Python中提取一个标签的字符串方法、使用BeautifulSoup库解析HTML、正则表达式匹配标签内容、通过lxml库解析XML
在Python中提取一个标签的字符串有多种方法,其中使用BeautifulSoup库解析HTML、正则表达式匹配标签内容、通过lxml库解析XML是最常用的三种方式。使用BeautifulSoup库解析HTML是其中最常用且高效的方法,因为它提供了简洁的API,可以轻松地遍历、搜索和修改HTML文档。下面将详细介绍如何使用BeautifulSoup库来实现这一功能。
一、使用BeautifulSoup库解析HTML
BeautifulSoup是Python中一个用于解析HTML和XML的库。它可以将复杂的HTML文档转换成一个复杂的树形结构,每个节点都是Python对象,所有对象可以归纳为四种:Tag、NavigableString、BeautifulSoup以及Comment。
1、安装BeautifulSoup和lxml
在使用BeautifulSoup之前,需要先安装BeautifulSoup库以及解析器lxml。你可以通过pip命令来安装它们:
pip install beautifulsoup4
pip install lxml
2、解析HTML并提取标签
以下是一个示例代码,展示了如何使用BeautifulSoup库解析HTML并提取一个标签的字符串内容:
from bs4 import BeautifulSoup
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'lxml')
提取第一个<p>标签的内容
p_tag = soup.find('p')
print(p_tag.text)
输出结果将是:
The Dormouse's story
二、使用正则表达式匹配标签内容
正则表达式是一种强大的工具,可以用来匹配复杂的字符串模式。在Python中,可以使用re
模块来处理正则表达式。
1、导入re模块
首先,导入re模块:
import re
2、编写正则表达式并提取标签内容
以下是一个示例代码,展示了如何使用正则表达式提取一个标签的字符串内容:
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
使用正则表达式提取第一个<p>标签的内容
pattern = re.compile(r'<p.*?>(.*?)</p>', re.DOTALL)
matches = pattern.findall(html_doc)
for match in matches:
print(match)
输出结果将是:
<b>The Dormouse's story</b>
Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.
...
三、通过lxml库解析XML
lxml是一个非常强大的库,可以用来解析和处理XML和HTML。在解析速度和功能上,lxml都要比BeautifulSoup更强大,但是相对来说,它的API也更复杂一些。
1、安装lxml库
如果你还没有安装lxml库,可以通过pip来安装:
pip install lxml
2、解析XML并提取标签
以下是一个示例代码,展示了如何使用lxml库解析XML并提取一个标签的字符串内容:
from lxml import etree
xml_doc = """
<root>
<title>The Dormouse's story</title>
<content>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</content>
</root>
"""
tree = etree.fromstring(xml_doc)
提取第一个<p>标签的内容
p_tag = tree.xpath('//p')[0]
print(p_tag.text)
输出结果将是:
The Dormouse's story
四、总结
在Python中提取一个标签的字符串有多种方法,其中使用BeautifulSoup库解析HTML、正则表达式匹配标签内容、通过lxml库解析XML是最常用的三种方式。使用BeautifulSoup库解析HTML是其中最常用且高效的方法,因为它提供了简洁的API,可以轻松地遍历、搜索和修改HTML文档。无论你选择哪种方法,都需要根据具体的需求和数据格式来选择最适合的工具和技术。
相关问答FAQs:
如何在Python中读取HTML文档以提取标签内的字符串?
在Python中,可以使用BeautifulSoup库来解析HTML文档并提取标签内的字符串。首先,需要安装BeautifulSoup库及其依赖库(如lxml或html.parser)。然后,使用BeautifulSoup
类加载HTML内容,接着通过标签名称找到相应的标签,并使用.string
或.text
属性获取其中的字符串。
使用正则表达式提取标签字符串的最佳实践是什么?
虽然BeautifulSoup是解析HTML的推荐工具,但正则表达式也可以用于简单的字符串提取。使用re
库,可以编写一个匹配特定标签的正则表达式,从而提取标签内的文本。然而,正则表达式对于复杂的HTML结构可能不够稳健,建议在HTML解析时优先考虑使用BeautifulSoup。
是否有其他库可以帮助提取标签字符串?
除了BeautifulSoup,Python中还有其他库可以用于提取标签字符串。例如,lxml提供了高效的XML和HTML解析功能,使用XPath表达式可以方便地查找特定标签及其内容。此外,html5lib也可以解析HTML文档,适用于处理不规范的HTML结构。这些工具各有优缺点,选择时可以根据具体需求和文档结构来决定。
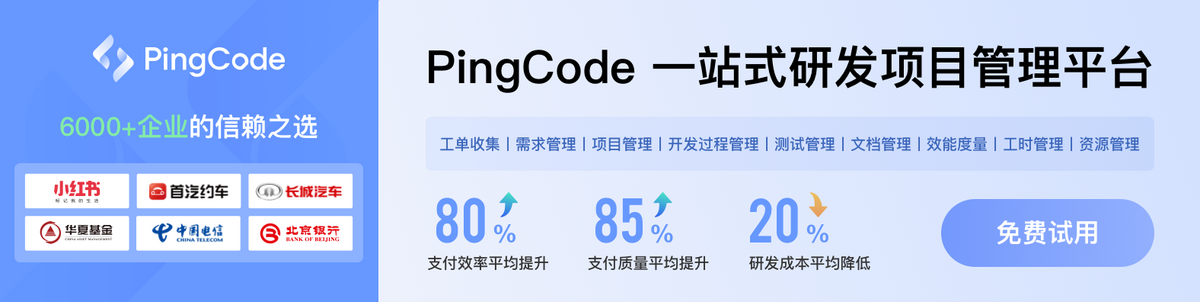