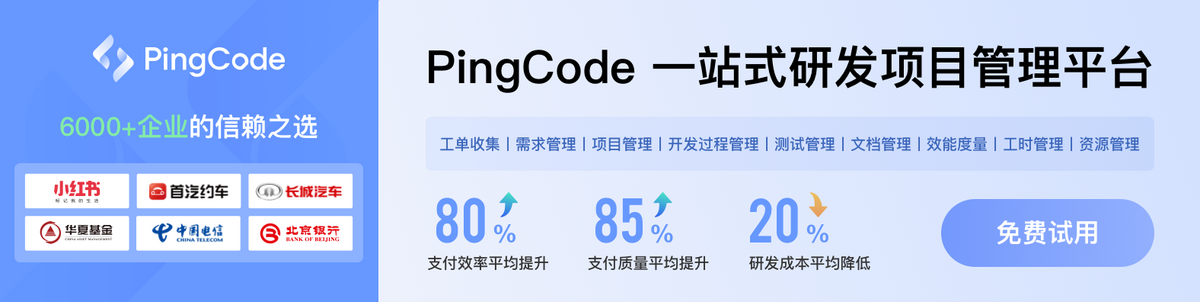