在Python中,您可以通过几种方法将列表中的数值进行四舍五入,包括使用内置的round()函数、列表推导式、以及NumPy库。以下是详细的解答和实践方法。最常用的方法是使用内置的round()
函数,因为它简单且适用于大多数情况。
一、使用内置的round()函数
Python提供了一个内置的round()
函数,可以直接将数值四舍五入。你可以利用列表推导式将列表中的每个元素应用round()
函数。
# 原始列表
numbers = [1.234, 2.678, 3.456, 4.987]
使用列表推导式和round()函数
rounded_numbers = [round(num) for num in numbers]
print(rounded_numbers)
二、使用map()函数结合round()函数
另一种方法是使用map()
函数,这个函数可以将round()
函数应用到列表的每一个元素。
# 原始列表
numbers = [1.234, 2.678, 3.456, 4.987]
使用map()函数和round()函数
rounded_numbers = list(map(round, numbers))
print(rounded_numbers)
三、使用NumPy库进行四舍五入
如果你处理的是大量数据,或者需要更复杂的数学操作,NumPy库提供了更高效和更灵活的解决方案。NumPy的round()
函数可以对数组进行四舍五入。
import numpy as np
原始列表
numbers = [1.234, 2.678, 3.456, 4.987]
转换为NumPy数组
numbers_array = np.array(numbers)
使用NumPy的round()函数
rounded_numbers_array = np.round(numbers_array)
print(rounded_numbers_array)
四、指定小数点位数
在某些情况下,你可能需要将数值四舍五入到特定的小数点位数。round()
函数可以接收第二个参数来指定小数点位数。
# 原始列表
numbers = [1.234, 2.678, 3.456, 4.987]
使用列表推导式和round()函数,指定小数点位数
rounded_numbers = [round(num, 2) for num in numbers]
print(rounded_numbers)
五、处理嵌套列表
如果你的列表是嵌套结构,即列表中包含列表,你可以使用递归函数进行四舍五入。
# 原始嵌套列表
nested_numbers = [[1.234, 2.678], [3.456, 4.987]]
def round_nested_list(lst, decimal_places=0):
rounded_list = []
for element in lst:
if isinstance(element, list):
rounded_list.append(round_nested_list(element, decimal_places))
else:
rounded_list.append(round(element, decimal_places))
return rounded_list
使用递归函数进行四舍五入
rounded_nested_numbers = round_nested_list(nested_numbers, 2)
print(rounded_nested_numbers)
六、性能和效率
在处理大规模数据时,选择合适的方法尤为重要。对于小规模数据,内置的round()
函数和map()
函数是非常高效的选择。但如果你处理的是数十万甚至数百万的数据,使用NumPy库可能会更高效,因为它是专门为大规模数据处理而设计的。
七、实际应用案例
考虑一个实际的应用场景:你是一个数据分析师,需要对一组金融数据进行处理。这些数据包含了许多小数,你需要将这些数据四舍五入到两位小数,以便于后续的统计分析和报告。
import numpy as np
模拟金融数据
financial_data = np.random.uniform(0, 1000, 1000000)
将数据四舍五入到两位小数
rounded_financial_data = np.round(financial_data, 2)
进行统计分析
mean_value = np.mean(rounded_financial_data)
median_value = np.median(rounded_financial_data)
std_deviation = np.std(rounded_financial_data)
print(f"Mean: {mean_value}, Median: {median_value}, Standard Deviation: {std_deviation}")
通过这些方法,你可以在Python中方便且高效地对列表中的数值进行四舍五入处理。无论是简单的列表推导式,还是使用功能更强大的NumPy库,都能满足不同场景下的需求。选择合适的方法,不仅能提高代码的可读性,还能显著提升代码的执行效率。
相关问答FAQs:
如何在Python中对列表中的数字进行四舍五入?
在Python中,可以使用内置的round()
函数对列表中的每个数字进行四舍五入。可以通过列表推导式来实现这一点。例如,假设你有一个包含浮点数的列表,可以通过以下代码将每个数字四舍五入到最接近的整数:
numbers = [1.2, 2.5, 3.7, 4.1]
rounded_numbers = [round(num) for num in numbers]
是否可以指定四舍五入的小数位数?
确实可以。round()
函数允许你指定所需的小数位数。只需在调用round()
时添加第二个参数。例如,如果你想将列表中的数字四舍五入到小数点后两位,可以这样做:
numbers = [1.234, 2.678, 3.456]
rounded_numbers = [round(num, 2) for num in numbers]
如果列表中包含非数字元素,如何处理?
在处理包含非数字元素的列表时,可以使用try-except
结构来避免错误。可以在遍历列表时检查每个元素是否为数字,如果是,则进行四舍五入,否则可以选择保留该元素或用特定值替代。例如:
numbers = [1.2, 'a', 2.5, None, 3.7]
rounded_numbers = []
for num in numbers:
try:
rounded_numbers.append(round(num))
except TypeError:
rounded_numbers.append(None) # 或者用其他默认值替代
这种方式确保了在列表中遇到非数字元素时不会导致程序崩溃,同时仍然能够处理有效的数字。
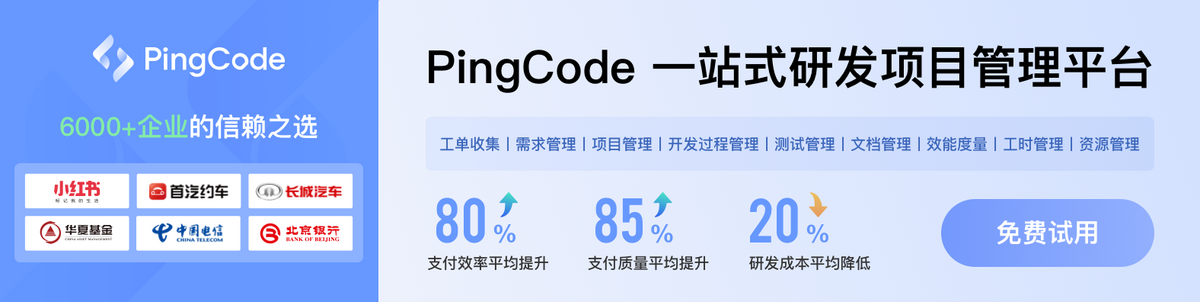