Python如何写一个简单计算器
在Python中写一个简单的计算器可以通过基本的编程概念和一些内置函数来实现。使用基本的数学运算符、通过函数分割不同操作、处理用户输入和输出,这些都是实现简单计算器的关键步骤。本文将详细解释如何在Python中编写一个简单的计算器,并逐步介绍每个步骤。
一、使用基本的数学运算符
Python提供了许多内置的数学运算符,如加法(+)、减法(-)、乘法(*)、除法(/)等。通过这些运算符,我们可以实现基本的数学运算。
1. 加法和减法
加法和减法是最基本的数学运算,也是计算器的基础功能。我们可以通过定义函数来实现这些运算。
def add(x, y):
return x + y
def subtract(x, y):
return x - y
2. 乘法和除法
乘法和除法是稍微复杂一些的运算,但在Python中实现起来也很简单。
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
raise ValueError("Cannot divide by zero!")
return x / y
二、通过函数分割不同操作
为了使代码更清晰和模块化,我们可以将每个操作(加法、减法、乘法、除法)放在单独的函数中。这样不仅使代码更易于维护,还提高了代码的可读性。
1. 定义函数
我们已经定义了基本的数学运算函数,现在可以将这些函数整合到一个主函数中。
def calculator():
operation = input("Enter operation (+, -, *, /): ")
if operation not in ('+', '-', '*', '/'):
raise ValueError("Invalid operation!")
x = float(input("Enter first number: "))
y = float(input("Enter second number: "))
if operation == '+':
print(f"The result is: {add(x, y)}")
elif operation == '-':
print(f"The result is: {subtract(x, y)}")
elif operation == '*':
print(f"The result is: {multiply(x, y)}")
elif operation == '/':
print(f"The result is: {divide(x, y)}")
三、处理用户输入和输出
用户输入和输出是计算器的重要部分。我们需要确保用户输入的数据类型正确,并且在操作完成后输出正确的结果。
1. 输入验证
在计算器中,我们需要确保用户输入的是有效的数字和操作符。可以使用异常处理机制来捕捉无效输入,并提示用户重新输入。
def get_number(prompt):
while True:
try:
value = float(input(prompt))
return value
except ValueError:
print("Invalid input. Please enter a number.")
2. 输出结果
将结果输出给用户也是计算器的重要功能。我们可以使用print函数来显示计算结果。
def print_result(result):
print(f"The result is: {result}")
四、综合实现
现在我们将所有部分整合起来,形成一个完整的简单计算器程序。
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
raise ValueError("Cannot divide by zero!")
return x / y
def get_number(prompt):
while True:
try:
value = float(input(prompt))
return value
except ValueError:
print("Invalid input. Please enter a number.")
def calculator():
operation = input("Enter operation (+, -, *, /): ")
if operation not in ('+', '-', '*', '/'):
raise ValueError("Invalid operation!")
x = get_number("Enter first number: ")
y = get_number("Enter second number: ")
if operation == '+':
result = add(x, y)
elif operation == '-':
result = subtract(x, y)
elif operation == '*':
result = multiply(x, y)
elif operation == '/':
result = divide(x, y)
print_result(result)
def print_result(result):
print(f"The result is: {result}")
if __name__ == "__main__":
while True:
try:
calculator()
another = input("Do you want to perform another calculation? (yes/no): ")
if another.lower() != 'yes':
break
except Exception as e:
print(e)
五、进一步扩展
虽然我们已经实现了一个基本的计算器,但我们还可以进一步扩展其功能。例如,可以加入幂运算、平方根计算、三角函数等高级数学运算。
1. 幂运算
我们可以使用Python的内置运算符来实现幂运算。
def power(x, y):
return x y
2. 平方根计算
可以使用math模块中的sqrt函数来实现平方根计算。
import math
def sqrt(x):
return math.sqrt(x)
3. 三角函数
同样,使用math模块中的sin、cos、tan等函数可以实现三角函数计算。
def sin(x):
return math.sin(x)
def cos(x):
return math.cos(x)
def tan(x):
return math.tan(x)
六、用户界面(CLI和GUI)
为了提高用户体验,我们可以为计算器添加一个简单的用户界面。可以选择使用命令行界面(CLI)或图形用户界面(GUI)。
1. 命令行界面(CLI)
通过一些第三方库,如click,可以更方便地创建命令行界面。
import click
@click.command()
@click.option('--operation', prompt='Operation (+, -, *, /, , sqrt, sin, cos, tan)',
help='The operation to perform.')
@click.option('--x', prompt='Enter first number', type=float, help='First number.')
@click.option('--y', prompt='Enter second number (if applicable)', type=float, help='Second number.', default=1.0)
def cli_calculator(operation, x, y):
if operation == '+':
result = add(x, y)
elif operation == '-':
result = subtract(x, y)
elif operation == '*':
result = multiply(x, y)
elif operation == '/':
result = divide(x, y)
elif operation == '':
result = power(x, y)
elif operation == 'sqrt':
result = sqrt(x)
elif operation == 'sin':
result = sin(x)
elif operation == 'cos':
result = cos(x)
elif operation == 'tan':
result = tan(x)
else:
raise ValueError("Invalid operation!")
print(f"The result is: {result}")
if __name__ == "__main__":
cli_calculator()
2. 图形用户界面(GUI)
可以使用tkinter库来创建一个简单的图形用户界面。
import tkinter as tk
from tkinter import messagebox
def on_calculate():
try:
x = float(entry_x.get())
y = float(entry_y.get())
operation = entry_operation.get()
if operation == '+':
result = add(x, y)
elif operation == '-':
result = subtract(x, y)
elif operation == '*':
result = multiply(x, y)
elif operation == '/':
result = divide(x, y)
elif operation == '':
result = power(x, y)
elif operation == 'sqrt':
result = sqrt(x)
elif operation == 'sin':
result = sin(x)
elif operation == 'cos':
result = cos(x)
elif operation == 'tan':
result = tan(x)
else:
raise ValueError("Invalid operation!")
messagebox.showinfo("Result", f"The result is: {result}")
except Exception as e:
messagebox.showerror("Error", str(e))
root = tk.Tk()
root.title("Simple Calculator")
tk.Label(root, text="First Number:").grid(row=0)
tk.Label(root, text="Second Number:").grid(row=1)
tk.Label(root, text="Operation (+, -, *, /, , sqrt, sin, cos, tan):").grid(row=2)
entry_x = tk.Entry(root)
entry_y = tk.Entry(root)
entry_operation = tk.Entry(root)
entry_x.grid(row=0, column=1)
entry_y.grid(row=1, column=1)
entry_operation.grid(row=2, column=1)
tk.Button(root, text='Calculate', command=on_calculate).grid(row=3, column=0, columnspan=2)
root.mainloop()
七、总结
通过这篇文章,我们详细介绍了如何在Python中编写一个简单的计算器,包括基本的数学运算、函数分割、用户输入和输出处理、进一步扩展功能以及用户界面的实现。使用基本的数学运算符、通过函数分割不同操作、处理用户输入和输出,这些都是实现简单计算器的关键步骤。希望本文能帮助你更好地理解和实现一个简单的Python计算器。
相关问答FAQs:
如何使用Python创建一个简单的加法计算器?
要创建一个简单的加法计算器,可以使用Python的input()函数获取用户输入,并将其转换为数字。示例代码如下:
def add(x, y):
return x + y
num1 = float(input("输入第一个数字: "))
num2 = float(input("输入第二个数字: "))
result = add(num1, num2)
print("结果是:", result)
这个代码片段将提示用户输入两个数字,并输出它们的和。
Python的简单计算器可以处理哪些基本运算?
一个简单的Python计算器可以处理加法、减法、乘法和除法等基本运算。可以通过定义不同的函数来实现这些运算,例如:
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y != 0:
return x / y
else:
return "错误:除数不能为零"
通过调用这些函数,可以轻松实现多种计算功能。
如何在Python中实现一个具有用户交互功能的计算器?
实现用户交互的计算器可以使用循环和条件语句来让用户选择操作类型。以下是一个简单示例:
while True:
print("选择运算: 1. 加法 2. 减法 3. 乘法 4. 除法 5. 退出")
choice = input("输入你的选择: ")
if choice in ['1', '2', '3', '4']:
num1 = float(input("输入第一个数字: "))
num2 = float(input("输入第二个数字: "))
if choice == '1':
print("结果是:", add(num1, num2))
elif choice == '2':
print("结果是:", subtract(num1, num2))
elif choice == '3':
print("结果是:", multiply(num1, num2))
elif choice == '4':
print("结果是:", divide(num1, num2))
elif choice == '5':
print("退出计算器")
break
else:
print("无效输入,请重试")
这样的实现使得用户能够多次进行计算,并选择退出。
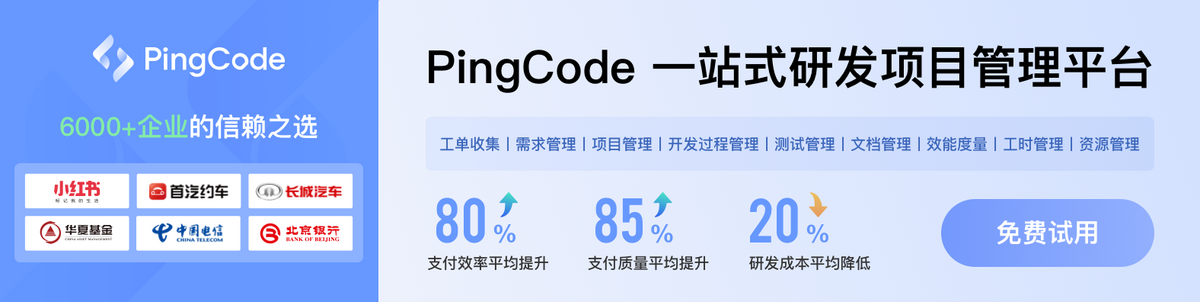