爬取特定标签下一行内容的方法包括使用BeautifulSoup、XPath、正则表达式等技术。 在具体实现中,使用BeautifulSoup解析HTML、选择特定标签、获取标签下一行内容是最常用的方法。下面将详细描述如何通过这些方法实现Python爬取特定标签下一行的内容。
一、安装和引入所需库
要使用Python爬取网页内容,首先需要安装并引入相关的库。常用的库包括requests
、BeautifulSoup
以及lxml
。
# 安装所需库
pip install requests beautifulsoup4 lxml
引入所需库
import requests
from bs4 import BeautifulSoup
二、请求目标网页
使用requests
库获取目标网页的内容。
url = 'http://example.com'
response = requests.get(url)
确保请求成功
if response.status_code == 200:
page_content = response.content
else:
print(f"Failed to retrieve the page. Status code: {response.status_code}")
三、解析HTML内容
使用BeautifulSoup
解析获取的HTML内容。
soup = BeautifulSoup(page_content, 'lxml')
四、选择特定标签
选择需要爬取的特定标签,并获取该标签下一行的内容。
# 假设我们要爬取的是 <div class="target"> 标签下一行的内容
target_div = soup.find('div', class_='target')
if target_div:
# 获取目标标签的下一行内容,假设是紧接着的 <p> 标签
next_sibling = target_div.find_next_sibling('p')
if next_sibling:
print("Content of the next line:", next_sibling.get_text())
else:
print("No next sibling found.")
else:
print("Target div not found.")
五、处理复杂结构
在实际应用中,网页结构可能复杂多变,需要根据具体情况调整爬取方式。
1、处理嵌套结构
如果目标标签嵌套在其他标签中,可以使用多层选择器。
# 选择嵌套结构
nested_div = soup.select_one('div.outer > div.inner > div.target')
if nested_div:
next_sibling = nested_div.find_next_sibling('p')
if next_sibling:
print("Content of the next line:", next_sibling.get_text())
else:
print("No next sibling found.")
else:
print("Nested target div not found.")
2、使用正则表达式
对于复杂的HTML结构或不规则标签,可以结合正则表达式来匹配内容。
import re
使用正则表达式查找目标标签
regex = re.compile(r'<div class="target">(.*?)</div>', re.DOTALL)
match = regex.search(page_content.decode('utf-8'))
if match:
# 获取匹配标签后的内容
following_content = match.group(1).find_next_sibling('p')
print("Content of the next line:", following_content.get_text())
else:
print("No matching content found.")
六、处理动态网页
对于一些动态加载内容的网页,可能需要使用Selenium来模拟浏览器操作。
from selenium import webdriver
from selenium.webdriver.common.by import By
设置浏览器驱动(需要下载浏览器驱动,如ChromeDriver)
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
获取目标标签和其下一行内容
target_element = driver.find_element(By.CLASS_NAME, 'target')
if target_element:
next_sibling = target_element.find_element(By.XPATH, './following-sibling::p[1]')
if next_sibling:
print("Content of the next line:", next_sibling.text)
else:
print("No next sibling found.")
else:
print("Target element not found.")
关闭浏览器
driver.quit()
七、处理异常和边界情况
在实际应用中,处理异常和边界情况非常重要。需要考虑各种可能的错误情况,如标签不存在、网络请求失败等。
try:
response = requests.get(url)
response.raise_for_status()
page_content = response.content
soup = BeautifulSoup(page_content, 'lxml')
target_div = soup.find('div', class_='target')
if target_div:
next_sibling = target_div.find_next_sibling('p')
if next_sibling:
print("Content of the next line:", next_sibling.get_text())
else:
print("No next sibling found.")
else:
print("Target div not found.")
except requests.RequestException as e:
print(f"Request failed: {e}")
except Exception as e:
print(f"An error occurred: {e}")
八、总结
通过以上步骤,可以使用Python有效地爬取网页中特定标签下一行的内容。本文介绍了使用requests
和BeautifulSoup
库进行网页内容请求和解析的基本方法,以及处理嵌套结构、正则表达式、动态网页等复杂情况的技巧。希望这些方法能帮助你在实际项目中更好地处理网页爬取任务。
核心点总结:
- 安装和引入所需库
- 请求目标网页
- 解析HTML内容
- 选择特定标签
- 处理复杂结构
- 处理动态网页
- 处理异常和边界情况
相关问答FAQs:
如何使用Python爬取特定标签的下一行内容?
要实现这一目标,您可以使用BeautifulSoup库进行HTML解析。首先,通过requests库获取网页内容,然后使用BeautifulSoup解析HTML文档,定位到目标标签,接着提取该标签的下一行内容。具体步骤包括发送请求、解析HTML、查找标签及提取相应文本。
爬取网页时,如何处理动态加载的内容?
动态加载的内容通常通过JavaScript生成,您可以使用Selenium库模拟浏览器行为,等待页面加载完毕后再进行内容提取。Selenium可以帮助您处理AJAX请求和JavaScript渲染的内容,从而确保获取到完整的网页数据。
在爬取过程中,如何应对反爬虫机制?
网站通常会采取反爬虫措施来保护数据,您可以通过设置合理的请求头、使用代理IP以及控制爬取速度来减少被检测的风险。此外,随机化请求间隔和使用用户代理字符串也有助于提高爬虫的隐蔽性。
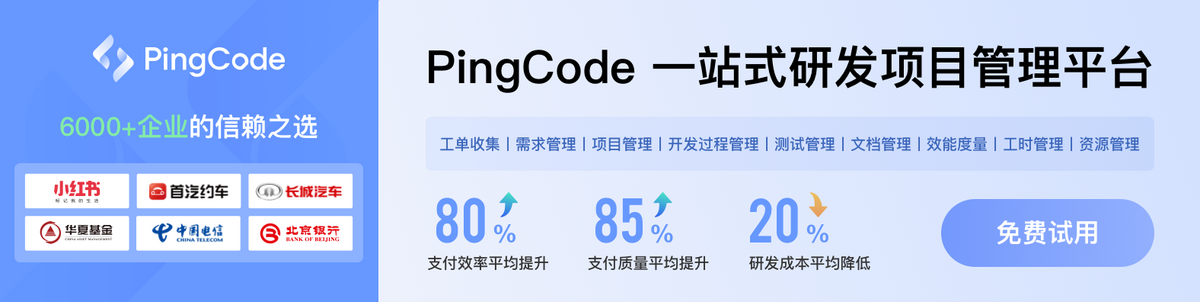