用Python画一朵玫瑰花可以通过使用图形库如Turtle、Matplotlib等来实现。 常用的库有Turtle、Matplotlib、PIL。其中,Turtle库是一种轻量级的图形绘制库,适合初学者;Matplotlib则是一个强大的绘图库,适合更复杂的图形绘制;PIL(Python Imaging Library)则适用于图像处理。本文将详细介绍如何使用这三种库来绘制玫瑰花,并提供相应的代码示例。
一、使用Turtle库绘制玫瑰花
Turtle库是Python标准库中一个简单易用的图形绘制库,非常适合初学者学习绘图。
1、安装Turtle库
Turtle库是Python的标准库,无需额外安装。只需确保你已安装Python环境。
2、绘制玫瑰花的代码实现
以下是使用Turtle库绘制玫瑰花的代码:
import turtle
import math
def draw_rose():
turtle.speed(0)
turtle.Screen().bgcolor("white")
turtle.color("red")
for i in range(300):
t = i / 30 * math.pi
x = 160 * math.sin(t) 3
y = 130 * math.cos(t) - 50 * math.cos(2 * t) - 20 * math.cos(3 * t) - 10 * math.cos(4 * t)
turtle.goto(x, y)
turtle.hideturtle()
turtle.done()
if __name__ == "__main__":
turtle.penup()
draw_rose()
3、代码解读
- turtle.speed(0):设置绘图速度,0为最快。
- turtle.Screen().bgcolor("white"):设置背景颜色为白色。
- turtle.color("red"):设置画笔颜色为红色。
- turtle.penup():抬起画笔,不绘制路径。
- turtle.goto(x, y):移动到指定的x, y坐标。
- turtle.hideturtle():隐藏箭头。
二、使用Matplotlib库绘制玫瑰花
Matplotlib是一个强大的绘图库,适合绘制更复杂的图形。
1、安装Matplotlib库
pip install matplotlib
2、绘制玫瑰花的代码实现
以下是使用Matplotlib绘制玫瑰花的代码:
import numpy as np
import matplotlib.pyplot as plt
theta = np.linspace(0, 2 * np.pi, 1000)
r = 5 * np.sin(4 * theta)
plt.figure(figsize=(6,6))
plt.polar(theta, r, color='red')
plt.title('Rose Plot')
plt.show()
3、代码解读
- np.linspace(0, 2 * np.pi, 1000):生成从0到2π的1000个等间距的点。
- r = 5 * np.sin(4 * theta):定义玫瑰曲线的极坐标方程。
- plt.polar(theta, r, color='red'):绘制极坐标图,颜色为红色。
三、使用PIL库绘制玫瑰花
PIL(Python Imaging Library)适用于图像处理,可以用来绘制复杂的图形。
1、安装PIL库
PIL库已经被Pillow取代,使用以下命令安装:
pip install pillow
2、绘制玫瑰花的代码实现
以下是使用Pillow绘制玫瑰花的代码:
from PIL import Image, ImageDraw
import math
def draw_rose():
image = Image.new("RGB", (400, 400), "white")
draw = ImageDraw.Draw(image)
for i in range(300):
t = i / 30 * math.pi
x = 200 + 160 * math.sin(t) 3
y = 200 - (130 * math.cos(t) - 50 * math.cos(2 * t) - 20 * math.cos(3 * t) - 10 * math.cos(4 * t))
draw.point((x, y), fill="red")
image.show()
if __name__ == "__main__":
draw_rose()
3、代码解读
- Image.new("RGB", (400, 400), "white"):创建一个400×400的白色背景图像。
- ImageDraw.Draw(image):创建一个绘图对象。
- draw.point((x, y), fill="red"):在指定坐标绘制一个红色点。
- image.show():显示绘制的图像。
四、总结
以上介绍了使用Python三种不同的库来绘制玫瑰花的方法,包括Turtle、Matplotlib和PIL。这些方法各有优劣,Turtle适合初学者,Matplotlib适合复杂图形,PIL适合图像处理。根据自己的需求选择适合的工具,可以更好地完成绘图任务。
希望这篇文章对你有所帮助,祝你在Python绘图的道路上越走越远!
相关问答FAQs:
如何使用Python绘制玫瑰花的基本步骤是什么?
绘制玫瑰花的基本步骤包括:安装必要的Python库(如matplotlib和numpy),然后使用极坐标方程定义玫瑰花的形状。通过设置适当的参数,可以控制花瓣的数量和形状。最终,利用绘图库将这些参数可视化,生成玫瑰花的图像。
有哪些Python库可以帮助我绘制玫瑰花?
常用的Python库包括matplotlib和numpy。matplotlib提供了强大的绘图功能,适合创建各种图形和数据可视化,而numpy则用于处理数学运算和生成数据点。此外,turtle库也可以用来绘制简单的图形,适合初学者。
绘制玫瑰花时,我需要注意哪些参数设置?
在绘制玫瑰花时,需要关注几个关键参数,包括花瓣的数量(k值)、花瓣的长度和颜色。k值决定了花瓣的数量和形状,通常在0到10之间选择。花瓣的长度和颜色则可以根据个人喜好进行调整,以达到理想的视觉效果。
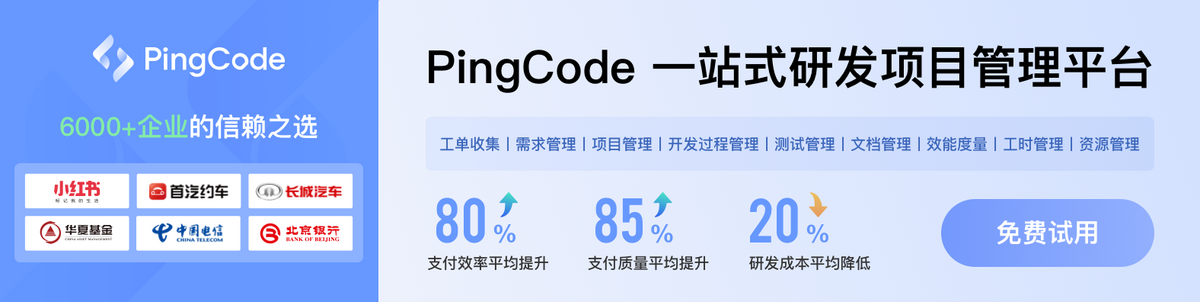