用Python写一个选择题程序的方法有很多,其中最常用的方式包括:使用基础的控制结构、利用面向对象编程、集成图形用户界面(GUI)等。 下面我们将详细介绍一种基本的实现方法,并逐步扩展到更加复杂的版本。
基础选择题程序
用Python写一个简单的选择题程序,可以通过使用基础的控制结构,如循环和条件判断。以下是一个简单的实现:
def run_quiz():
questions = [
{
"question": "What is the capital of France?",
"options": ["A) Paris", "B) London", "C) Berlin", "D) Madrid"],
"answer": "A"
},
{
"question": "What is 2 + 2?",
"options": ["A) 3", "B) 4", "C) 5", "D) 6"],
"answer": "B"
}
]
score = 0
for question in questions:
print(question["question"])
for option in question["options"]:
print(option)
answer = input("Enter your answer: ").strip().upper()
if answer == question["answer"]:
score += 1
print(f"Your final score is {score} out of {len(questions)}")
if __name__ == "__main__":
run_quiz()
一、扩展基础程序
在基础程序之上,我们可以通过以下几种方式扩展和增强功能:
- 增加题库: 扩展题目数量和复杂度。
- 增加题目类型: 除了选择题,还可以加入判断题、填空题等。
- 分数计算: 提供详细的分数计算及反馈。
- 用户界面: 使用图形用户界面(如Tkinter)提升用户体验。
二、增加题库和题目类型
增加题库和题目类型可以使选择题程序更加丰富和有挑战性。我们可以通过增加更多的题目和不同类型的题目来实现这一点。
def run_quiz():
questions = [
{
"question": "What is the capital of France?",
"options": ["A) Paris", "B) London", "C) Berlin", "D) Madrid"],
"answer": "A"
},
{
"question": "What is 2 + 2?",
"options": ["A) 3", "B) 4", "C) 5", "D) 6"],
"answer": "B"
},
{
"question": "Is Python a programming language? (True/False)",
"options": ["A) True", "B) False"],
"answer": "A"
},
{
"question": "Complete the following: 3 + 5 = _",
"options": [],
"answer": "8"
}
]
score = 0
for question in questions:
print(question["question"])
for option in question["options"]:
print(option)
answer = input("Enter your answer: ").strip().upper()
if answer == question["answer"]:
score += 1
print(f"Your final score is {score} out of {len(questions)}")
if __name__ == "__main__":
run_quiz()
三、分数计算和反馈
提供详细的分数计算和反馈可以让用户了解自己的表现。可以在每道题目后提供即时反馈,并在最后给出总体评价。
def run_quiz():
questions = [
{
"question": "What is the capital of France?",
"options": ["A) Paris", "B) London", "C) Berlin", "D) Madrid"],
"answer": "A"
},
{
"question": "What is 2 + 2?",
"options": ["A) 3", "B) 4", "C) 5", "D) 6"],
"answer": "B"
},
{
"question": "Is Python a programming language? (True/False)",
"options": ["A) True", "B) False"],
"answer": "A"
},
{
"question": "Complete the following: 3 + 5 = _",
"options": [],
"answer": "8"
}
]
score = 0
for question in questions:
print(question["question"])
for option in question["options"]:
print(option)
answer = input("Enter your answer: ").strip().upper()
if answer == question["answer"]:
print("Correct!")
score += 1
else:
print("Wrong!")
print(f"Your final score is {score} out of {len(questions)}")
if score == len(questions):
print("Excellent! You got all the questions right.")
elif score >= len(questions) / 2:
print("Good job! You got more than half of the questions right.")
else:
print("You need more practice. Better luck next time!")
if __name__ == "__main__":
run_quiz()
四、用户界面(GUI)
使用图形用户界面可以提升用户体验。我们可以使用Tkinter库来创建一个简单的选择题界面。
import tkinter as tk
from tkinter import messagebox
questions = [
{
"question": "What is the capital of France?",
"options": ["Paris", "London", "Berlin", "Madrid"],
"answer": "Paris"
},
{
"question": "What is 2 + 2?",
"options": ["3", "4", "5", "6"],
"answer": "4"
},
{
"question": "Is Python a programming language?",
"options": ["True", "False"],
"answer": "True"
},
{
"question": "Complete the following: 3 + 5 = _",
"options": [],
"answer": "8"
}
]
class QuizApp:
def __init__(self, root):
self.root = root
self.root.title("Quiz App")
self.current_question = 0
self.score = 0
self.display_question()
def display_question(self):
question = questions[self.current_question]
self.question_label = tk.Label(self.root, text=question["question"])
self.question_label.pack()
self.options_var = tk.StringVar()
if question["options"]:
for option in question["options"]:
tk.Radiobutton(self.root, text=option, variable=self.options_var, value=option).pack()
else:
self.answer_entry = tk.Entry(self.root)
self.answer_entry.pack()
self.submit_button = tk.Button(self.root, text="Submit", command=self.check_answer)
self.submit_button.pack()
def check_answer(self):
question = questions[self.current_question]
if question["options"]:
answer = self.options_var.get()
else:
answer = self.answer_entry.get()
if answer == question["answer"]:
self.score += 1
self.current_question += 1
self.clear_widgets()
if self.current_question < len(questions):
self.display_question()
else:
self.show_result()
def clear_widgets(self):
for widget in self.root.winfo_children():
widget.destroy()
def show_result(self):
result_text = f"Your final score is {self.score} out of {len(questions)}"
tk.Label(self.root, text=result_text).pack()
if self.score == len(questions):
tk.Label(self.root, text="Excellent! You got all the questions right.").pack()
elif self.score >= len(questions) / 2:
tk.Label(self.root, text="Good job! You got more than half of the questions right.").pack()
else:
tk.Label(self.root, text="You need more practice. Better luck next time!").pack()
if __name__ == "__main__":
root = tk.Tk()
app = QuizApp(root)
root.mainloop()
通过上述步骤,我们可以看到,从基础的选择题程序到扩展题库、提供即时反馈,再到使用图形用户界面,Python可以灵活地实现各种复杂程度的选择题程序。我们可以根据具体需求,不断优化和扩展程序的功能和用户体验。
相关问答FAQs:
如何使用Python创建选择题程序的基本步骤是什么?
要创建一个选择题程序,可以按照以下步骤进行:首先,定义一个包含问题及其选项的字典或列表。接着,使用循环遍历这些问题,向用户展示每个问题及其选项。然后,接收用户的输入并判断答案是否正确。最后,记录用户的得分并在程序结束时展示结果。
我可以在选择题程序中添加多种题型吗?
是的,您可以在选择题程序中添加多种题型,例如单选题、多选题和填空题。通过在程序中设置不同的输入方式和答案验证逻辑,您可以使程序更具互动性和趣味性。
如何提高选择题程序的用户体验?
提升用户体验可以从多个方面入手。设计清晰的界面,使用简洁易懂的语言,以及提供反馈信息(如正确答案和解释)都是有效的方法。此外,可以考虑增加计时功能或难度等级,让用户在游戏中享受更多挑战。
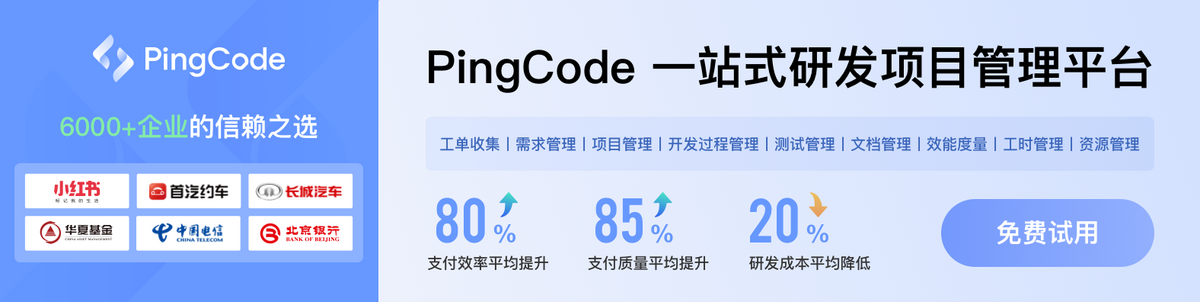