在Python中,表示一个字符串的方式有多种,常见的有单引号、双引号、三重引号等方式。 使用单引号和双引号都可以创建简单的字符串,而三重引号通常用于跨多行的字符串或文档字符串。详细描述如下:
-
单引号和双引号:单引号(')和双引号(")的使用非常灵活,可以创建简单的字符串。例如:'hello'、"world"。Python允许在字符串中包含引号的另一种类型,例如,字符串中包含单引号时,可以使用双引号来包围它,反之亦然。
-
三重引号:三重引号('''或""")用于创建多行字符串或文档字符串(docstring)。例如:'''This is a multi-line string'''、"""This is also a multi-line string"""。它们还可以包含内部的单引号和双引号,而无需使用转义字符。
一、字符串的基本表示方法
1. 单引号和双引号
在Python中,单引号和双引号的使用没有区别,都是用于创建字符串对象。一个字符串可以通过以下方式创建:
single_quote_string = 'Hello, World!'
double_quote_string = "Hello, World!"
2. 转义字符
如果字符串中包含引号,或者需要在字符串中使用特殊字符,可以使用反斜杠(\)作为转义字符。例如:
quote_in_string = 'He said, "Hello!"'
escape_single_quote = 'It\'s a sunny day'
在这些例子中,双引号被包含在单引号字符串中,反之亦然,另外通过使用反斜杠转义单引号避免了语法错误。
二、三重引号的使用
三重引号允许字符串跨多行,并且可以包含单引号和双引号而无需转义。这在编写文档字符串(docstring)时特别有用:
multi_line_string = """This is a multi-line string.
It can span multiple lines.
It can also contain 'single quotes' and "double quotes" without escaping."""
三、字符串的常见操作
1. 拼接字符串
字符串可以通过加号(+)进行拼接:
string1 = "Hello"
string2 = "World"
combined_string = string1 + ", " + string2 + "!"
print(combined_string) # Output: Hello, World!
2. 重复字符串
可以使用乘法运算符(*)重复字符串:
repeat_string = "Ha" * 3
print(repeat_string) # Output: HaHaHa
3. 字符串切片
字符串是不可变的序列,可以使用切片操作获取子字符串:
sample_string = "Hello, World!"
substring = sample_string[7:12]
print(substring) # Output: World
4. 字符串方法
Python提供了丰富的字符串方法,用于各种操作:
upper_string = "hello".upper() # Output: 'HELLO'
lower_string = "WORLD".lower() # Output: 'world'
capitalized_string = "python".capitalize() # Output: 'Python'
四、格式化字符串
1. 使用百分号(%)格式化
name = "John"
age = 30
formatted_string = "My name is %s and I am %d years old." % (name, age)
print(formatted_string) # Output: My name is John and I am 30 years old.
2. 使用str.format()
formatted_string = "My name is {} and I am {} years old.".format(name, age)
print(formatted_string) # Output: My name is John and I am 30 years old.
3. 使用f-strings(Python 3.6+)
formatted_string = f"My name is {name} and I am {age} years old."
print(formatted_string) # Output: My name is John and I am 30 years old.
五、字符串的编码和解码
在Python中,字符串是Unicode字符的序列。可以使用encode()
方法将字符串转换为字节对象(bytes),使用decode()
将字节对象转换为字符串:
unicode_string = "Hello, World!"
encoded_string = unicode_string.encode("utf-8")
print(encoded_string) # Output: b'Hello, World!'
decoded_string = encoded_string.decode("utf-8")
print(decoded_string) # Output: Hello, World!
六、字符串的常见问题和解决方案
1. 如何处理包含特殊字符的字符串?
可以使用转义字符或三重引号来处理包含特殊字符的字符串:
special_char_string = "This is a string with a newline character.\nSee?"
print(special_char_string)
2. 如何检查字符串是否包含子字符串?
可以使用in
关键字:
main_string = "Hello, World!"
substring = "World"
if substring in main_string:
print(f"The string '{main_string}' contains '{substring}'")
七、总结
通过了解和掌握Python中字符串的表示方法和操作,可以更有效地处理文本数据。单引号、双引号、三重引号、转义字符、字符串拼接、字符串切片、字符串方法、字符串格式化、字符串编码和解码等基本操作都是开发过程中常用的技能。通过深入理解这些概念和技巧,可以提升编程效率和代码质量。
相关问答FAQs:
如何在Python中创建一个字符串?
在Python中,字符串可以通过将字符放在单引号(')或双引号(")之间来创建。例如,my_string = 'Hello, World!'
或 my_string = "Hello, World!"
。无论是使用单引号还是双引号,效果是相同的,选择哪种形式主要取决于个人习惯或字符串内容。
Python字符串支持哪些操作?
Python字符串支持多种操作,包括拼接、切片、查找、替换和格式化等。拼接可以通过使用加号(+)实现,例如 str1 + str2
。切片可以通过索引访问字符串的特定部分,例如 my_string[0:5]
将返回 'Hello'。此外,Python还提供了许多内置方法,如 find()
、replace()
和 upper()
,以便于对字符串进行处理。
如何在Python中处理多行字符串?
在Python中,可以使用三重引号('''或""")来创建多行字符串。这种方法允许字符串跨越多行,而不需要使用换行符。例如:
multi_line_string = """这是第一行
这是第二行
这是第三行"""
使用三重引号时,字符串内部的换行会被保留,使得在处理多行文本时更加方便和直观。
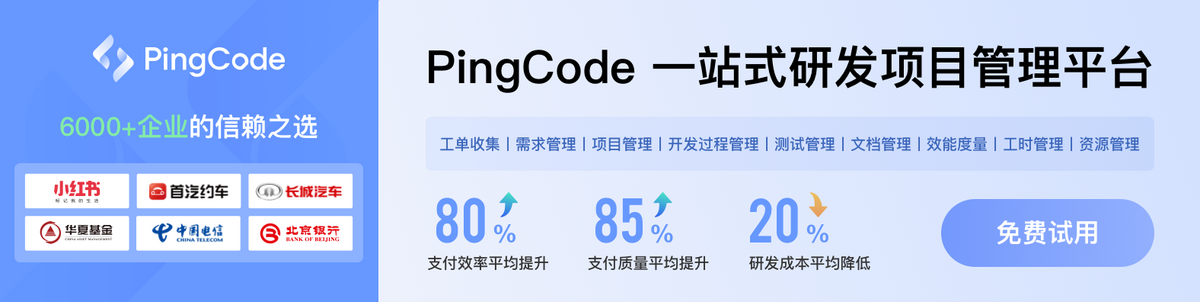