实现摄氏度转华氏度的Python编程的核心在于理解并应用公式、编写函数、处理用户输入。其中最关键的一点是理解公式,摄氏度转华氏度的公式是:华氏度 = 摄氏度 * 9/5 + 32。以下将详细描述如何通过Python编程实现这一过程。
一、理解摄氏度与华氏度转换公式
在开始编写代码之前,首先需要理解摄氏度和华氏度之间的转换关系。摄氏度(Celsius)和华氏度(Fahrenheit)是两种常用的温度计量单位。它们之间的转换公式如下:
[ \text{Fahrenheit} = \text{Celsius} \times \frac{9}{5} + 32 ]
通过这个公式,可以将任意摄氏度温度转换为华氏度温度。接下来,我们将基于这个公式编写Python代码。
二、编写转换函数
首先,我们需要编写一个函数来执行摄氏度到华氏度的转换。这个函数将接收一个摄氏度温度,并返回相应的华氏度温度。
def celsius_to_fahrenheit(celsius):
fahrenheit = celsius * 9/5 + 32
return fahrenheit
这个函数的工作原理非常简单:它接收一个摄氏度温度作为参数,按照公式进行计算,并返回计算结果。
三、处理用户输入
为了使程序更加实用,我们需要添加处理用户输入的功能。用户将输入一个摄氏度温度,程序将调用我们编写的函数进行转换,并输出结果。
def main():
try:
celsius = float(input("请输入摄氏度温度: "))
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"对应的华氏度温度是: {fahrenheit}")
except ValueError:
print("输入无效,请输入一个数字。")
if __name__ == "__main__":
main()
在这个代码段中,我们使用了input()
函数来获取用户输入,并将其转换为浮点数。然后,我们调用celsius_to_fahrenheit
函数进行转换,并输出结果。如果用户输入的不是数字,程序将捕捉到ValueError
并提示用户输入无效。
四、添加更多功能
我们可以进一步增强程序的功能,例如添加单位转换选项、进行批量转换等。
1、添加单位转换选项
有时用户不仅需要摄氏度转华氏度,还可能需要华氏度转摄氏度。我们可以通过添加一个选项来实现这一功能。
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5/9
return celsius
def main():
try:
option = input("请选择转换类型 (1: 摄氏度到华氏度, 2: 华氏度到摄氏度): ")
if option == '1':
celsius = float(input("请输入摄氏度温度: "))
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"对应的华氏度温度是: {fahrenheit}")
elif option == '2':
fahrenheit = float(input("请输入华氏度温度: "))
celsius = fahrenheit_to_celsius(fahrenheit)
print(f"对应的摄氏度温度是: {celsius}")
else:
print("无效的选项,请选择 1 或 2。")
except ValueError:
print("输入无效,请输入一个数字。")
if __name__ == "__main__":
main()
在这个代码段中,我们添加了一个fahrenheit_to_celsius
函数,并在main
函数中添加了选项选择。用户可以选择转换类型,并输入相应的温度值进行转换。
2、进行批量转换
如果需要一次性转换多个温度值,我们可以使用列表来处理批量转换。
def batch_convert(temperatures, conversion_function):
return [conversion_function(temp) for temp in temperatures]
def main():
try:
option = input("请选择转换类型 (1: 摄氏度到华氏度, 2: 华氏度到摄氏度): ")
if option == '1':
temperatures = input("请输入要转换的摄氏度温度列表,用逗号分隔: ").split(',')
temperatures = [float(temp) for temp in temperatures]
converted_temps = batch_convert(temperatures, celsius_to_fahrenheit)
print(f"对应的华氏度温度列表是: {converted_temps}")
elif option == '2':
temperatures = input("请输入要转换的华氏度温度列表,用逗号分隔: ").split(',')
temperatures = [float(temp) for temp in temperatures]
converted_temps = batch_convert(temperatures, fahrenheit_to_celsius)
print(f"对应的摄氏度温度列表是: {converted_temps}")
else:
print("无效的选项,请选择 1 或 2。")
except ValueError:
print("输入无效,请输入一个数字。")
if __name__ == "__main__":
main()
在这个代码段中,我们添加了一个batch_convert
函数来处理批量转换。用户可以输入一个逗号分隔的温度列表,程序将转换每个温度值并输出结果列表。
五、优化用户体验
为了提升用户体验,我们可以添加更多优化和改进措施,如错误处理、单位提示、结果格式化等。
1、改进错误处理
我们可以改进错误处理,使程序更加健壮。
def main():
try:
option = input("请选择转换类型 (1: 摄氏度到华氏度, 2: 华氏度到摄氏度): ")
if option == '1':
temperatures = input("请输入要转换的摄氏度温度列表,用逗号分隔: ").split(',')
temperatures = [float(temp.strip()) for temp in temperatures]
converted_temps = batch_convert(temperatures, celsius_to_fahrenheit)
print(f"对应的华氏度温度列表是: {converted_temps}")
elif option == '2':
temperatures = input("请输入要转换的华氏度温度列表,用逗号分隔: ").split(',')
temperatures = [float(temp.strip()) for temp in temperatures]
converted_temps = batch_convert(temperatures, fahrenheit_to_celsius)
print(f"对应的摄氏度温度列表是: {converted_temps}")
else:
print("无效的选项,请选择 1 或 2。")
except ValueError as e:
print(f"输入无效,请输入一个数字。错误: {e}")
if __name__ == "__main__":
main()
在这个代码段中,我们使用strip()
函数去除输入的多余空格,并捕捉ValueError
异常以提供更详细的错误信息。
2、添加单位提示
在提示用户输入时,我们可以添加单位提示以明确输入要求。
def main():
try:
option = input("请选择转换类型 (1: 摄氏度到华氏度, 2: 华氏度到摄氏度): ")
if option == '1':
temperatures = input("请输入要转换的摄氏度温度列表 (单位: °C),用逗号分隔: ").split(',')
temperatures = [float(temp.strip()) for temp in temperatures]
converted_temps = batch_convert(temperatures, celsius_to_fahrenheit)
print(f"对应的华氏度温度列表 (单位: °F) 是: {converted_temps}")
elif option == '2':
temperatures = input("请输入要转换的华氏度温度列表 (单位: °F),用逗号分隔: ").split(',')
temperatures = [float(temp.strip()) for temp in temperatures]
converted_temps = batch_convert(temperatures, fahrenheit_to_celsius)
print(f"对应的摄氏度温度列表 (单位: °C) 是: {converted_temps}")
else:
print("无效的选项,请选择 1 或 2。")
except ValueError as e:
print(f"输入无效,请输入一个数字。错误: {e}")
if __name__ == "__main__":
main()
在这个代码段中,我们在输入提示中添加了单位信息,使用户明确输入要求,并在输出结果中也包含了单位信息。
3、格式化输出结果
为了提升输出结果的可读性,我们可以对结果进行格式化处理。
def main():
try:
option = input("请选择转换类型 (1: 摄氏度到华氏度, 2: 华氏度到摄氏度): ")
if option == '1':
temperatures = input("请输入要转换的摄氏度温度列表 (单位: °C),用逗号分隔: ").split(',')
temperatures = [float(temp.strip()) for temp in temperatures]
converted_temps = batch_convert(temperatures, celsius_to_fahrenheit)
formatted_temps = [f"{temp:.2f} °F" for temp in converted_temps]
print(f"对应的华氏度温度列表是: {', '.join(formatted_temps)}")
elif option == '2':
temperatures = input("请输入要转换的华氏度温度列表 (单位: °F),用逗号分隔: ").split(',')
temperatures = [float(temp.strip()) for temp in temperatures]
converted_temps = batch_convert(temperatures, fahrenheit_to_celsius)
formatted_temps = [f"{temp:.2f} °C" for temp in converted_temps]
print(f"对应的摄氏度温度列表是: {', '.join(formatted_temps)}")
else:
print("无效的选项,请选择 1 或 2。")
except ValueError as e:
print(f"输入无效,请输入一个数字。错误: {e}")
if __name__ == "__main__":
main()
在这个代码段中,我们使用了Python的字符串格式化功能,将转换结果保留两位小数,并在输出中包含单位信息,从而提升结果的可读性。
通过上述步骤,我们已经详细介绍了如何实现摄氏度转华氏度的Python编程。希望这些内容对您有所帮助。
相关问答FAQs:
如何在Python中实现摄氏度和华氏度的转换?
在Python中,可以通过简单的数学公式实现摄氏度转华氏度的转换。转换公式为:华氏度 = (摄氏度 × 9/5) + 32。你可以使用一个函数来实现这个转换,代码示例如下:
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 9/5) + 32
return fahrenheit
是否可以在Python中处理多个摄氏度值的转换?
当然可以。你可以使用循环来处理多个摄氏度值的转换。在列表中存储摄氏度值,然后逐个调用转换函数。以下是一个示例:
celsius_values = [0, 20, 37, 100]
fahrenheit_values = [celsius_to_fahrenheit(c) for c in celsius_values]
print(fahrenheit_values)
如何在Python中将华氏度转换为摄氏度?
华氏度转摄氏度的转换同样简单。转换公式为:摄氏度 = (华氏度 – 32) × 5/9。你可以创建一个新的函数来实现这个转换,示例代码如下:
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5/9
return celsius
通过这些代码示例,你可以轻松实现摄氏度和华氏度之间的转换。
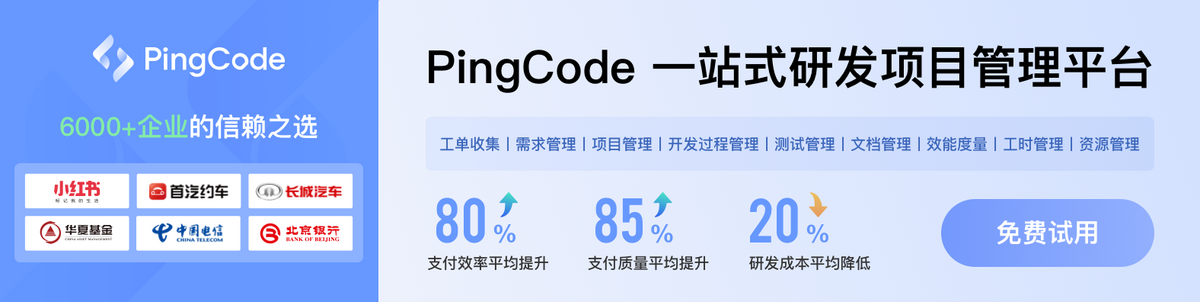