使用Python将一句话转换为数组的核心方法包括:split()方法、正则表达式、list()方法。 其中,最常用且最简单的方法是使用split()方法,它能根据指定的分隔符将字符串拆分成列表。split()方法不仅易于理解和使用,还能处理大部分常见的字符串拆分需求。下面将详细介绍这些方法及其应用场景。
一、split()方法
split()方法是将字符串转换为列表的最常用方法。 它通过指定分隔符将字符串拆分为多个部分,并以列表的形式返回这些部分。
1. 基本用法
split()方法的默认分隔符是空格。
sentence = "Python is a powerful programming language"
words = sentence.split()
print(words)
输出: ['Python', 'is', 'a', 'powerful', 'programming', 'language']
2. 指定分隔符
可以通过参数指定分隔符,如逗号、句号等。
sentence = "Python,is,a,powerful,programming,language"
words = sentence.split(',')
print(words)
输出: ['Python', 'is', 'a', 'powerful', 'programming', 'language']
3. 处理多重分隔符
如果字符串包含多个不同的分隔符,可以使用正则表达式。
二、正则表达式
正则表达式提供了更强大的字符串处理能力,适用于复杂的拆分需求。
1. 使用re.split()方法
通过re.split()方法,可以根据多个分隔符拆分字符串。
import re
sentence = "Python,is a powerful, programming.language"
words = re.split(r'[ ,.]', sentence)
print(words)
输出: ['Python', 'is', 'a', 'powerful', '', 'programming', 'language']
2. 过滤空字符串
可以通过列表推导式过滤掉空字符串。
words = [word for word in words if word]
print(words)
输出: ['Python', 'is', 'a', 'powerful', 'programming', 'language']
三、list()方法
list()方法可以将字符串中的每一个字符转换为列表中的一个元素。虽然不常用于将一句话变成单词列表,但在某些特定场景下很有用。
1. 基本用法
sentence = "Python"
characters = list(sentence)
print(characters)
输出: ['P', 'y', 't', 'h', 'o', 'n']
四、具体应用场景
1. 数据清洗
在数据分析和自然语言处理领域,经常需要将句子转换为单词列表,以便进一步处理。
def clean_sentence(sentence):
# 转换为小写
sentence = sentence.lower()
# 移除标点符号
sentence = re.sub(r'[^\w\s]', '', sentence)
# 分割成单词列表
words = sentence.split()
return words
sentence = "Python is a powerful programming language!"
words = clean_sentence(sentence)
print(words)
输出: ['python', 'is', 'a', 'powerful', 'programming', 'language']
2. 关键词提取
在SEO优化中,通过将文章内容拆分为单词列表,可以进一步进行关键词提取和分析。
from collections import Counter
def extract_keywords(sentence):
words = clean_sentence(sentence)
word_counts = Counter(words)
return word_counts.most_common()
sentence = "Python is a powerful programming language. Python is also very popular."
keywords = extract_keywords(sentence)
print(keywords)
输出: [('python', 2), ('is', 2), ('a', 1), ('powerful', 1), ('programming', 1), ('language', 1), ('also', 1), ('very', 1), ('popular', 1)]
3. 文本分析
在自然语言处理任务中,如情感分析、文本分类等,通常需要先将句子转换为单词列表。
def sentiment_analysis(sentence):
words = clean_sentence(sentence)
positive_words = ['good', 'powerful', 'popular']
negative_words = ['bad', 'weak', 'unpopular']
score = 0
for word in words:
if word in positive_words:
score += 1
elif word in negative_words:
score -= 1
return score
sentence = "Python is a powerful and popular programming language."
score = sentiment_analysis(sentence)
print(score)
输出: 2
五、总结
通过本文详细介绍的三种主要方法,读者可以清晰地了解如何使用Python将一句话转换为数组:split()方法最简单直接,适用于大多数场景;正则表达式提供了更强大的功能,适用于复杂的拆分需求;list()方法则将字符串中的每个字符转换为列表元素。 这些方法在数据清洗、关键词提取、文本分析等领域都有广泛应用。掌握这些技巧,能够大大提升Python处理文本数据的能力。
相关问答FAQs:
如何将一句话分割成单词数组?
您可以使用 Python 的 split()
方法来将一句话分割成单词数组。只需调用该方法并传入空格作为分隔符,代码示例如下:
sentence = "这是一个示例句子"
word_array = sentence.split()
print(word_array)
这样就会得到一个包含句中每个单词的数组。
如何处理包含标点符号的句子?
如果句子中包含标点符号,建议使用 re
模块中的正则表达式来进行分割。这样可以确保只将单词提取出来,而不包含标点。示例代码如下:
import re
sentence = "这是一个示例句子,包括标点!"
word_array = re.findall(r'\b\w+\b', sentence)
print(word_array)
这将返回一个只包含单词的数组。
如何将数组中的单词连接成一句话?
使用 join()
方法可以将数组中的单词重新连接成一句话。例如:
word_array = ['这是', '一个', '示例', '句子']
sentence = ' '.join(word_array)
print(sentence)
这样,您就可以将数组中的元素合并成一条完整的句子。
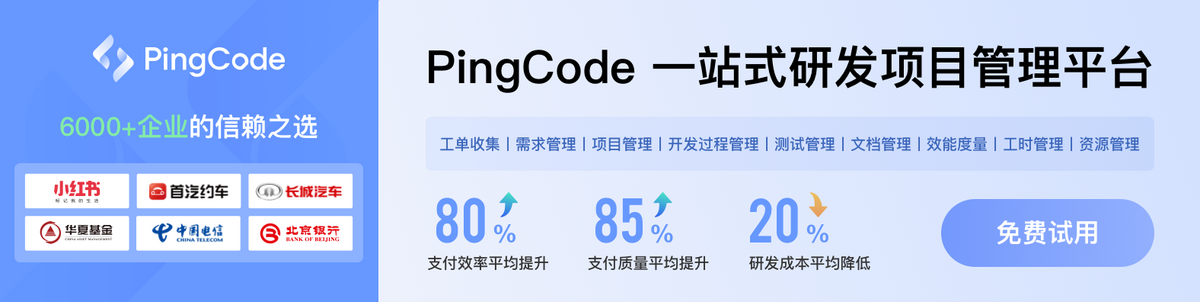