要让Python将两个字符串逐字比较,可以使用多种方法:利用循环遍历字符、使用内置函数、结合正则表达式等。在这篇文章中,我们将详细探讨这些方法,并提供示例代码来帮助你更好地理解。
一、利用循环逐字比较
循环遍历是逐字比较字符串的最直接方法。通过遍历字符串的每一个字符,我们可以逐字进行比较。
1.1 使用for循环
for循环是Python中最常用的循环结构之一,可以方便地遍历字符串中的每一个字符。
def compare_strings_for_loop(str1, str2):
len1 = len(str1)
len2 = len(str2)
min_len = min(len1, len2)
for i in range(min_len):
if str1[i] != str2[i]:
print(f"Character {i + 1} is different: '{str1[i]}' vs '{str2[i]}'")
if len1 != len2:
print("The strings are of different lengths.")
示例调用
compare_strings_for_loop("hello", "hallo")
1.2 使用while循环
while循环功能相似,但更灵活,可以在满足特定条件时终止。
def compare_strings_while_loop(str1, str2):
i = 0
len1 = len(str1)
len2 = len(str2)
min_len = min(len1, len2)
while i < min_len:
if str1[i] != str2[i]:
print(f"Character {i + 1} is different: '{str1[i]}' vs '{str2[i]}'")
i += 1
if len1 != len2:
print("The strings are of different lengths.")
示例调用
compare_strings_while_loop("hello", "hullo")
二、使用内置函数进行比较
Python提供了一些内置函数,可以简化字符串比较的工作。
2.1 使用zip函数
zip函数可以将两个字符串对应位置的字符打包成元组,方便逐字比较。
def compare_strings_zip(str1, str2):
for i, (char1, char2) in enumerate(zip(str1, str2)):
if char1 != char2:
print(f"Character {i + 1} is different: '{char1}' vs '{char2}'")
if len(str1) != len(str2):
print("The strings are of different lengths.")
示例调用
compare_strings_zip("hello", "helxo")
2.2 使用map函数
map函数可以将一个函数应用于两个字符串的每个字符,并返回一个迭代器。
def compare_strings_map(str1, str2):
differences = list(map(lambda x, y: x != y, str1, str2))
for i, diff in enumerate(differences):
if diff:
print(f"Character {i + 1} is different: '{str1[i]}' vs '{str2[i]}'")
if len(str1) != len(str2):
print("The strings are of different lengths.")
示例调用
compare_strings_map("hello", "hezlo")
三、结合正则表达式进行比较
正则表达式是一种强大的工具,可以用于复杂模式匹配和字符串操作。
3.1 使用re模块
虽然正则表达式主要用于复杂的字符串匹配,但也可以用于字符串比较。
import re
def compare_strings_regex(str1, str2):
min_len = min(len(str1), len(str2))
for i in range(min_len):
if re.match(re.escape(str1[i]), str2[i]) is None:
print(f"Character {i + 1} is different: '{str1[i]}' vs '{str2[i]}'")
if len(str1) != len(str2):
print("The strings are of different lengths.")
示例调用
compare_strings_regex("hello", "heLlo")
四、进一步优化和应用
在实际应用中,逐字比较字符串可能需要结合其他逻辑,如处理大小写、忽略空格等。
4.1 忽略大小写
可以在比较之前将字符串转换为小写或大写。
def compare_strings_ignore_case(str1, str2):
str1 = str1.lower()
str2 = str2.lower()
compare_strings_for_loop(str1, str2)
示例调用
compare_strings_ignore_case("Hello", "hello")
4.2 忽略空格
可以在比较之前去除字符串中的空格。
def compare_strings_ignore_spaces(str1, str2):
str1 = str1.replace(" ", "")
str2 = str2.replace(" ", "")
compare_strings_for_loop(str1, str2)
示例调用
compare_strings_ignore_spaces("he llo", "hello")
五、综合示例
结合上述所有方法,我们可以编写一个综合函数来处理各种情况。
def compare_strings_comprehensive(str1, str2, ignore_case=False, ignore_spaces=False):
if ignore_case:
str1 = str1.lower()
str2 = str2.lower()
if ignore_spaces:
str1 = str1.replace(" ", "")
str2 = str2.replace(" ", "")
len1 = len(str1)
len2 = len(str2)
min_len = min(len1, len2)
for i in range(min_len):
if str1[i] != str2[i]:
print(f"Character {i + 1} is different: '{str1[i]}' vs '{str2[i]}'")
if len1 != len2:
print("The strings are of different lengths.")
示例调用
compare_strings_comprehensive("Hello world", "hello world!", ignore_case=True, ignore_spaces=True)
六、性能优化
在处理大规模字符串比较时,性能优化非常重要。以下是一些优化建议:
6.1 使用生成器
生成器可以在需要时生成值,减少内存使用。
def compare_strings_generator(str1, str2):
def generator():
for i, (char1, char2) in enumerate(zip(str1, str2)):
if char1 != char2:
yield f"Character {i + 1} is different: '{char1}' vs '{char2}'"
for result in generator():
print(result)
if len(str1) != len(str2):
print("The strings are of different lengths.")
示例调用
compare_strings_generator("hello", "heLlo")
6.2 使用多线程
对于非常大的字符串,可以考虑使用多线程进行比较。
import threading
def compare_strings_multithreaded(str1, str2):
def worker(start, end, results):
for i in range(start, end):
if str1[i] != str2[i]:
results.append(f"Character {i + 1} is different: '{str1[i]}' vs '{str2[i]}'")
len1 = len(str1)
len2 = len(str2)
min_len = min(len1, len2)
results = []
threads = []
num_threads = 4
chunk_size = min_len // num_threads
for i in range(num_threads):
start = i * chunk_size
end = (i + 1) * chunk_size if i != num_threads - 1 else min_len
thread = threading.Thread(target=worker, start=start, end=end, args=(results,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
for result in results:
print(result)
if len1 != len2:
print("The strings are of different lengths.")
示例调用
compare_strings_multithreaded("hellohellohellohello", "heLlohellohellohello")
通过上述方法,我们可以灵活地实现Python字符串逐字比较,并根据具体需求进行优化。希望这篇文章能对你有所帮助。
相关问答FAQs:
如何在Python中实现字符串逐字比较?
在Python中,可以使用循环或者列表推导式来实现字符串的逐字比较。你可以使用zip()
函数将两个字符串组合在一起,然后逐个字母进行比较。例如:
str1 = "hello"
str2 = "hxllo"
comparison = [(c1, c2) for c1, c2 in zip(str1, str2) if c1 != c2]
print(comparison)
这段代码会输出不同的字符对,帮助你了解两个字符串之间的差异。
Python提供了哪些内置函数来帮助比较字符串?
Python提供了多个内置函数来帮助字符串的比较,例如==
运算符可以直接比较两个字符串是否相等,str.casefold()
可以用于不区分大小写的比较,str.find()
和str.index()
可以帮助查找某个子字符串的位置。使用这些函数,可以灵活地对字符串进行比较和处理。
如何处理长度不同的字符串比较?
当两个字符串长度不同时,可以通过对较短的字符串进行填充或截断来进行逐字比较。比如,可以使用str.ljust()
方法在较短的字符串末尾填充空白字符,这样就可以确保两个字符串的长度相同,从而进行逐字比较。示例代码如下:
str1 = "hello"
str2 = "hi"
str1_padded = str1.ljust(len(str2))
comparison = [(c1, c2) for c1, c2 in zip(str1_padded, str2)]
print(comparison)
这样就能够对不同长度的字符串进行有效比较。
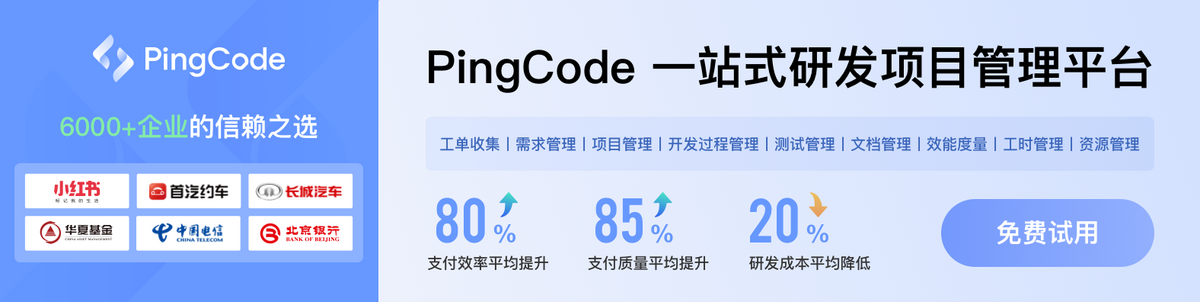