在Python中,获取字符串的第二个值可以通过索引操作来实现。字符串在Python中是一个序列,通过索引可以访问其每一个字符。Python的索引从0开始,因此字符串的第二个字符的索引是1。下面将详细介绍这一点,并探讨字符串操作的更多方面。
一、字符串索引操作
字符串索引是通过方括号[]
来实现的。假设有一个字符串str
,要获取其第二个字符,可以使用str[1]
。例如:
str = "hello"
second_char = str[1]
print(second_char) # 输出 'e'
在这个例子中,字符串"hello"
的第二个字符是"e"
,通过str[1]
成功获取。
二、字符串的不可变性
Python中的字符串是不可变的,这意味着一旦创建,就无法更改其内容。每次对字符串进行操作时,都会创建一个新的字符串对象。因此,不能直接通过索引修改字符串中的字符。例如:
str = "hello"
str[1] = 'a' # 这将引发错误
如果需要更改字符串,可以使用字符串切片和连接操作来实现。例如:
str = "hello"
new_str = str[:1] + 'a' + str[2:]
print(new_str) # 输出 'hallo'
在这个例子中,使用字符串切片和连接操作,将字符串的第二个字符修改为'a'
。
三、字符串切片操作
字符串切片允许你获取字符串的子字符串。切片的基本语法是str[start:stop:step]
,其中start
是起始索引,stop
是结束索引,step
是步长。例如:
str = "hello"
sub_str = str[1:4]
print(sub_str) # 输出 'ell'
在这个例子中,str[1:4]
获取从索引1开始到索引4之前的子字符串。
四、字符串常用操作
- 字符串拼接
字符串拼接可以使用加号+
操作符。例如:
str1 = "hello"
str2 = "world"
result = str1 + " " + str2
print(result) # 输出 'hello world'
- 字符串格式化
Python提供了多种字符串格式化的方法,包括%
操作符、str.format()
方法和f字符串。例如:
name = "Alice"
age = 30
formatted_str = "Name: %s, Age: %d" % (name, age)
print(formatted_str) # 输出 'Name: Alice, Age: 30'
formatted_str = "Name: {}, Age: {}".format(name, age)
print(formatted_str) # 输出 'Name: Alice, Age: 30'
formatted_str = f"Name: {name}, Age: {age}"
print(formatted_str) # 输出 'Name: Alice, Age: 30'
- 字符串查找
可以使用find()
方法查找子字符串在字符串中的位置。例如:
str = "hello world"
index = str.find("world")
print(index) # 输出 6
- 字符串替换
可以使用replace()
方法替换字符串中的子字符串。例如:
str = "hello world"
new_str = str.replace("world", "Python")
print(new_str) # 输出 'hello Python'
五、字符串的高级操作
- 正则表达式
Python的re
模块提供了强大的字符串匹配和替换功能。使用正则表达式,可以进行复杂的字符串操作。例如:
import re
str = "hello world"
pattern = r"world"
replacement = "Python"
new_str = re.sub(pattern, replacement, str)
print(new_str) # 输出 'hello Python'
- 字符串的编码和解码
在处理不同编码的字符串时,可以使用encode()
和decode()
方法。例如:
str = "你好"
encoded_str = str.encode('utf-8')
print(encoded_str) # 输出 b'\xe4\xbd\xa0\xe5\xa5\xbd'
decoded_str = encoded_str.decode('utf-8')
print(decoded_str) # 输出 '你好'
- 字符串的分割和连接
可以使用split()
方法将字符串分割为列表,使用join()
方法将列表连接为字符串。例如:
str = "hello world"
words = str.split()
print(words) # 输出 ['hello', 'world']
joined_str = " ".join(words)
print(joined_str) # 输出 'hello world'
六、字符串的性能优化
在进行大量字符串拼接时,使用加号+
操作符会创建多个中间字符串,导致性能下降。可以使用列表和join()
方法来优化。例如:
words = ["hello", "world", "this", "is", "Python"]
result = " ".join(words)
print(result) # 输出 'hello world this is Python'
通过使用join()
方法,可以避免创建多个中间字符串,从而提高性能。
七、总结
在Python中,字符串是一个强大且灵活的数据类型。通过索引、切片和各种字符串操作方法,可以轻松地处理和操作字符串。了解这些基本和高级操作,可以帮助你在编写Python代码时更加高效和灵活地处理字符串。
相关问答FAQs:
如何在Python中获取字符串的第二个字符?
在Python中,可以通过索引来获取字符串中的特定字符。字符串的索引是从0开始的,因此第二个字符的索引是1。可以使用以下代码来获取第二个字符:
my_string = "Hello"
second_char = my_string[1]
print(second_char) # 输出 'e'
这个方法简单且高效,适用于任何长度的字符串。
如何在Python中从字符串中提取第二个单词?
要提取字符串中的第二个单词,可以使用split()
方法将字符串分割成单词列表,然后访问列表中的第二个元素。示例如下:
my_string = "Hello world from Python"
words = my_string.split()
second_word = words[1]
print(second_word) # 输出 'world'
这种方式对于处理空格分隔的字符串非常有效。
如何在Python中获取字符串中第二个出现的特定字符的位置?
如果想找到字符串中某个特定字符第二次出现的位置,可以使用find()
或index()
方法结合循环来实现。示例如下:
my_string = "banana"
char_to_find = 'a'
first_index = my_string.find(char_to_find)
second_index = my_string.find(char_to_find, first_index + 1)
print(second_index) # 输出 3
这种方法可以帮助你精确定位字符在字符串中的位置。
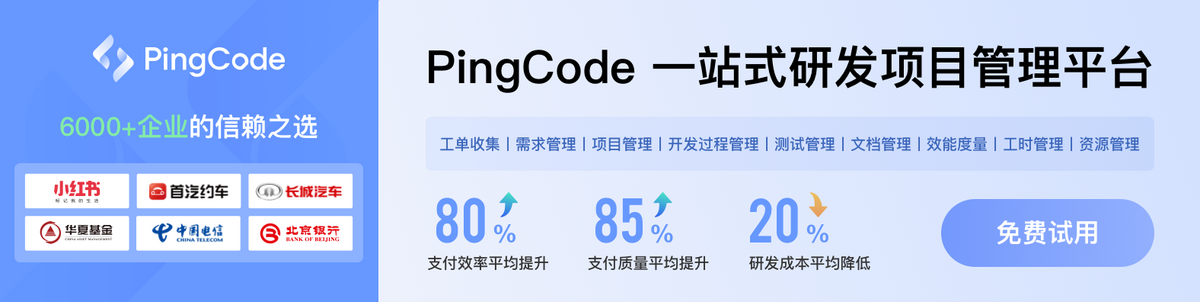