Python去掉字符串中某个字符串的方法有多种:使用replace方法、正则表达式、split和join方法等。推荐使用replace方法,因为它简单高效、代码易读、适用大部分情况。
replace方法可以直接替换字符串中的指定子字符串,不需要额外的库支持。举个例子,如果我们有一个字符串 "Hello, World!",想要去掉其中的逗号,可以这样做:
text = "Hello, World!"
new_text = text.replace(",", "")
print(new_text) # 输出: Hello World!
一、replace方法
replace方法是处理字符串的常用方法,通过将指定的子字符串替换为空字符串,可以达到去掉该子字符串的效果。replace方法具有以下特点:
- 简洁高效:代码简洁,性能优越。
- 代码易读:直观易懂,便于维护。
- 适用范围广:适用于各种简单替换需求。
示例代码:
original_string = "Python is amazing and Python is versatile."
substring_to_remove = "Python"
result_string = original_string.replace(substring_to_remove, "")
print(result_string) # 输出: " is amazing and is versatile."
在上面的例子中,我们将字符串中的所有 "Python" 替换成了空字符串,从而去掉了所有的 "Python"。
二、使用正则表达式
正则表达式(Regular Expressions)是处理复杂字符串匹配与替换的强大工具。Python的re模块提供了支持。在需要进行复杂的字符串替换或匹配时,正则表达式非常有用。
1、re.sub方法
re.sub方法用于替换字符串中的匹配项。它的使用方法如下:
import re
pattern = r"Python"
replacement = ""
original_string = "Python is amazing and Python is versatile."
result_string = re.sub(pattern, replacement, original_string)
print(result_string) # 输出: " is amazing and is versatile."
2、re.compile方法
re.compile方法可以预编译正则表达式,提高效率。对于频繁使用的正则表达式,可以考虑使用这种方法。
import re
pattern = re.compile(r"Python")
replacement = ""
original_string = "Python is amazing and Python is versatile."
result_string = pattern.sub(replacement, original_string)
print(result_string) # 输出: " is amazing and is versatile."
三、使用split和join方法
split方法将字符串拆分成一个列表,join方法将列表重新组合成字符串。通过split和join方法,我们可以去掉指定的子字符串。
original_string = "Python is amazing and Python is versatile."
substring_to_remove = "Python"
split_list = original_string.split(substring_to_remove)
result_string = "".join(split_list)
print(result_string) # 输出: " is amazing and is versatile."
四、字符串替换的性能比较
在实际应用中,选择高效的方法可以提高程序的性能。下面我们通过一个示例来比较replace方法和正则表达式方法的性能:
import time
import re
original_string = "Python " * 1000000
substring_to_remove = "Python"
测试replace方法
start_time = time.time()
result_string = original_string.replace(substring_to_remove, "")
end_time = time.time()
print(f"replace方法耗时: {end_time - start_time} 秒")
测试正则表达式方法
pattern = re.compile(substring_to_remove)
start_time = time.time()
result_string = pattern.sub("", original_string)
end_time = time.time()
print(f"正则表达式方法耗时: {end_time - start_time} 秒")
通过运行上述代码,可以发现replace方法通常比正则表达式方法更快。对于简单的字符串替换,推荐使用replace方法,而正则表达式适用于复杂的替换需求。
五、处理Unicode和多字节字符
在处理包含Unicode或多字节字符的字符串时,Python的字符串方法同样有效。以下是一个处理Unicode字符串的示例:
original_string = "Python 是非常棒的编程语言。Python 是非常灵活的。"
substring_to_remove = "Python"
result_string = original_string.replace(substring_to_remove, "")
print(result_string) # 输出: " 是非常棒的编程语言。 是非常灵活的。"
六、在实际项目中的应用
在实际项目中,字符串替换操作非常常见。例如,处理用户输入、清理数据、生成报告等场景都可能需要去掉指定的子字符串。以下是一个实际应用示例:
def clean_user_input(user_input):
unwanted_substring = "badword"
cleaned_input = user_input.replace(unwanted_substring, "")
return cleaned_input
user_input = "This is a badword test."
cleaned_input = clean_user_input(user_input)
print(cleaned_input) # 输出: "This is a test."
在这个示例中,我们实现了一个清理用户输入的函数,用于去掉不需要的子字符串。
七、总结
通过本文的介绍,我们了解了Python中去掉字符串中某个字符串的多种方法,包括replace方法、正则表达式、split和join方法等。其中,replace方法简单高效,适用于大部分情况,而正则表达式适用于复杂的字符串替换需求。根据实际需求选择合适的方法,可以提高代码的可读性和性能。
相关问答FAQs:
如何在Python中删除字符串中的特定子字符串?
在Python中,可以使用字符串的replace()
方法来删除特定的子字符串。只需将要删除的子字符串替换为空字符串即可。例如,string.replace("要删除的子字符串", "")
将返回一个新的字符串,其中所有的“要删除的子字符串”都被移除。
使用正则表达式能否去掉字符串中的某个子字符串?
是的,使用re
模块可以通过正则表达式来删除字符串中的特定子字符串。可以使用re.sub()
函数,语法为re.sub("要删除的子字符串", "", 原始字符串)
。这样可以灵活地匹配和删除更复杂的模式。
在处理字符串时,删除子字符串会影响原始字符串吗?
在Python中,字符串是不可变的,意味着原始字符串不会被改变。使用replace()
或re.sub()
方法时,都会返回一个新的字符串,而原始字符串保持不变。如果需要修改原始字符串,可以将新字符串赋值给原始字符串的变量。
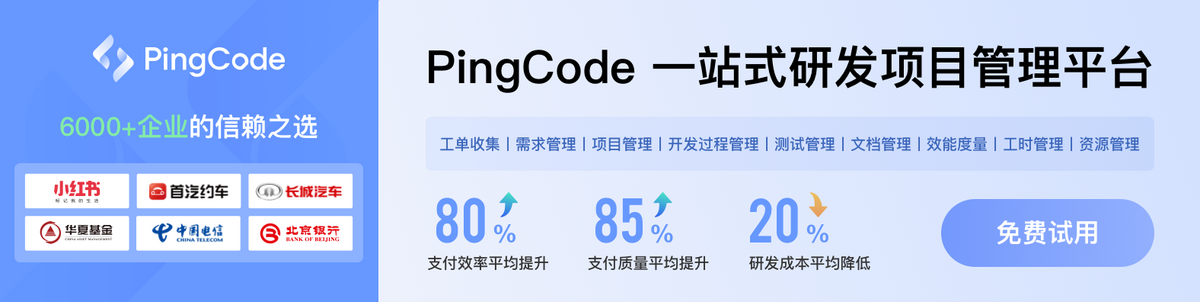