要在一个页面上绘制多个图像,可以使用Python中的Matplotlib库,具体步骤包括:创建多个子图、使用plt.subplot
、调整布局。
其中,创建多个子图是实现这一目标的关键步骤。使用plt.subplot
方法可以在一个页面上布置多个图像。接下来,我们将详细介绍如何使用这些方法来实现多个图像在一个页面上的绘制。
一、MATPLOTLIB库介绍
1、什么是Matplotlib
Matplotlib是Python中最常用的绘图库之一,提供了一整套绘图API,可以用于生成高质量的图表。它支持多种图表类型,包括线图、柱状图、饼图、散点图等等。Matplotlib的核心组件是pyplot
模块,该模块提供了一系列函数来创建和操作图表。
2、安装Matplotlib
在开始使用Matplotlib之前,需要先安装这个库。可以通过pip来安装:
pip install matplotlib
安装完成后,可以通过导入matplotlib.pyplot
模块来使用该库:
import matplotlib.pyplot as plt
二、创建多个子图
1、使用plt.subplot
方法
Matplotlib提供了plt.subplot
方法,可以在一个页面上创建多个子图。plt.subplot
方法的基本语法如下:
plt.subplot(nrows, ncols, index)
其中:
nrows
:子图的行数。ncols
:子图的列数。index
:子图的索引,从1开始。
例如,创建一个包含2行2列的子图布局,并在每个子图中绘制一个简单的线图:
import matplotlib.pyplot as plt
创建2x2的子图布局
plt.subplot(2, 2, 1)
plt.plot([1, 2, 3], [1, 4, 9])
plt.title('Subplot 1')
plt.subplot(2, 2, 2)
plt.plot([1, 2, 3], [1, 2, 3])
plt.title('Subplot 2')
plt.subplot(2, 2, 3)
plt.plot([1, 2, 3], [1, 0, 1])
plt.title('Subplot 3')
plt.subplot(2, 2, 4)
plt.plot([1, 2, 3], [3, 2, 1])
plt.title('Subplot 4')
显示图表
plt.tight_layout()
plt.show()
2、调整子图布局
使用plt.subplot
方法创建的子图布局默认是紧凑的,但有时可能需要调整子图之间的间距。可以使用plt.tight_layout
方法自动调整子图布局,使其更加美观:
plt.tight_layout()
另外,还可以使用plt.subplots_adjust
方法手动调整子图之间的间距:
plt.subplots_adjust(left=0.1, right=0.9, top=0.9, bottom=0.1, wspace=0.4, hspace=0.4)
其中:
left
、right
、top
、bottom
:分别表示子图布局的左、右、上、下边距。wspace
:表示子图之间的水平间距。hspace
:表示子图之间的垂直间距。
三、使用plt.subplots
方法
1、创建多个子图
除了plt.subplot
方法,Matplotlib还提供了plt.subplots
方法,可以更方便地创建多个子图。plt.subplots
方法的基本语法如下:
fig, axes = plt.subplots(nrows, ncols)
其中:
nrows
:子图的行数。ncols
:子图的列数。fig
:表示整个图表对象。axes
:表示子图对象数组。
例如,创建一个包含2行2列的子图布局,并在每个子图中绘制一个简单的线图:
import matplotlib.pyplot as plt
创建2x2的子图布局
fig, axes = plt.subplots(2, 2)
绘制子图
axes[0, 0].plot([1, 2, 3], [1, 4, 9])
axes[0, 0].set_title('Subplot 1')
axes[0, 1].plot([1, 2, 3], [1, 2, 3])
axes[0, 1].set_title('Subplot 2')
axes[1, 0].plot([1, 2, 3], [1, 0, 1])
axes[1, 0].set_title('Subplot 3')
axes[1, 1].plot([1, 2, 3], [3, 2, 1])
axes[1, 1].set_title('Subplot 4')
调整布局
plt.tight_layout()
plt.show()
2、共享轴
在某些情况下,可能需要共享子图的轴。例如,创建一个包含2行2列的子图布局,并共享所有子图的x轴和y轴:
import matplotlib.pyplot as plt
创建2x2的子图布局,并共享x轴和y轴
fig, axes = plt.subplots(2, 2, sharex=True, sharey=True)
绘制子图
axes[0, 0].plot([1, 2, 3], [1, 4, 9])
axes[0, 0].set_title('Subplot 1')
axes[0, 1].plot([1, 2, 3], [1, 2, 3])
axes[0, 1].set_title('Subplot 2')
axes[1, 0].plot([1, 2, 3], [1, 0, 1])
axes[1, 0].set_title('Subplot 3')
axes[1, 1].plot([1, 2, 3], [3, 2, 1])
axes[1, 1].set_title('Subplot 4')
调整布局
plt.tight_layout()
plt.show()
通过sharex
和sharey
参数,可以方便地共享子图的x轴和y轴。
四、使用GridSpec方法
1、什么是GridSpec
GridSpec是Matplotlib中的一个类,用于定义子图的布局。使用GridSpec可以创建更加复杂和灵活的子图布局。GridSpec类的基本语法如下:
import matplotlib.gridspec as gridspec
gs = gridspec.GridSpec(nrows, ncols)
其中:
nrows
:子图的行数。ncols
:子图的列数。
例如,创建一个包含2行3列的子图布局:
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
创建GridSpec对象
gs = gridspec.GridSpec(2, 3)
绘制子图
plt.subplot(gs[0, 0])
plt.plot([1, 2, 3], [1, 4, 9])
plt.title('Subplot 1')
plt.subplot(gs[0, 1])
plt.plot([1, 2, 3], [1, 2, 3])
plt.title('Subplot 2')
plt.subplot(gs[0, 2])
plt.plot([1, 2, 3], [1, 0, 1])
plt.title('Subplot 3')
plt.subplot(gs[1, :])
plt.plot([1, 2, 3], [3, 2, 1])
plt.title('Subplot 4')
调整布局
plt.tight_layout()
plt.show()
2、灵活的子图布局
使用GridSpec可以创建更加灵活的子图布局。例如,创建一个包含3行3列的子图布局,并在其中绘制不同大小的子图:
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
创建GridSpec对象
gs = gridspec.GridSpec(3, 3)
绘制子图
plt.subplot(gs[0, 0])
plt.plot([1, 2, 3], [1, 4, 9])
plt.title('Subplot 1')
plt.subplot(gs[0, 1:3])
plt.plot([1, 2, 3], [1, 2, 3])
plt.title('Subplot 2')
plt.subplot(gs[1:, 0])
plt.plot([1, 2, 3], [1, 0, 1])
plt.title('Subplot 3')
plt.subplot(gs[1:, 1:])
plt.plot([1, 2, 3], [3, 2, 1])
plt.title('Subplot 4')
调整布局
plt.tight_layout()
plt.show()
通过指定子图占用的行和列范围,可以创建不同大小的子图。
五、在子图中绘制不同类型的图表
1、线图
可以在子图中绘制简单的线图。例如,在一个包含2行2列的子图布局中,绘制4个不同的线图:
import matplotlib.pyplot as plt
创建2x2的子图布局
fig, axes = plt.subplots(2, 2)
绘制子图
axes[0, 0].plot([1, 2, 3], [1, 4, 9])
axes[0, 0].set_title('Line Plot 1')
axes[0, 1].plot([1, 2, 3], [1, 2, 3])
axes[0, 1].set_title('Line Plot 2')
axes[1, 0].plot([1, 2, 3], [1, 0, 1])
axes[1, 0].set_title('Line Plot 3')
axes[1, 1].plot([1, 2, 3], [3, 2, 1])
axes[1, 1].set_title('Line Plot 4')
调整布局
plt.tight_layout()
plt.show()
2、柱状图
可以在子图中绘制柱状图。例如,在一个包含2行2列的子图布局中,绘制4个不同的柱状图:
import matplotlib.pyplot as plt
创建2x2的子图布局
fig, axes = plt.subplots(2, 2)
绘制子图
axes[0, 0].bar([1, 2, 3], [1, 4, 9])
axes[0, 0].set_title('Bar Plot 1')
axes[0, 1].bar([1, 2, 3], [1, 2, 3])
axes[0, 1].set_title('Bar Plot 2')
axes[1, 0].bar([1, 2, 3], [1, 0, 1])
axes[1, 0].set_title('Bar Plot 3')
axes[1, 1].bar([1, 2, 3], [3, 2, 1])
axes[1, 1].set_title('Bar Plot 4')
调整布局
plt.tight_layout()
plt.show()
3、散点图
可以在子图中绘制散点图。例如,在一个包含2行2列的子图布局中,绘制4个不同的散点图:
import matplotlib.pyplot as plt
创建2x2的子图布局
fig, axes = plt.subplots(2, 2)
绘制子图
axes[0, 0].scatter([1, 2, 3], [1, 4, 9])
axes[0, 0].set_title('Scatter Plot 1')
axes[0, 1].scatter([1, 2, 3], [1, 2, 3])
axes[0, 1].set_title('Scatter Plot 2')
axes[1, 0].scatter([1, 2, 3], [1, 0, 1])
axes[1, 0].set_title('Scatter Plot 3')
axes[1, 1].scatter([1, 2, 3], [3, 2, 1])
axes[1, 1].set_title('Scatter Plot 4')
调整布局
plt.tight_layout()
plt.show()
六、在子图中添加图例和注释
1、添加图例
可以在子图中添加图例,以便更好地解释图表内容。例如,在一个包含2行2列的子图布局中,绘制4个不同的线图,并添加图例:
import matplotlib.pyplot as plt
创建2x2的子图布局
fig, axes = plt.subplots(2, 2)
绘制子图
axes[0, 0].plot([1, 2, 3], [1, 4, 9], label='Line 1')
axes[0, 0].set_title('Line Plot 1')
axes[0, 0].legend()
axes[0, 1].plot([1, 2, 3], [1, 2, 3], label='Line 2')
axes[0, 1].set_title('Line Plot 2')
axes[0, 1].legend()
axes[1, 0].plot([1, 2, 3], [1, 0, 1], label='Line 3')
axes[1, 0].set_title('Line Plot 3')
axes[1, 0].legend()
axes[1, 1].plot([1, 2, 3], [3, 2, 1], label='Line 4')
axes[1, 1].set_title('Line Plot 4')
axes[1, 1].legend()
调整布局
plt.tight_layout()
plt.show()
2、添加注释
可以在子图中添加注释,以便更好地解释图表内容。例如,在一个包含2行2列的子图布局中,绘制4个不同的线图,并添加注释:
import matplotlib.pyplot as plt
创建2x2的子图布局
fig, axes = plt.subplots(2, 2)
绘制子图
axes[0, 0].plot([1, 2, 3], [1, 4, 9])
axes[0, 0].set_title('Line Plot 1')
axes[0, 0].annotate('Peak', xy=(2, 4), xytext=(2, 7),
arrowprops=dict(facecolor='black', shrink=0.05))
axes[0, 1].plot([1, 2, 3], [1, 2, 3])
axes[0, 1].set_title('Line Plot 2')
axes[0, 1].annotate('Point', xy=(2, 2), xytext=(2, 4),
arrowprops=dict(facecolor='black', shrink=0.05))
axes[1, 0].plot([1, 2, 3], [1, 0, 1])
axes[1, 0].set_title('Line Plot 3')
axes[1, 0].annotate('Valley', xy=(2, 0), xytext=(2, 2),
arrowprops=dict(facecolor='black', shrink=0.05))
axes[1, 1].plot([1, 2, 3], [3, 2, 1])
axes[1, 1].set_title('Line Plot 4')
axes[1, 1].annotate('Decrease', xy=(2, 2), xytext=(2, 4),
arrowprops=dict(facecolor='black', shrink=0.05))
调整布局
plt.tight_layout()
plt.show()
七、总结
使用Matplotlib库可以方便地在一个页面上绘制多个图像。通过plt.subplot
、plt.subplots
和GridSpec方法,可以创建不同布局的子图,并在子图中绘制不同类型的图表。此外,还可以在子图中添加图例和注释,以便更好地解释图表内容。希望本文能帮助你更好地理解如何在一个页面上绘制多个图像,并应用到实际项目中。
相关问答FAQs:
如何在Python中使用Matplotlib绘制多个图像?
在Python中,可以使用Matplotlib库中的subplot
功能来在一个页面上绘制多个图像。首先,确保你安装了Matplotlib库。然后,通过创建多个子图,可以在同一窗口中显示不同的图形。例如,使用plt.subplot(2, 2, 1)
可以在2行2列的布局中选择第一个位置绘制图像,依此类推。
在一个图像中绘制多个数据系列的最佳方法是什么?
要在同一个图像中展示多个数据系列,可以使用Matplotlib的plot
函数,并在同一图形上多次调用它。通过指定不同的颜色和样式,可以清晰地区分各个数据系列。此外,可以使用图例功能来标识每个系列,确保观众能够理解每条线代表的数据。
如何控制多个图像之间的间距和布局?
在使用Matplotlib绘制多个图像时,可以使用plt.subplots_adjust()
来调整图像之间的间距。通过设置wspace
和hspace
参数,可以控制子图的水平和垂直间距。此外,使用fig.tight_layout()
可以自动优化布局,确保各个图像不会重叠,从而提升可读性。
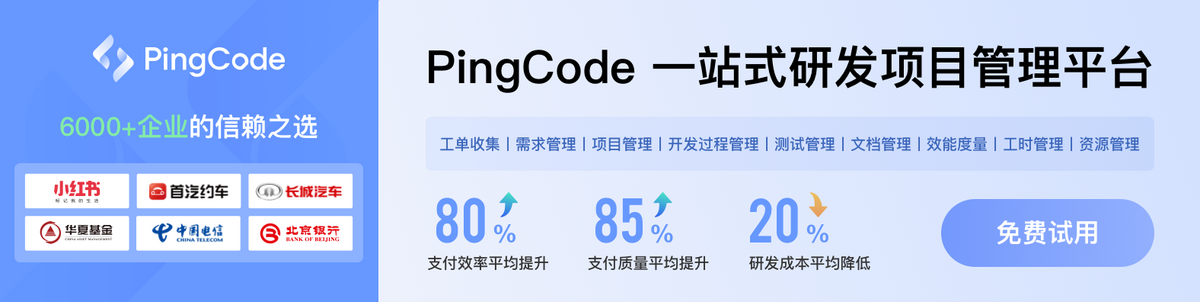