用Python将文本中无意义的符号去除,可以通过多种方法实现,包括使用正则表达式、字符串方法和第三方库等。最常见的方法是使用正则表达式来匹配并删除特定的无意义符号。正则表达式功能强大、灵活、适应性强,能够处理多种复杂的文本格式。举个例子,正则表达式可以轻松识别和删除标点符号、特殊字符以及其他不需要的符号,使你的文本更加干净和易于处理。
用Python将文本中无意义的符号去除
一、正则表达式
正则表达式(Regular Expression,简称regex)是一个非常强大的工具,可以用来匹配复杂的字符串模式。在Python中,我们可以通过re
模块来使用正则表达式。
1、基础用法
正则表达式的基本用法是使用re.sub()
函数来替换不需要的符号。下面是一个简单的例子:
import re
text = "Hello, World! This is a test text with some #special# characters."
cleaned_text = re.sub(r'[^\w\s]', '', text)
print(cleaned_text)
在这个例子中,re.sub(r'[^\w\s]', '', text)
将所有非字母、数字和空白符号的字符都替换为空字符串,从而去掉了无意义的符号。
2、复杂匹配
对于更复杂的文本处理需求,我们可以使用更复杂的正则表达式模式。例如,我们想要去除所有的HTML标签:
text = "<div>Hello, <b>World</b>! This is a <i>test</i> text with some HTML tags.</div>"
cleaned_text = re.sub(r'<.*?>', '', text)
print(cleaned_text)
在这个例子中,re.sub(r'<.*?>', '', text)
将所有的HTML标签替换为空字符串。
二、字符串方法
除了使用正则表达式,我们还可以使用Python的字符串方法来去除无意义的符号。
1、str.replace()
str.replace()
方法可以用来替换字符串中的特定字符。例如:
text = "Hello, World! This is a test text with some #special# characters."
cleaned_text = text.replace('#', '').replace('!', '').replace(',', '')
print(cleaned_text)
虽然str.replace()
方法比较简单,但它只能处理特定的字符,对于复杂的符号匹配还是需要正则表达式。
2、str.translate()
str.translate()
方法更加高效,适合处理大量的字符替换。我们可以使用str.maketrans()
来创建一个翻译表,然后使用str.translate()
来进行替换:
text = "Hello, World! This is a test text with some #special# characters."
translator = str.maketrans('', '', '#!,')
cleaned_text = text.translate(translator)
print(cleaned_text)
三、第三方库
对于一些更复杂的文本处理需求,我们可以使用一些第三方库,比如nltk
和textacy
。
1、nltk
nltk
是一个非常强大的自然语言处理库,可以用来进行文本预处理。例如,我们可以使用nltk
来去除标点符号:
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
import string
nltk.download('punkt')
nltk.download('stopwords')
text = "Hello, World! This is a test text with some #special# characters."
tokens = word_tokenize(text)
cleaned_tokens = [word for word in tokens if word.isalnum()]
cleaned_text = ' '.join(cleaned_tokens)
print(cleaned_text)
2、textacy
textacy
是另一个强大的文本处理库,可以用来进行更复杂的文本清理操作。例如,我们可以使用textacy
来去除特定的符号:
import textacy.preprocessing as tp
text = "Hello, World! This is a test text with some #special# characters."
cleaned_text = tp.remove_punctuation(text)
print(cleaned_text)
四、结合多种方法
在实际项目中,我们通常需要结合多种方法来处理文本。下面是一个综合示例,展示了如何结合正则表达式、字符串方法和第三方库来进行文本清理:
import re
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
import string
import textacy.preprocessing as tp
nltk.download('punkt')
nltk.download('stopwords')
def clean_text(text):
# Step 1: Remove HTML tags
text = re.sub(r'<.*?>', '', text)
# Step 2: Remove special characters
translator = str.maketrans('', '', string.punctuation)
text = text.translate(translator)
# Step 3: Tokenize and remove non-alphanumeric tokens
tokens = word_tokenize(text)
cleaned_tokens = [word for word in tokens if word.isalnum()]
# Step 4: Join tokens back into a single string
cleaned_text = ' '.join(cleaned_tokens)
return cleaned_text
text = "<div>Hello, World! This is a <b>test</b> text with some #special# characters.</div>"
cleaned_text = clean_text(text)
print(cleaned_text)
在这个综合示例中,我们首先使用正则表达式去除HTML标签,然后使用str.translate()
方法去除标点符号,接着使用nltk
进行分词和去除非字母数字的词,最后将清理后的词组合成一个字符串。
五、性能优化
在处理大量文本时,性能是一个需要考虑的重要因素。以下是一些优化建议:
1、批量处理
对于大量文本,可以使用批量处理的方式来提高效率。例如:
def batch_clean_text(texts):
cleaned_texts = []
for text in texts:
cleaned_text = clean_text(text)
cleaned_texts.append(cleaned_text)
return cleaned_texts
texts = [
"<div>Hello, World! This is a <b>test</b> text with some #special# characters.</div>",
"<div>Another example text with <i>HTML</i> tags and special characters!</div>"
]
cleaned_texts = batch_clean_text(texts)
print(cleaned_texts)
2、多线程处理
对于非常大的数据集,可以考虑使用多线程或多进程来加速处理。例如:
from concurrent.futures import ThreadPoolExecutor
def clean_text(text):
# (使用上文中定义的clean_text函数)
pass
def batch_clean_text_multithread(texts):
with ThreadPoolExecutor(max_workers=4) as executor:
cleaned_texts = list(executor.map(clean_text, texts))
return cleaned_texts
texts = [
"<div>Hello, World! This is a <b>test</b> text with some #special# characters.</div>",
"<div>Another example text with <i>HTML</i> tags and special characters!</div>"
]
cleaned_texts = batch_clean_text_multithread(texts)
print(cleaned_texts)
通过这些方法,可以显著提高文本处理的效率。
总结
去除文本中无意义的符号是文本预处理的重要步骤,直接影响到后续的自然语言处理和数据分析的效果。本文介绍了如何使用正则表达式、字符串方法和第三方库来清理文本,并提供了性能优化的建议。通过合理结合这些方法,可以有效地去除无意义的符号,使文本更加干净和易于处理。
相关问答FAQs:
如何识别和删除文本中的无意义符号?
在使用Python处理文本时,可以利用正则表达式(re
模块)来识别并删除无意义的符号。通过编写合适的正则表达式,可以轻松匹配并去除特定的符号。例如,使用re.sub(r'[^a-zA-Z0-9\s]', '', text)
可以删除所有非字母、数字和空格的字符,从而清理文本。
在Python中处理文本时,如何保持重要的标点符号?
在清理文本时,有时需要保留某些标点符号,例如句号、逗号等。可以通过修改正则表达式来实现这一点。例如,使用re.sub(r'[^a-zA-Z0-9\s.,!?]', '', text)
将只删除不必要的符号,同时保留句号、逗号和其他所需标点符号。
使用Python清洗文本数据时,有哪些常见的无意义符号?
常见的无意义符号包括特殊字符(如@、#、$、%)、多余的空格、换行符以及任何与文本内容无关的符号。通过分析数据源,可以识别出这些符号并使用Python脚本进行清理,以提高文本分析的准确性和效率。
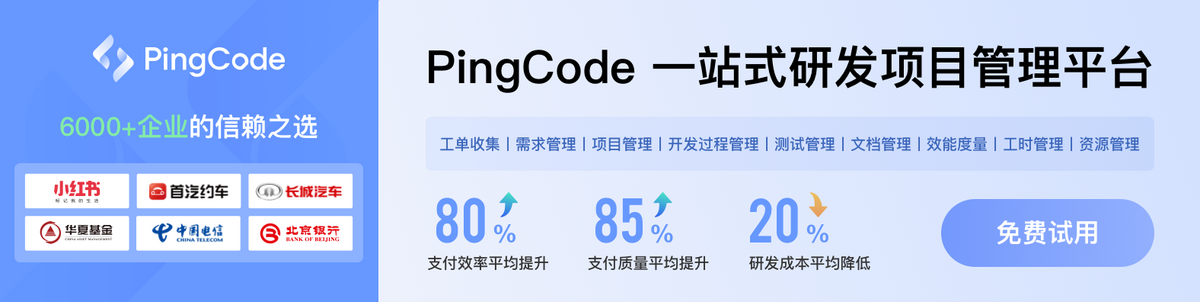