用Python格式化输出字符串的主要方法有三种:百分号格式化、str.format()方法、f-string(格式化字符串字面量)。 在这三种方法中,f-string 是最现代且推荐使用的方法,因为它简洁、直观且性能更好。接下来,我们将详细介绍这三种方法,并提供一些实际应用中的示例。
一、百分号格式化
百分号格式化是Python中最早期的字符串格式化方法。尽管这种方法现在不推荐使用,但了解它在维护旧代码时仍然很有用。
基本用法
百分号格式化通过在字符串中使用 %
符号来指定占位符。以下是一些常见的占位符:
%s
:字符串%d
:整数%f
:浮点数
示例
name = "Alice"
age = 30
height = 5.6
formatted_string = "Name: %s, Age: %d, Height: %.1f" % (name, age, height)
print(formatted_string)
在上述示例中,%s
被 name
替换,%d
被 age
替换,%.1f
被 height
替换,并且只保留一位小数。
优缺点
- 优点:简单直观,适用于简单的字符串格式化。
- 缺点:不支持复杂的格式化需求,语法不够灵活。
二、str.format()方法
str.format()
方法是Python 3引入的,提供了更强大的格式化功能和更灵活的语法。
基本用法
str.format()
方法通过在字符串中使用花括号 {}
来指定占位符。以下是一些常见的用法:
name = "Alice"
age = 30
height = 5.6
formatted_string = "Name: {}, Age: {}, Height: {:.1f}".format(name, age, height)
print(formatted_string)
在上述示例中,花括号 {}
被对应的变量替换,{:.1f}
指定保留一位小数。
高级用法
str.format()
支持位置参数和关键字参数:
formatted_string = "Name: {0}, Age: {1}, Height: {2:.1f}".format(name, age, height)
print(formatted_string)
formatted_string = "Name: {name}, Age: {age}, Height: {height:.1f}".format(name=name, age=age, height=height)
print(formatted_string)
优缺点
- 优点:功能强大,支持复杂的格式化需求。
- 缺点:语法相对冗长,不够简洁。
三、f-string(格式化字符串字面量)
f-string 是Python 3.6引入的,提供了最简洁和高效的字符串格式化方法。
基本用法
f-string 通过在字符串前加 f
或 F
,并在花括号 {}
中直接插入变量或表达式:
name = "Alice"
age = 30
height = 5.6
formatted_string = f"Name: {name}, Age: {age}, Height: {height:.1f}"
print(formatted_string)
在上述示例中,花括号 {}
中的变量和表达式会被直接替换。
高级用法
f-string 支持任意Python表达式:
import datetime
now = datetime.datetime.now()
formatted_string = f"Current date and time: {now:%Y-%m-%d %H:%M:%S}"
print(formatted_string)
formatted_string = f"2 + 2 = {2 + 2}"
print(formatted_string)
优缺点
- 优点:简洁直观,支持复杂的格式化需求,性能更好。
- 缺点:仅支持Python 3.6及以上版本。
四、使用场景和最佳实践
日常字符串拼接
在日常开发中,f-string 是最推荐使用的方法,因为它简洁易读:
name = "Alice"
age = 30
print(f"Name: {name}, Age: {age}")
打印调试信息
在调试代码时,f-string 可以快速插入变量值:
debug_info = f"Variable x: {x}, Variable y: {y}"
print(debug_info)
生成复杂字符串
对于生成复杂的报告或日志,str.format()
可能更适合,因为它支持更复杂的格式化需求:
report = "Name: {name}\nAge: {age}\nHeight: {height:.1f}".format(name=name, age=age, height=height)
print(report)
兼容旧代码
在维护旧代码时,可能会遇到百分号格式化。这时,可以保持原有格式,避免大幅度修改:
name = "Alice"
age = 30
print("Name: %s, Age: %d" % (name, age))
五、常见问题和解决方法
1. 如何在字符串中插入花括号 {}
?
在字符串中插入花括号 {}
时,可以使用双花括号 {{
和 }}
:
name = "Alice"
formatted_string = f"Name: {{name}}"
print(formatted_string)
2. 如何控制浮点数的精度?
在f-string和str.format()
中,可以通过指定格式来控制浮点数的精度:
pi = 3.14159
print(f"Pi to 2 decimal places: {pi:.2f}")
print("Pi to 2 decimal places: {:.2f}".format(pi))
3. 如何格式化日期和时间?
可以使用 datetime
模块配合f-string或str.format()
来格式化日期和时间:
import datetime
now = datetime.datetime.now()
print(f"Current date and time: {now:%Y-%m-%d %H:%M:%S}")
print("Current date and time: {:%Y-%m-%d %H:%M:%S}".format(now))
4. 如何在字符串中插入百分号 %
?
在字符串中插入百分号 %
时,可以使用双百分号 %%
:
percentage = 75
print(f"Completion rate: {percentage}%")
print("Completion rate: {}%".format(percentage))
print("Completion rate: %d%%" % percentage)
5. 如何处理长字符串?
对于长字符串,可以使用多行字符串或连接多个字符串:
# 使用多行字符串
long_string = f"""
Name: {name}
Age: {age}
Height: {height:.1f}
"""
print(long_string)
连接多个字符串
long_string = (
f"Name: {name}\n"
f"Age: {age}\n"
f"Height: {height:.1f}\n"
)
print(long_string)
六、总结
Python提供了三种主要的字符串格式化方法:百分号格式化、str.format()方法、f-string(格式化字符串字面量)。 其中,f-string 是最现代且推荐使用的方法,因为它简洁、直观且性能更好。了解和掌握这三种方法,可以帮助我们在不同场景下高效地格式化字符串。无论是日常开发、打印调试信息、生成复杂字符串,还是维护旧代码,这些方法都能满足我们的需求。
希望这篇文章能够帮助你更好地理解和使用Python的字符串格式化方法。如果有任何问题或建议,欢迎在评论区留言。
相关问答FAQs:
如何在Python中实现字符串的格式化输出?
在Python中,可以使用多种方法来格式化输出字符串。最常用的方法包括使用f-string、format()方法和百分号(%)格式化。f-string是Python 3.6及更高版本中引入的,使用起来非常直观方便。例如,使用f-string可以这样写:name = "Alice"; age = 30; print(f"My name is {name} and I am {age} years old.")
。这种方式将变量直接嵌入字符串中,简洁明了。
Python中如何控制格式化字符串的精度和宽度?
在进行字符串格式化时,有时需要控制数字的精度和宽度。这可以通过在format()方法中使用格式规范来实现。例如,print("{:.2f}".format(3.14159))
会输出3.14,保留两位小数。对于宽度控制,可以使用类似于print("{:10}".format("Hello"))
的方式,这样可以确保输出的字符串在宽度为10的情况下右对齐。
如何将多个变量格式化输出为字符串?
要将多个变量格式化为一个字符串,可以使用f-string或format()方法。对于f-string,可以直接在字符串中插入多个变量,例如:name = "Bob"; score = 95; print(f"{name} scored {score} points.")
。而使用format()方法时,可以这样写:print("{} scored {} points.".format(name, score))
。这两种方法都能轻松地将多个变量合并到一个字符串中,便于输出和显示。
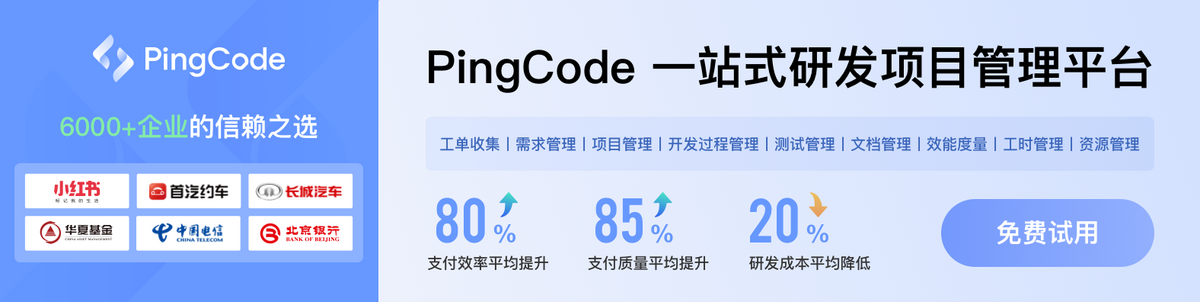