如何将Python对象转换成字符串数组中
Python对象转换为字符串数组可以通过多种方法实现,主要包括使用内置函数str()、repr()、json.dumps()和自定义方法。这些方法各有优缺点,适用不同的场景。最常用的方式是使用内置函数str()进行简单的字符串转换,但在需要保持对象的具体结构和内容时,json.dumps()往往是更好的选择。接下来,我们详细探讨其中一种方法,即使用json.dumps()方法。
json.dumps()方法是Python内置的JSON库中的一个函数,用于将Python对象转换为JSON字符串。这种方法特别适用于需要将复杂数据结构转换为字符串的场景,比如字典、列表等。其优势在于能够保持数据的结构化,方便后续解析和处理。
一、内置函数str()和repr()
Python提供了两个内置函数str()和repr(),用于将对象转换为字符串。
1.1 使用str()函数
str()函数用于将对象转换为人类可读的形式,适用于打印输出等场景。
example_list = [1, 2, 3, 4]
string_representation = str(example_list)
print(string_representation) # 输出: '[1, 2, 3, 4]'
1.2 使用repr()函数
repr()函数用于生成对象的“官方”字符串表示,适用于调试等场景。
example_dict = {"name": "Alice", "age": 25}
repr_representation = repr(example_dict)
print(repr_representation) # 输出: "{'name': 'Alice', 'age': 25}"
二、json.dumps()方法
json.dumps()方法是将Python对象转换为JSON字符串的常用方法,特别适用于复杂数据结构。
2.1 基本用法
import json
example_dict = {"name": "Alice", "age": 25}
json_string = json.dumps(example_dict)
print(json_string) # 输出: '{"name": "Alice", "age": 25}'
2.2 处理复杂数据结构
json.dumps()可以处理列表、字典、嵌套结构等复杂数据。
complex_data = {
"name": "Alice",
"age": 25,
"skills": ["Python", "Machine Learning"],
"projects": [
{"name": "Project A", "status": "Completed"},
{"name": "Project B", "status": "In Progress"}
]
}
json_string = json.dumps(complex_data)
print(json_string) # 输出: '{"name": "Alice", "age": 25, "skills": ["Python", "Machine Learning"], "projects": [{"name": "Project A", "status": "Completed"}, {"name": "Project B", "status": "In Progress"}]}'
三、自定义方法
在某些特殊场景下,可能需要自定义方法来实现对象到字符串数组的转换。
3.1 使用类方法
可以在类中定义一个方法,将对象的属性转换为字符串数组。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def to_string_array(self):
return [str(self.name), str(self.age)]
person = Person("Alice", 25)
string_array = person.to_string_array()
print(string_array) # 输出: ['Alice', '25']
3.2 使用递归方法
对于复杂嵌套数据结构,可以使用递归方法进行转换。
def recursive_to_string_array(data):
if isinstance(data, dict):
return {k: recursive_to_string_array(v) for k, v in data.items()}
elif isinstance(data, list):
return [recursive_to_string_array(i) for i in data]
else:
return str(data)
complex_data = {
"name": "Alice",
"age": 25,
"skills": ["Python", "Machine Learning"],
"projects": [
{"name": "Project A", "status": "Completed"},
{"name": "Project B", "status": "In Progress"}
]
}
string_array = recursive_to_string_array(complex_data)
print(string_array)
四、结合使用多种方法
在实际开发中,可能需要结合多种方法来实现对象到字符串数组的转换,以满足不同的需求。
4.1 结合str()和json.dumps()
可以先使用str()将对象的简单属性转换为字符串,再使用json.dumps()处理复杂结构。
class Person:
def __init__(self, name, age, skills):
self.name = name
self.age = age
self.skills = skills
def to_string_array(self):
basic_info = [str(self.name), str(self.age)]
skills_json = json.dumps(self.skills)
return basic_info + [skills_json]
person = Person("Alice", 25, ["Python", "Machine Learning"])
string_array = person.to_string_array()
print(string_array) # 输出: ['Alice', '25', '["Python", "Machine Learning"]']
五、处理特殊数据类型
在某些情况下,可能需要处理特殊数据类型,如自定义对象、日期时间等。
5.1 自定义对象
对于自定义对象,可以在类中实现__str__或__repr__方法。
class CustomObject:
def __init__(self, attribute):
self.attribute = attribute
def __str__(self):
return f"CustomObject with attribute: {self.attribute}"
custom_obj = CustomObject("example")
string_representation = str(custom_obj)
print(string_representation) # 输出: CustomObject with attribute: example
5.2 日期时间
对于日期时间,可以使用datetime模块中的方法进行转换。
from datetime import datetime
current_time = datetime.now()
string_representation = current_time.strftime("%Y-%m-%d %H:%M:%S")
print(string_representation) # 输出: '2023-01-01 12:00:00'
六、总结
将Python对象转换为字符串数组是一个常见的需求,可以通过多种方法实现,包括内置函数str()、repr()、json.dumps()和自定义方法。不同的方法适用于不同的场景,开发者需要根据实际需求选择合适的方法。通过本文的详细介绍,相信读者可以更好地理解和应用这些方法,实现Python对象到字符串数组的高效转换。
相关问答FAQs:
如何将Python中的对象转换为字符串形式?
在Python中,可以使用str()
函数或repr()
函数将对象转换为字符串。这两种方法都会返回对象的字符串表示,但repr()
通常用于调试,它返回的信息更为详细。对于自定义对象,建议在类中实现__str__()
和__repr__()
方法,以便控制对象转换成字符串时的表现。
Python中有哪些方法可以将对象转换成数组?
可以使用列表推导式或map()
函数将多个对象转换为字符串数组。例如,假设你有一个对象列表,可以通过[str(obj) for obj in object_list]
或list(map(str, object_list))
将其转换为字符串数组。这种方法简单且高效。
如何处理复杂对象的转换?
对于复杂对象,如字典或自定义类,可以使用json
模块将其转换为字符串。通过json.dumps()
方法,你可以将Python对象转换为JSON格式的字符串。要将其转换为字符串数组,先将对象转换为字符串,然后使用split()
方法将其分割成数组。例如:json_str = json.dumps(complex_object)
,再通过string_array = json_str.split()
进行处理。
是否有库可以帮助对象转换?
确实存在一些第三方库,例如pandas
和numpy
,可以将Python对象(尤其是数据结构)转换为数组或数据框。这些库提供了强大的功能,可以方便地处理和转换数据。例如,使用pandas.DataFrame
可以轻松将字典或列表转换为数据框,再通过to_numpy()
方法获取数组形式。
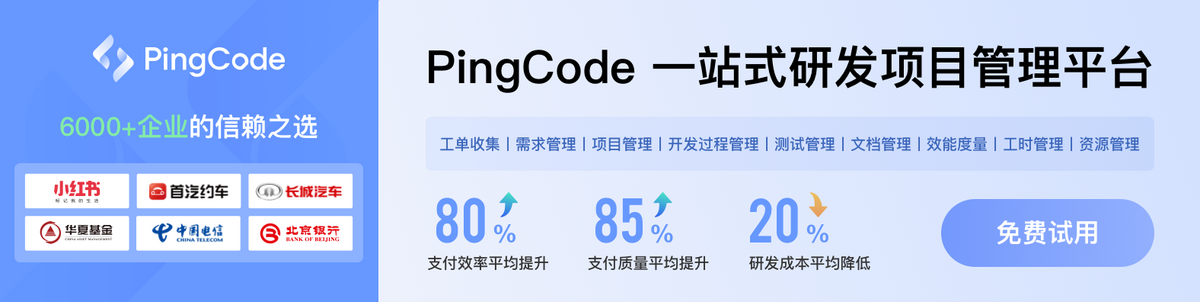