Python如何把文件复制到另一个目录下
使用Python将文件复制到另一个目录下,可以通过使用shutil模块、os模块、自定义函数等多种方式实现。 其中,最常用的方式是使用shutil模块,这个模块提供了许多文件操作的功能,如复制、移动、删除等。本文将详细介绍各种方法并提供相应的代码示例。
一、使用shutil模块
shutil模块是Python标准库中的一个模块,提供了高级的文件操作功能。可以使用shutil.copy()
和shutil.copy2()
方法将文件复制到另一个目录下。
1、shutil.copy()方法
shutil.copy(src, dst)
将文件src复制到dst。这个方法仅复制文件的内容和权限,但不包括元数据(如创建时间、修改时间)。
import shutil
src_file = 'path/to/source/file.txt'
dst_directory = 'path/to/destination/'
shutil.copy(src_file, dst_directory)
print(f"{src_file} has been copied to {dst_directory}")
2、shutil.copy2()方法
shutil.copy2(src, dst)
与shutil.copy()
类似,但它会同时复制文件的内容、权限和元数据。
import shutil
src_file = 'path/to/source/file.txt'
dst_directory = 'path/to/destination/'
shutil.copy2(src_file, dst_directory)
print(f"{src_file} has been copied to {dst_directory} with metadata")
二、使用os模块
os模块提供了与操作系统交互的功能,但没有直接的复制文件的方法。可以通过读取和写入文件的方式实现文件复制。
1、读取和写入文件
通过os模块打开源文件,读取其内容,然后在目标目录下创建一个新文件并写入读取的内容。
import os
src_file = 'path/to/source/file.txt'
dst_directory = 'path/to/destination/'
Ensure the destination directory exists
if not os.path.exists(dst_directory):
os.makedirs(dst_directory)
with open(src_file, 'rb') as f_src:
with open(os.path.join(dst_directory, os.path.basename(src_file)), 'wb') as f_dst:
f_dst.write(f_src.read())
print(f"{src_file} has been copied to {dst_directory}")
三、使用Pathlib模块
Pathlib是Python 3.4引入的一个模块,提供了面向对象的文件系统路径操作。可以使用Pathlib的Path
类的copy()
方法来实现文件复制。
from pathlib import Path
import shutil
src_file = Path('path/to/source/file.txt')
dst_directory = Path('path/to/destination/')
Ensure the destination directory exists
dst_directory.mkdir(parents=True, exist_ok=True)
shutil.copy(src_file, dst_directory)
print(f"{src_file} has been copied to {dst_directory}")
四、使用第三方库
有时,标准库的功能可能不够用,可以考虑使用第三方库如send2trash
和pyfilesystem2
,这些库提供了更高级的文件操作功能。
1、send2trash库
send2trash
库提供了将文件和文件夹移动到回收站的功能,而不是直接删除。虽然这个库主要用于删除操作,但也可以用来实现文件复制。
from send2trash import send2trash
src_file = 'path/to/source/file.txt'
dst_directory = 'path/to/destination/'
Ensure the destination directory exists
if not os.path.exists(dst_directory):
os.makedirs(dst_directory)
shutil.copy(src_file, dst_directory)
send2trash(src_file)
print(f"{src_file} has been moved to {dst_directory} and original file sent to trash")
2、pyfilesystem2库
pyfilesystem2
是一个文件系统抽象层,可以用来处理各种文件系统操作。这个库提供了丰富的文件操作API,可以方便地实现文件复制等操作。
import fs
src_file = 'path/to/source/file.txt'
dst_directory = 'path/to/destination/'
Open the source file system
with fs.open_fs('osfs://.') as src_fs:
# Open the destination file system
with fs.open_fs('osfs://' + dst_directory) as dst_fs:
src_fs.copy(src_file, dst_fs)
print(f"{src_file} has been copied to {dst_directory}")
五、最佳实践和注意事项
在实际应用中,需要考虑以下几点:
1、处理异常
在进行文件操作时,可能会遇到各种异常情况,如文件不存在、权限不足等。因此,需要使用try-except语句来捕获和处理这些异常。
import shutil
import os
src_file = 'path/to/source/file.txt'
dst_directory = 'path/to/destination/'
try:
if not os.path.exists(dst_directory):
os.makedirs(dst_directory)
shutil.copy2(src_file, dst_directory)
print(f"{src_file} has been copied to {dst_directory} with metadata")
except FileNotFoundError as e:
print(f"Error: {e}")
except PermissionError as e:
print(f"Error: {e}")
2、处理大文件
对于大文件,可以使用分块读取和写入的方式来避免内存溢出。
import os
src_file = 'path/to/source/file.txt'
dst_directory = 'path/to/destination/'
buffer_size = 1024 * 1024 # 1MB
if not os.path.exists(dst_directory):
os.makedirs(dst_directory)
with open(src_file, 'rb') as f_src:
with open(os.path.join(dst_directory, os.path.basename(src_file)), 'wb') as f_dst:
while True:
buffer = f_src.read(buffer_size)
if not buffer:
break
f_dst.write(buffer)
print(f"{src_file} has been copied to {dst_directory}")
3、跨平台兼容性
在编写跨平台的代码时,需要注意路径分隔符的差异。可以使用os.path.join()方法来构建路径,以确保代码在不同操作系统上运行正常。
import os
src_file = os.path.join('path', 'to', 'source', 'file.txt')
dst_directory = os.path.join('path', 'to', 'destination')
if not os.path.exists(dst_directory):
os.makedirs(dst_directory)
shutil.copy2(src_file, dst_directory)
print(f"{src_file} has been copied to {dst_directory} with metadata")
六、总结
使用Python将文件复制到另一个目录下,可以通过shutil模块、os模块、Pathlib模块以及第三方库来实现。每种方法都有其优缺点和适用场景,选择合适的方法可以提高代码的可读性和可维护性。在实际应用中,还需要处理异常情况、考虑大文件的处理方式以及确保代码的跨平台兼容性。
通过本文的介绍,相信你已经掌握了多种在Python中复制文件的方法。希望这些内容对你有所帮助。
相关问答FAQs:
如何在Python中使用 shutil 库复制文件?
使用 Python 的 shutil 库可以轻松地复制文件。首先,您需要导入 shutil 库,然后使用 shutil.copy()
函数指定源文件路径和目标目录。这个函数不仅会复制文件内容,还会保留文件的权限信息。
在复制文件时,如何处理文件已存在的情况?
在复制文件到目标目录时,如果目标文件已存在,shutil.copy() 会覆盖它。如果您想避免这种情况,可以先检查目标文件是否存在,使用 os.path.exists()
函数来判断,并决定是否进行复制或是重命名。
使用 Python 复制文件时,如何处理不同的文件格式?
Python 的 shutil.copy() 函数可以处理各种文件格式,包括文本文件、图像文件和二进制文件等。无论文件类型如何,复制方法是相同的。确保在指定源文件路径时使用正确的文件格式,以便顺利完成复制操作。
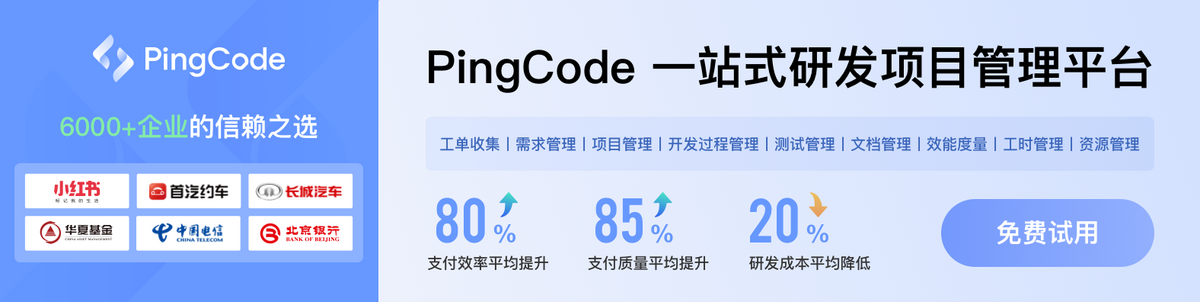