Python如何筛选字符串中指定的字符串
在Python中,筛选字符串中的指定字符串可以通过使用内置方法如find()
、in
关键字、正则表达式等方式来实现。其中,正则表达式提供了更强大的匹配和筛选能力,可以处理更复杂的字符串模式。接下来我们将详细讨论这些方法,并给出具体的代码示例和使用场景。
一、使用 find()
方法
find()
方法用于在字符串中查找指定子字符串,如果找到则返回子字符串的起始索引,否则返回 -1。这个方法适用于简单的字符串查找。
示例代码:
text = "Python is a powerful programming language."
substring = "powerful"
index = text.find(substring)
if index != -1:
print(f"Found '{substring}' at index {index}")
else:
print(f"'{substring}' not found in the text.")
二、使用 in
关键字
in
关键字用于检查子字符串是否存在于字符串中,返回布尔值。这种方法简洁易懂,适用于简单的包含检查。
示例代码:
text = "Python is a powerful programming language."
substring = "powerful"
if substring in text:
print(f"'{substring}' is in the text.")
else:
print(f"'{substring}' is not in the text.")
三、使用正则表达式
正则表达式提供了更强大的字符串匹配和筛选功能,适用于复杂的字符串模式匹配。Python 的 re
模块可以实现正则表达式的匹配、查找、替换等功能。
示例代码:
import re
text = "Python is a powerful programming language."
pattern = "powerful"
match = re.search(pattern, text)
if match:
print(f"Found '{pattern}' at index {match.start()}")
else:
print(f"'{pattern}' not found in the text.")
四、分割字符串并筛选
通过分割字符串并遍历每个部分,可以实现更复杂的筛选逻辑。例如,可以按空格分割字符串,并检查每个单词是否包含指定子字符串。
示例代码:
text = "Python is a powerful programming language."
substring = "power"
words = text.split()
filtered_words = [word for word in words if substring in word]
if filtered_words:
print(f"Words containing '{substring}': {filtered_words}")
else:
print(f"No words contain '{substring}' in the text.")
五、使用字符串方法 startswith()
和 endswith()
如果需要检查字符串是否以指定子字符串开头或结尾,可以使用 startswith()
和 endswith()
方法。
示例代码:
text = "Python is a powerful programming language."
prefix = "Python"
suffix = "language."
if text.startswith(prefix):
print(f"Text starts with '{prefix}'")
else:
print(f"Text does not start with '{prefix}'")
if text.endswith(suffix):
print(f"Text ends with '{suffix}'")
else:
print(f"Text does not end with '{suffix}'")
六、使用 count()
方法
count()
方法用于统计子字符串在字符串中出现的次数,这对于需要频率统计的场景非常有用。
示例代码:
text = "Python is a powerful programming language. Python is also versatile."
substring = "Python"
count = text.count(substring)
print(f"'{substring}' appears {count} times in the text.")
七、结合多种方法实现复杂筛选
在实际应用中,可能需要结合多种方法来实现复杂的字符串筛选和处理。例如,可以先通过正则表达式匹配,然后使用字符串方法进行进一步处理。
示例代码:
import re
text = "Python is a powerful programming language. Python is also versatile."
pattern = r"\bPython\b"
matches = re.finditer(pattern, text)
for match in matches:
start_index = match.start()
end_index = match.end()
word = text[start_index:end_index]
print(f"Found '{word}' at index range ({start_index}, {end_index})")
八、处理特殊字符和转义字符
在处理包含特殊字符的字符串时,需要使用转义字符。例如,处理包含引号或反斜杠的字符串时,需要使用反斜杠进行转义。
示例代码:
text = 'She said, "Python is great!"'
substring = '"Python'
index = text.find(substring)
if index != -1:
print(f"Found '{substring}' at index {index}")
else:
print(f"'{substring}' not found in the text.")
九、筛选多种子字符串
在一些场景下,可能需要筛选多个子字符串。可以通过循环或列表推导式实现。
示例代码:
text = "Python is a powerful programming language. Python is also versatile."
substrings = ["powerful", "versatile"]
found_substrings = [substring for substring in substrings if substring in text]
if found_substrings:
print(f"Found substrings: {found_substrings}")
else:
print("No specified substrings found in the text.")
十、性能优化和注意事项
在处理大文本时,需要关注性能问题。可以通过分块处理、异步处理等方式优化性能。此外,使用正则表达式时需要注意避免过于复杂的模式匹配,以免影响性能。
示例代码:
import re
def process_text_in_chunks(text, pattern, chunk_size=1024):
start = 0
while start < len(text):
end = min(start + chunk_size, len(text))
chunk = text[start:end]
match = re.search(pattern, chunk)
if match:
print(f"Found '{pattern}' in chunk: {chunk}")
start = end
text = "Python is a powerful programming language. Python is also versatile." * 1000
pattern = "Python"
process_text_in_chunks(text, pattern)
总结
筛选字符串中的指定子字符串是Python编程中常见的任务。通过使用内置方法(如find()
、in
关键字)、正则表达式、字符串分割和筛选、前缀和后缀检查等方法,可以实现不同场景下的字符串筛选需求。在实际应用中,可以结合多种方法,并关注性能优化和特殊字符处理,以实现更加高效和可靠的字符串筛选。
相关问答FAQs:
如何在Python中检查字符串是否包含指定的子字符串?
在Python中,可以使用in
运算符来检查一个字符串是否包含另一个字符串。例如,if "指定字符串" in "待检查的字符串":
这种方式简单易懂,能够有效判断一个子字符串是否存在于另一个字符串中。
Python中有哪些方法可以提取字符串中的特定内容?
可以使用正则表达式模块re
来提取字符串中的特定内容。通过re.findall()
函数,可以根据模式匹配提取所有符合条件的子字符串。例如,re.findall(r"指定的模式", "待提取的字符串")
将返回所有匹配的结果,适合复杂的字符串匹配需求。
如何在Python中删除字符串中的指定部分?
可以使用str.replace()
方法来删除字符串中的指定部分。例如,"原字符串".replace("要删除的部分", "")
将返回一个新的字符串,去除了所有指定的部分。这种方法简单且高效,适合进行字符串的快速处理。
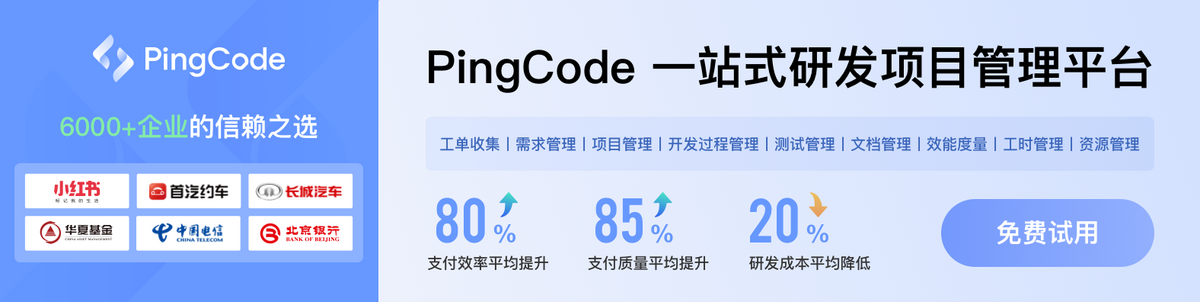