要用Python把三个数从小到大排列,可以使用内置的排序函数、比较运算符、或自定义的排序逻辑。在这篇文章中,我们将详细探讨如何使用Python实现这一目标,并提供多种方法来满足不同的需求。本文将涵盖内置排序函数、手动排序逻辑、以及其他一些高级技巧,以确保您能够找到最适合您情况的方法。
一、使用内置排序函数
Python 提供了强大的内置函数 sorted()
和 list.sort()
,使得排序变得非常简单。我们可以直接利用这些函数来对三个数进行排序。
1.1 sorted()
函数
sorted()
函数返回一个新的排好序的列表。这个函数不会改变原来的列表。
def sort_three_numbers(a, b, c):
return sorted([a, b, c])
示例
a, b, c = 3, 1, 2
sorted_numbers = sort_three_numbers(a, b, c)
print(sorted_numbers) # 输出: [1, 2, 3]
1.2 list.sort()
方法
list.sort()
方法会就地对列表进行排序,并不会返回新的列表。
def sort_three_numbers_in_place(a, b, c):
numbers = [a, b, c]
numbers.sort()
return numbers
示例
a, b, c = 3, 1, 2
sorted_numbers = sort_three_numbers_in_place(a, b, c)
print(sorted_numbers) # 输出: [1, 2, 3]
二、使用比较运算符手动排序
虽然内置函数很方便,但有时我们可能希望了解排序的内部工作机制。手动排序可以帮助我们更好地理解排序算法。
2.1 两两比较法
我们可以通过两两比较并交换位置的方式来排序三个数。
def manual_sort_three_numbers(a, b, c):
if a > b:
a, b = b, a
if b > c:
b, c = c, b
if a > b:
a, b = b, a
return [a, b, c]
示例
a, b, c = 3, 1, 2
sorted_numbers = manual_sort_three_numbers(a, b, c)
print(sorted_numbers) # 输出: [1, 2, 3]
2.2 嵌套条件法
这种方法通过嵌套条件来直接确定每个位置的数值。
def nested_condition_sort(a, b, c):
if a <= b and a <= c:
if b <= c:
return [a, b, c]
else:
return [a, c, b]
elif b <= a and b <= c:
if a <= c:
return [b, a, c]
else:
return [b, c, a]
else:
if a <= b:
return [c, a, b]
else:
return [c, b, a]
示例
a, b, c = 3, 1, 2
sorted_numbers = nested_condition_sort(a, b, c)
print(sorted_numbers) # 输出: [1, 2, 3]
三、使用库函数
有时,我们可能需要使用一些更复杂的排序逻辑或进行更多的操作。Python 提供了多个库,可以帮助我们实现这一点。
3.1 使用 numpy
库
numpy
库是一个强大的数值计算库,可以方便地进行数组操作和排序。
import numpy as np
def numpy_sort(a, b, c):
numbers = np.array([a, b, c])
return np.sort(numbers)
示例
a, b, c = 3, 1, 2
sorted_numbers = numpy_sort(a, b, c)
print(sorted_numbers) # 输出: [1, 2, 3]
3.2 使用 pandas
库
pandas
是一个数据分析库,虽然主要用于处理表格数据,但也可以进行简单的排序操作。
import pandas as pd
def pandas_sort(a, b, c):
numbers = pd.Series([a, b, c])
return numbers.sort_values().tolist()
示例
a, b, c = 3, 1, 2
sorted_numbers = pandas_sort(a, b, c)
print(sorted_numbers) # 输出: [1, 2, 3]
四、优化和扩展
在实际应用中,我们可能需要对更多的数进行排序,或者需要更高效的排序算法。
4.1 对更多数进行排序
如果需要排序的不止三个数,我们可以直接扩展之前的方法。
def sort_numbers(*args):
return sorted(args)
示例
numbers = sort_numbers(3, 1, 2, 5, 4)
print(numbers) # 输出: [1, 2, 3, 4, 5]
4.2 使用高效排序算法
对于更大规模的数据,我们可以使用更高效的排序算法,如快速排序(Quick Sort)或归并排序(Merge Sort)。
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
示例
numbers = [3, 1, 2, 5, 4]
sorted_numbers = quick_sort(numbers)
print(sorted_numbers) # 输出: [1, 2, 3, 4, 5]
五、总结
通过这篇文章,我们探讨了多种用Python对三个数进行排序的方法,包括使用内置函数、手动排序、以及使用库函数等。每种方法都有其优缺点,选择哪种方法取决于具体的应用场景和需求。
无论是简单的排序需求,还是复杂的数据处理任务,Python都能提供灵活和高效的解决方案。希望本文能帮助您更好地理解和应用这些方法。
相关问答FAQs:
如何在Python中实现三个数的排序?
在Python中,可以使用内置的sorted()
函数来对三个数进行排序。您只需将这三个数字放入一个列表中,然后调用sorted()
函数即可。例如:
numbers = [3, 1, 2]
sorted_numbers = sorted(numbers)
print(sorted_numbers) # 输出:[1, 2, 3]
这样就可以轻松实现从小到大的排序。
Python中是否有其他方法可以排序三个数?
除了使用sorted()
函数,还可以使用条件语句来手动排序这三个数。可以通过比较它们的值来实现,具体代码示例如下:
a, b, c = 3, 1, 2
if a > b:
a, b = b, a
if a > c:
a, c = c, a
if b > c:
b, c = c, b
print(a, b, c) # 输出:1 2 3
这种方法适用于需要更深入理解排序逻辑的场景。
在Python中,能否使用第三方库来排序数字?
确实可以使用第三方库来进行排序,例如NumPy。NumPy是一个强大的科学计算库,提供了高效的数组操作功能。可以通过以下方式对三个数字进行排序:
import numpy as np
numbers = np.array([3, 1, 2])
sorted_numbers = np.sort(numbers)
print(sorted_numbers) # 输出:[1 2 3]
这种方法适合处理更复杂的数据结构或者更大的数据集。
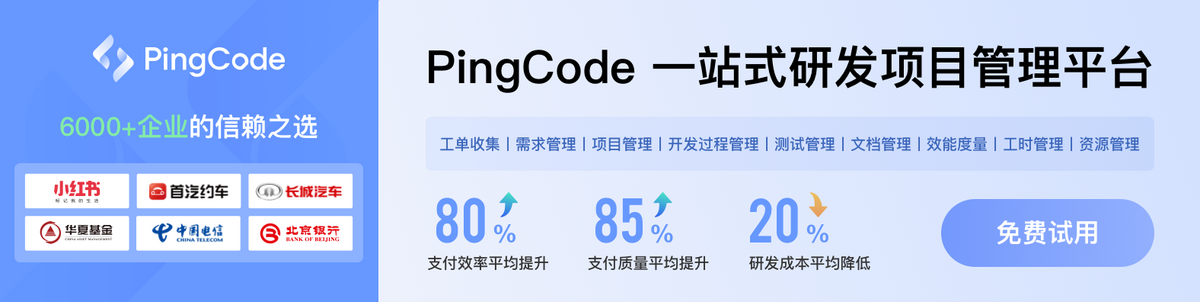