如何结束一个正在运行的Python脚本?
使用内置函数exit()
、调用sys.exit()
、触发异常、利用os._exit()
。在Python编程中,可以通过多种方式终止一个正在运行的脚本。其中,调用sys.exit()
是最常见的方法,因为它提供了更多的灵活性和控制。sys.exit()
允许您通过传递状态码来指示程序的退出状态,0表示正常退出,其他值表示异常退出。此外,sys.exit()
会引发SystemExit
异常,这样可以在需要时通过捕获该异常进行清理操作。
一、使用内置函数exit()
exit()
函数是Python内置的一个函数,主要用于在交互式环境下终止程序运行。虽然在脚本中也可以使用,但它不如sys.exit()
那样灵活。
print("This is the start of the script.")
exit()
print("This will not be printed.")
exit()
函数会立即终止程序的执行,并且不会执行后续的代码。
为什么不推荐在脚本中使用exit()
虽然exit()
可以终止脚本,但它主要是为交互式解释器设计的。在脚本或模块中使用时,它可能会导致不可预期的行为,特别是在嵌入式Python环境中。因此,sys.exit()
是更为推荐的选择。
二、调用sys.exit()
sys.exit()
是终止Python脚本的推荐方法。它提供了更多的灵活性和控制,同时还允许您传递退出状态码。
import sys
print("This is the start of the script.")
sys.exit(0)
print("This will not be printed.")
在上面的例子中,sys.exit(0)
会终止脚本,并返回一个状态码0,表示程序正常退出。
传递非零状态码
您还可以传递非零状态码,以表示程序异常退出。
import sys
print("This is the start of the script.")
sys.exit(1)
print("This will not be printed.")
在这种情况下,状态码1表示程序异常退出。这在编写命令行工具时非常有用,可以通过状态码来指示不同的错误类型。
三、触发异常
另一种结束脚本的方法是通过触发异常。虽然这不是最常见的方法,但在某些情况下可能会非常有用。
raise SystemExit("Terminating the script.")
在上面的例子中,raise SystemExit
会触发一个SystemExit
异常,从而终止脚本的执行。
捕获SystemExit
异常
如果您希望在终止脚本前执行一些清理操作,可以捕获SystemExit
异常。
try:
raise SystemExit("Terminating the script.")
except SystemExit as e:
print(f"Caught SystemExit exception: {e}")
# Perform cleanup here
在这种情况下,您可以在捕获SystemExit
异常后执行一些清理操作,然后再终止脚本。
四、利用os._exit()
os._exit()
是另一种终止脚本的方法,它会立即终止程序,而不执行任何清理操作。
import os
print("This is the start of the script.")
os._exit(0)
print("This will not be printed.")
与sys.exit()
的区别
os._exit()
和sys.exit()
的主要区别在于,os._exit()
不会引发SystemExit
异常,因此不会执行任何清理操作,例如关闭文件或释放资源。这在某些情况下可能是有用的,但一般情况下,sys.exit()
是更为推荐的选择。
五、使用threading
模块中的_stop()
在多线程环境中,有时需要终止某个特定的线程。Python的threading
模块提供了一些方法来实现这一点。
终止主线程
import threading
def thread_function(name):
print(f"Thread {name}: starting")
print(f"Thread {name}: finishing")
x = threading.Thread(target=thread_function, args=(1,))
x.start()
x.join()
print("Main thread terminating.")
threading.main_thread()._stop()
print("This will not be printed.")
终止子线程
import threading
import time
def thread_function(name):
print(f"Thread {name}: starting")
time.sleep(5)
print(f"Thread {name}: finishing")
x = threading.Thread(target=thread_function, args=(1,))
x.start()
time.sleep(1) # Give the thread some time to start
print("Main thread terminating the child thread.")
x._stop()
print("This will not be printed.")
在上面的例子中,_stop()
方法会立即终止线程的执行。
六、总结
在Python中,有多种方法可以终止一个正在运行的脚本。sys.exit()
是最推荐的方法,因为它提供了更多的控制和灵活性,允许您传递状态码并引发SystemExit
异常进行清理操作。os._exit()
和exit()
也可以用于终止脚本,但它们不如sys.exit()
那样灵活。在多线程环境中,您可以使用threading
模块中的_stop()
方法来终止特定的线程。了解这些方法及其适用场景,可以帮助您更好地控制Python脚本的执行和终止。
相关问答FAQs:
如何安全地终止一个正在运行的Python脚本?
在终止Python脚本时,建议使用Ctrl + C
组合键,这样可以安全地中断脚本的执行。此外,使用sys.exit()
函数也可以在脚本中添加退出点,以便在需要时安全地结束程序。
如果我在IDE中运行Python脚本,如何停止它?
大多数IDE(如PyCharm、VSCode等)都提供了一个停止按钮,通常是一个红色的方块,用户可以直接点击它来终止当前运行的Python脚本。这种方式通常比使用命令行更方便。
如何处理未响应的Python脚本?
当Python脚本未响应时,可以通过任务管理器(Windows)或活动监视器(Mac)手动结束该进程。在任务管理器中,找到对应的Python进程,右键选择“结束任务”即可。务必小心操作,以免意外关闭其他重要程序。
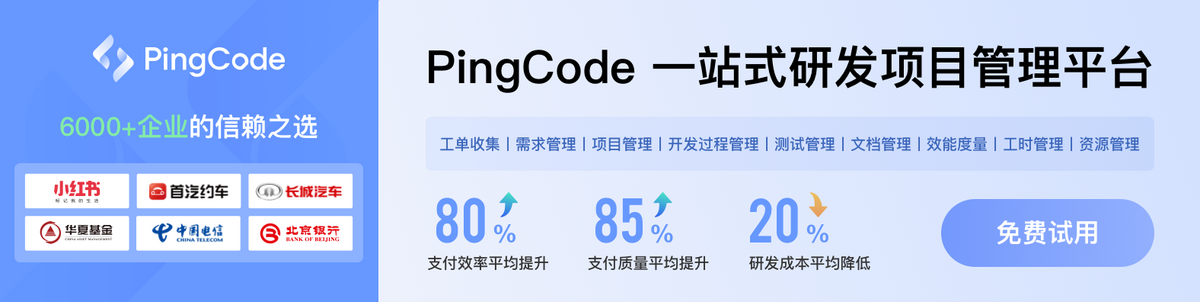