Python可以通过使用PIL(Pillow)库来将TIF文件导出为自定义名称、PIL库提供了方便的方法来处理图像文件、我们可以通过加载TIF文件并保存为新名称来实现这一点。以下是一个详细的步骤说明,展示了如何通过Python代码来完成这一任务。
要将TIF文件导出为自定义名称,首先需要安装PIL库。PIL(Python Imaging Library)是一个强大的图像处理库,Pillow是PIL的一个分支,具有更好的兼容性和更多的功能。以下是具体步骤:
一、安装Pillow库
Pillow库可以通过pip命令安装:
pip install pillow
二、加载TIF文件
首先,我们需要加载TIF文件。可以使用Pillow库中的Image
模块来完成这一操作。
from PIL import Image
加载TIF文件
image = Image.open("path_to_your_file.tif")
三、导出为自定义名称
接下来,我们可以使用save
方法将图像保存为自定义名称。
# 导出为自定义名称
custom_name = "custom_name.tif"
image.save(custom_name)
四、完整示例代码
以下是一个完整的示例代码,展示了如何将TIF文件加载并导出为自定义名称:
from PIL import Image
def export_tif_custom_name(input_path, output_name):
# 加载TIF文件
image = Image.open(input_path)
# 导出为自定义名称
image.save(output_name)
示例调用
input_path = "example.tif"
output_name = "custom_example.tif"
export_tif_custom_name(input_path, output_name)
五、处理批量文件
有时候我们可能需要处理多个TIF文件,并将它们导出为不同的自定义名称。以下是一个示例代码,展示了如何批量处理多个TIF文件:
import os
from PIL import Image
def batch_export_tif_custom_name(input_dir, output_dir, name_prefix):
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for file_name in os.listdir(input_dir):
if file_name.endswith(".tif"):
input_path = os.path.join(input_dir, file_name)
output_name = os.path.join(output_dir, f"{name_prefix}_{file_name}")
# 加载并保存图像
image = Image.open(input_path)
image.save(output_name)
示例调用
input_dir = "input_directory"
output_dir = "output_directory"
name_prefix = "custom"
batch_export_tif_custom_name(input_dir, output_dir, name_prefix)
六、处理图像格式转换
有时候我们可能需要将TIF文件转换为其他格式,如JPEG或PNG。以下是一个示例代码,展示了如何将TIF文件转换为其他格式并保存为自定义名称:
from PIL import Image
def convert_tif_to_format(input_path, output_name, output_format):
# 加载TIF文件
image = Image.open(input_path)
# 导出为指定格式和自定义名称
image.save(output_name, format=output_format)
示例调用
input_path = "example.tif"
output_name = "custom_example.jpeg"
output_format = "JPEG"
convert_tif_to_format(input_path, output_name, output_format)
七、处理图像的其他操作
在处理TIF文件时,我们可能需要进行其他操作,如调整图像大小、裁剪图像或应用滤镜。以下是一些示例代码,展示了如何进行这些操作:
调整图像大小
def resize_image(input_path, output_name, size):
# 加载图像
image = Image.open(input_path)
# 调整图像大小
resized_image = image.resize(size)
# 保存调整后的图像
resized_image.save(output_name)
示例调用
input_path = "example.tif"
output_name = "resized_example.tif"
size = (800, 600)
resize_image(input_path, output_name, size)
裁剪图像
def crop_image(input_path, output_name, crop_box):
# 加载图像
image = Image.open(input_path)
# 裁剪图像
cropped_image = image.crop(crop_box)
# 保存裁剪后的图像
cropped_image.save(output_name)
示例调用
input_path = "example.tif"
output_name = "cropped_example.tif"
crop_box = (100, 100, 400, 400) # (left, upper, right, lower)
crop_image(input_path, output_name, crop_box)
应用滤镜
from PIL import ImageFilter
def apply_filter(input_path, output_name, filter_type):
# 加载图像
image = Image.open(input_path)
# 应用滤镜
filtered_image = image.filter(filter_type)
# 保存滤镜后的图像
filtered_image.save(output_name)
示例调用
input_path = "example.tif"
output_name = "filtered_example.tif"
filter_type = ImageFilter.BLUR # 例如:ImageFilter.BLUR, ImageFilter.CONTOUR 等
apply_filter(input_path, output_name, filter_type)
八、总结
以上展示了如何通过Python和Pillow库来将TIF文件导出为自定义名称,并进行了图像的批量处理、格式转换、大小调整、裁剪和滤镜应用等操作。这些操作可以帮助我们更好地处理和管理图像文件。在实际应用中,我们可以根据具体需求对代码进行调整和扩展,以实现更多功能。希望这些示例代码能对您有所帮助。
相关问答FAQs:
如何在Python中自定义导出TIF文件的名称?
在Python中,可以使用图像处理库如PIL(Pillow)或OpenCV来处理和导出TIF文件。通过在保存文件时指定自定义名称,可以轻松实现这一点。示例如下:
from PIL import Image
# 打开TIF文件
image = Image.open('input.tif')
# 自定义名称
custom_name = 'my_custom_name.tif'
# 导出为自定义名称
image.save(custom_name)
通过这种方式,你可以根据需要为导出的TIF文件设置任何名称。
导出TIF文件时有哪些格式选项可以选择?
在导出TIF文件时,除了自定义文件名外,还可以选择不同的参数和格式选项。例如,使用Pillow库时,可以指定压缩方式,如“TIFF.LZW”或“TIFF.NONE”,以满足特定的存储需求。代码示例如下:
image.save(custom_name, compression='tiff_lzw')
这将以LZW压缩格式导出文件,减少文件大小,同时保持图像质量。
如何处理多个TIF文件并同时导出自定义名称?
如果需要处理多个TIF文件并为它们导出不同的自定义名称,可以使用循环和字符串格式化。以下是一个简单的示例:
import glob
# 获取当前目录下所有的TIF文件
files = glob.glob('*.tif')
for index, file in enumerate(files):
image = Image.open(file)
custom_name = f'output_image_{index + 1}.tif'
image.save(custom_name)
这种方法允许你为每个文件生成一个唯一的自定义名称,方便管理和存储。
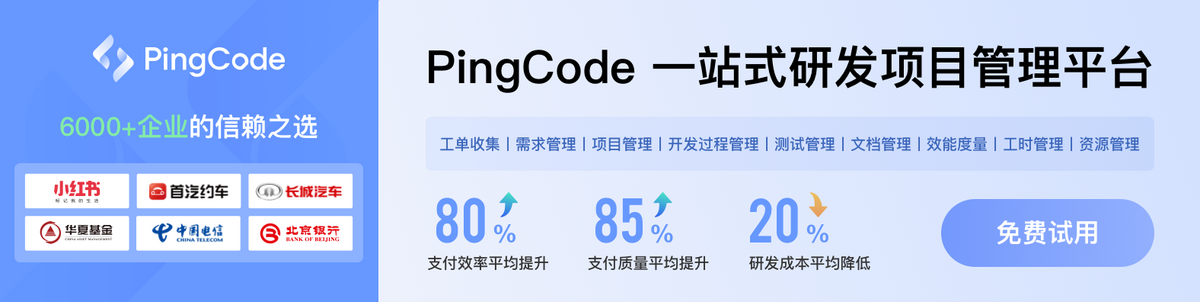