Python保留小数点后两位的方法有多种,包括使用字符串格式化、round函数、Decimal模块等。其中,字符串格式化 是最常用且易于理解的方法。通过使用格式化字符串,您可以轻松地指定小数点后保留的位数,例如 "{:.2f}".format(3.14159)
将输出 3.14
。为了让大家更全面了解各种方法,下面将详细介绍几种常见的实现方式及其具体应用场景。
一、字符串格式化
字符串格式化是Python中最常用的方法之一,能够灵活地控制输出格式。
1.1 使用 format()
函数
format()
函数可以用于格式化字符串,指定小数点后的位数。例如:
number = 3.14159
formatted_number = "{:.2f}".format(number)
print(formatted_number) # 输出: 3.14
这种方法的好处是简洁明了,容易理解且使用广泛。
1.2 使用 f-string(Python 3.6+)
Python 3.6之后引入了f-string(格式化字符串),使得格式化更加直观和简洁:
number = 3.14159
formatted_number = f"{number:.2f}"
print(formatted_number) # 输出: 3.14
f-string不仅格式化简便,还支持嵌入表达式和变量,使代码更加清晰可读。
二、使用 round()
函数
round()
函数用于将数字四舍五入到指定的小数位数:
number = 3.14159
rounded_number = round(number, 2)
print(rounded_number) # 输出: 3.14
需要注意的是,round()
函数返回的是浮点数,而不是字符串。如果需要字符串格式,可以结合 str()
函数使用:
rounded_number = round(number, 2)
formatted_number = str(rounded_number)
print(formatted_number) # 输出: 3.14
三、使用 Decimal
模块
Decimal
模块提供了高精度的浮点运算,适用于对精度要求较高的场景:
from decimal import Decimal, ROUND_HALF_UP
number = Decimal('3.14159')
rounded_number = number.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
print(rounded_number) # 输出: 3.14
这种方法的优点是精度高,适用于金融计算等对精度要求较高的场景。
四、结合 math
模块
math
模块也可以用于数值处理,不过在保留小数点后两位的场景中较少使用。以下是一个结合 math
模块和其他方法的例子:
import math
number = 3.14159
rounded_number = round(number, 2)
formatted_number = "{:.2f}".format(rounded_number)
print(formatted_number) # 输出: 3.14
这种方法展示了如何将不同的方法结合使用,以便在特定的应用场景中达到最佳效果。
五、应用场景和注意事项
5.1 金融计算
在金融计算中,精度至关重要。因此,推荐使用 Decimal
模块:
from decimal import Decimal, ROUND_HALF_UP
amount = Decimal('1234.5678')
formatted_amount = amount.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
print(formatted_amount) # 输出: 1234.57
5.2 科学计算
在科学计算中,常常需要保留一定的精度。可以使用 format()
函数或 round()
函数:
value = 1.23456789
formatted_value = "{:.2f}".format(value)
print(formatted_value) # 输出: 1.23
5.3 数据展示
在数据展示中,使用 format()
函数或 f-string 可以生成格式化的字符串,便于展示:
value = 98.7654321
formatted_value = f"{value:.2f}"
print(f"The formatted value is: {formatted_value}") # 输出: The formatted value is: 98.77
总结
Python提供了多种方法来保留小数点后两位,包括字符串格式化、round()
函数和 Decimal
模块等。字符串格式化 是最常用的方法,round()
函数 简洁易用,而 Decimal
模块 则提供了高精度的计算。根据具体的应用场景选择合适的方法,可以有效地提升代码的可靠性和可读性。
通过灵活运用这些方法,您可以在各种场景中精确地控制数字的显示格式,满足不同需求。
相关问答FAQs:
如何在Python中格式化浮点数以保留两位小数?
在Python中,可以使用内置的format()
函数或f-string语法来格式化浮点数。例如,使用format()
函数可以这样写:formatted_number = "{:.2f}".format(your_number)
,而在f-string中,可以这样写:formatted_number = f"{your_number:.2f}"
。这两种方法都能有效保留小数点后两位。
Python中是否有其他方法来控制小数位数?
除了使用format()
函数和f-string,Python还提供了round()
函数来控制小数位数。使用方法为rounded_number = round(your_number, 2)
,这会将数字四舍五入到小数点后两位。需要注意的是,round()
函数在某些情况下可能会返回整数。
在数据框中如何保留小数点后两位?
对于使用Pandas库的数据框,可以通过DataFrame.round()
方法来保留小数点后两位。示例代码为:df['column_name'] = df['column_name'].round(2)
。这将对指定列的所有值进行四舍五入并保留两位小数,方便数据分析和展示。
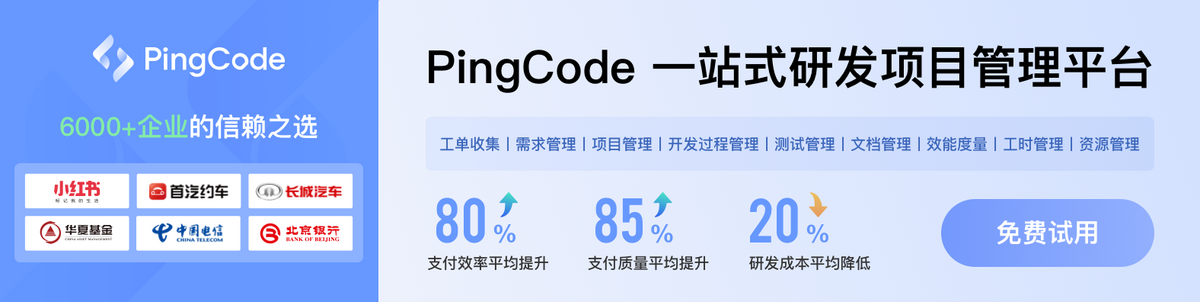