如何在Python中将两张图像合并在一起:使用Pillow和OpenCV
在Python中,合并图像的方法有很多,常用的库包括Pillow、OpenCV、NumPy。这些库提供了丰富的图像处理功能,可以帮助我们轻松地将两张图像合并。本文将详细介绍这几种方法,并通过具体的代码示例来展示如何实现图像合并。以下是使用Pillow库的具体步骤。
Pillow库
Pillow是Python Imaging Library(PIL)的一个分支和升级版本,支持多种格式的图像文件,并提供了强大的图像处理功能。以下是使用Pillow合并图像的步骤:
-
安装Pillow库:
pip install pillow
-
读取图像并合并:
from PIL import Image
打开两张图像
image1 = Image.open('path_to_image1.jpg')
image2 = Image.open('path_to_image2.jpg')
获取图像尺寸
width1, height1 = image1.size
width2, height2 = image2.size
创建一个新的图像,其尺寸为两张图像宽度之和,高度为两张图像的最大高度
new_image = Image.new('RGB', (width1 + width2, max(height1, height2)))
将两张图像粘贴到新图像上
new_image.paste(image1, (0, 0))
new_image.paste(image2, (width1, 0))
保存合并后的图像
new_image.save('merged_image.jpg')
详细描述:
在上面的代码中,我们首先使用Image.open()
方法打开两张图像,然后获取它们的尺寸。接着,我们创建一个新的图像,其尺寸为两张图像的宽度之和,高度为两张图像的最大高度。通过Image.new()
方法创建一个新的空白图像,并使用paste()
方法将两张图像粘贴到新图像上,最终保存合并后的图像。
一、Pillow库的详细介绍及使用
1、安装和基本使用
Pillow库是一个强大的图像处理库,它不仅支持多种格式的图像文件,还提供了许多图像处理功能。首先,我们需要安装Pillow库,可以通过以下命令进行安装:
pip install pillow
安装完成后,我们可以使用Pillow库来打开、显示、保存和处理图像。以下是一些基本的图像操作:
from PIL import Image
打开图像
image = Image.open('path_to_image.jpg')
显示图像
image.show()
保存图像
image.save('new_image.jpg')
获取图像尺寸
width, height = image.size
print(f'宽度: {width}, 高度: {height}')
2、合并图像的具体步骤
通过Pillow库,我们可以轻松地将两张图像合并在一起。以下是具体的步骤:
- 打开图像:使用
Image.open()
方法打开两张图像。 - 获取图像尺寸:使用
image.size
获取图像的宽度和高度。 - 创建新图像:使用
Image.new()
方法创建一个新的空白图像,其尺寸为两张图像宽度之和,高度为两张图像的最大高度。 - 粘贴图像:使用
paste()
方法将两张图像粘贴到新图像上。 - 保存图像:使用
save()
方法保存合并后的图像。
以下是详细的代码示例:
from PIL import Image
def merge_images(image1_path, image2_path, output_path):
# 打开两张图像
image1 = Image.open(image1_path)
image2 = Image.open(image2_path)
# 获取图像尺寸
width1, height1 = image1.size
width2, height2 = image2.size
# 创建一个新的图像,其尺寸为两张图像宽度之和,高度为两张图像的最大高度
new_image = Image.new('RGB', (width1 + width2, max(height1, height2)))
# 将两张图像粘贴到新图像上
new_image.paste(image1, (0, 0))
new_image.paste(image2, (width1, 0))
# 保存合并后的图像
new_image.save(output_path)
测试合并图像
merge_images('path_to_image1.jpg', 'path_to_image2.jpg', 'merged_image.jpg')
二、使用OpenCV库合并图像
OpenCV是一个开源的计算机视觉和图像处理库,提供了丰富的图像处理功能。相比Pillow,OpenCV更加侧重于图像处理和计算机视觉领域。以下是使用OpenCV合并图像的步骤:
1、安装和基本使用
首先,我们需要安装OpenCV库,可以通过以下命令进行安装:
pip install opencv-python
安装完成后,我们可以使用OpenCV库来读取、显示和处理图像。以下是一些基本的图像操作:
import cv2
读取图像
image = cv2.imread('path_to_image.jpg')
显示图像
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
保存图像
cv2.imwrite('new_image.jpg', image)
获取图像尺寸
height, width, channels = image.shape
print(f'宽度: {width}, 高度: {height}, 通道数: {channels}')
2、合并图像的具体步骤
通过OpenCV库,我们可以轻松地将两张图像合并在一起。以下是具体的步骤:
- 读取图像:使用
cv2.imread()
方法读取两张图像。 - 获取图像尺寸:使用
image.shape
获取图像的宽度和高度。 - 创建新图像:使用
numpy.zeros()
方法创建一个新的空白图像,其尺寸为两张图像宽度之和,高度为两张图像的最大高度。 - 粘贴图像:使用数组切片将两张图像粘贴到新图像上。
- 保存图像:使用
cv2.imwrite()
方法保存合并后的图像。
以下是详细的代码示例:
import cv2
import numpy as np
def merge_images(image1_path, image2_path, output_path):
# 读取两张图像
image1 = cv2.imread(image1_path)
image2 = cv2.imread(image2_path)
# 获取图像尺寸
height1, width1, channels1 = image1.shape
height2, width2, channels2 = image2.shape
# 创建一个新的图像,其尺寸为两张图像宽度之和,高度为两张图像的最大高度
new_image = np.zeros((max(height1, height2), width1 + width2, 3), dtype=np.uint8)
# 将两张图像粘贴到新图像上
new_image[0:height1, 0:width1] = image1
new_image[0:height2, width1:width1 + width2] = image2
# 保存合并后的图像
cv2.imwrite(output_path, new_image)
测试合并图像
merge_images('path_to_image1.jpg', 'path_to_image2.jpg', 'merged_image.jpg')
三、使用NumPy库合并图像
NumPy是一个用于科学计算的库,提供了多维数组对象和各种操作函数。虽然NumPy本身不是一个图像处理库,但我们可以结合Pillow或OpenCV来处理图像数据。以下是使用NumPy合并图像的步骤:
1、安装和基本使用
首先,我们需要安装NumPy库,可以通过以下命令进行安装:
pip install numpy
安装完成后,我们可以使用NumPy库来创建和操作多维数组。以下是一些基本的数组操作:
import numpy as np
创建一个数组
array = np.array([1, 2, 3, 4, 5])
打印数组
print(array)
创建一个二维数组
array_2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(array_2d)
获取数组形状
shape = array_2d.shape
print(f'形状: {shape}')
2、结合Pillow合并图像
我们可以使用Pillow库读取图像,然后使用NumPy库将图像数据转换为数组进行操作。以下是详细的代码示例:
from PIL import Image
import numpy as np
def merge_images(image1_path, image2_path, output_path):
# 打开两张图像
image1 = Image.open(image1_path)
image2 = Image.open(image2_path)
# 将图像转换为NumPy数组
array1 = np.array(image1)
array2 = np.array(image2)
# 获取图像尺寸
height1, width1, channels1 = array1.shape
height2, width2, channels2 = array2.shape
# 创建一个新的数组,其尺寸为两张图像宽度之和,高度为两张图像的最大高度
new_array = np.zeros((max(height1, height2), width1 + width2, 3), dtype=np.uint8)
# 将两张图像粘贴到新数组上
new_array[0:height1, 0:width1] = array1
new_array[0:height2, width1:width1 + width2] = array2
# 将数组转换为图像
new_image = Image.fromarray(new_array)
# 保存合并后的图像
new_image.save(output_path)
测试合并图像
merge_images('path_to_image1.jpg', 'path_to_image2.jpg', 'merged_image.jpg')
四、总结
在Python中,合并图像的方法有很多,常用的库包括Pillow、OpenCV、NumPy。这些库提供了丰富的图像处理功能,可以帮助我们轻松地将两张图像合并。通过本文的介绍,我们详细了解了使用Pillow、OpenCV和NumPy合并图像的具体步骤和代码示例。
- Pillow库:是一个强大的图像处理库,支持多种格式的图像文件,并提供了强大的图像处理功能。我们可以使用Pillow库打开、显示、保存和处理图像。
- OpenCV库:是一个开源的计算机视觉和图像处理库,提供了丰富的图像处理功能。相比Pillow,OpenCV更加侧重于图像处理和计算机视觉领域。
- NumPy库:是一个用于科学计算的库,提供了多维数组对象和各种操作函数。虽然NumPy本身不是一个图像处理库,但我们可以结合Pillow或OpenCV来处理图像数据。
通过本文的学习,读者可以根据自己的需求选择合适的库来合并图像,并掌握具体的实现方法。希望本文对您有所帮助,祝您在图像处理领域取得更大的进步!
相关问答FAQs:
如何使用Python将两张图像合并为一张?
要将两张图像合并为一张,您可以使用Python的PIL(Pillow)库。首先,确保您安装了Pillow库,通过命令pip install Pillow
进行安装。接下来,您可以使用以下示例代码来合并图像:
from PIL import Image
# 打开两张图片
img1 = Image.open('image1.jpg')
img2 = Image.open('image2.jpg')
# 创建一个新的图像,宽度为两张图像的宽度之和,高度为较高的图像高度
new_img = Image.new('RGB', (img1.width + img2.width, max(img1.height, img2.height)))
# 将两张图像粘贴到新图像上
new_img.paste(img1, (0, 0))
new_img.paste(img2, (img1.width, 0))
# 保存合并后的图像
new_img.save('combined_image.jpg')
在合并图像时如何处理不同尺寸的图像?
在合并不同尺寸的图像时,可以选择将它们调整为相同的高度或宽度。使用PIL库中的resize()
函数可以方便地调整图像大小。例如,您可以将较小的图像调整为较大的图像的高度,以确保它们在合并时的视觉效果更佳。
合并图像时有哪些常见的图像格式支持?
Python的PIL库支持多种图像格式,包括JPEG、PNG、BMP、GIF等。在合并图像时,确保您选择的格式能够满足您的需求。例如,PNG格式支持透明背景,而JPEG格式则更适合照片类图像。
如何在合并图像时添加边距或间距?
在合并图像时,可以通过在新图像的尺寸计算中添加边距来创建间距。例如,在计算新图像的宽度时,可以加上需要的边距值。以下是一个示例代码:
margin = 10 # 设置边距
new_img = Image.new('RGB', (img1.width + img2.width + margin, max(img1.height, img2.height)))
new_img.paste(img1, (0, 0))
new_img.paste(img2, (img1.width + margin, 0))
这将确保两张图像之间有10个像素的空白间距。
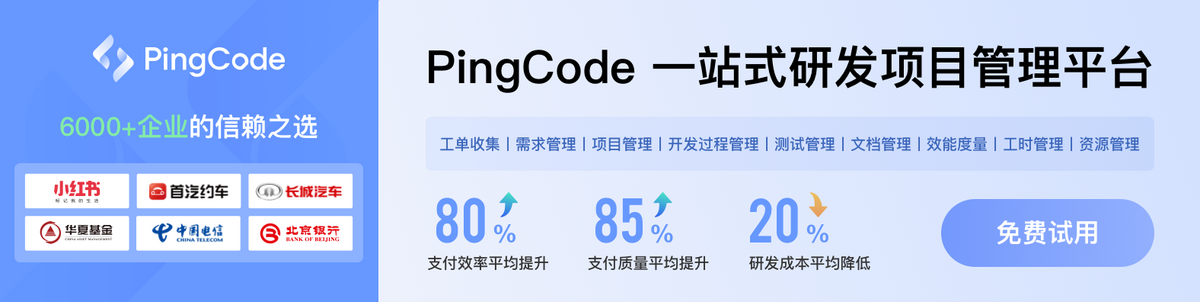