在Python中,替换一个字符串可以通过多种方法实现,包括使用内置的字符串方法、正则表达式以及其他高级技巧。最常用的方法有:使用str.replace()、使用正则表达式的re.sub()、以及通过字符串拼接。
其中,使用str.replace()
方法是最简单且常用的方法。它能够快速且高效地替换字符串中的子字符串。例如,如果你有一个字符串"Hello World"并且你想要将"World"替换为"Python",你可以使用str.replace()
方法来实现这一点。
text = "Hello World"
new_text = text.replace("World", "Python")
print(new_text) # 输出: Hello Python
接下来,我们将详细探讨Python中替换字符串的各种方法和技巧。
一、使用str.replace()方法
str.replace()
是Python中最常用的字符串替换方法。它的语法非常简单,接受两个参数:要被替换的子字符串和替换后的子字符串。
original_string = "I love apples, apples are my favorite fruit."
new_string = original_string.replace("apples", "oranges")
print(new_string) # 输出: I love oranges, oranges are my favorite fruit.
1.1、基本用法
str.replace()
方法在替换过程中不会改变原字符串,而是返回一个新的字符串。原字符串保持不变,因为字符串在Python中是不可变的。
1.2、指定替换次数
你还可以使用第三个参数count
来指定替换的次数:
original_string = "apple apple apple"
new_string = original_string.replace("apple", "orange", 2)
print(new_string) # 输出: orange orange apple
在这个例子中,只有前两个"apple"被替换为"orange"。
二、使用正则表达式re.sub()
对于更复杂的替换需求,尤其是需要匹配模式的情况,re.sub()
是一个强大的工具。re
模块提供了正则表达式的支持,可以进行复杂的字符串操作。
2.1、基本用法
import re
original_string = "The rain in Spain stays mainly in the plain."
new_string = re.sub(r"\brain\b", "sun", original_string)
print(new_string) # 输出: The sun in Spain stays mainly in the plain.
2.2、使用正则表达式模式
正则表达式允许你使用模式来匹配字符串中的特定部分。例如,你可以匹配所有的数字并将它们替换为特定的字符:
original_string = "My phone number is 123-456-7890."
new_string = re.sub(r"\d", "*", original_string)
print(new_string) # 输出: My phone number is <strong>*-</strong>*-<strong></strong>.
2.3、使用替换函数
re.sub()
还允许你使用一个函数来动态生成替换内容:
def replace_func(match):
return match.group(0).upper()
original_string = "hello world"
new_string = re.sub(r"\b\w+\b", replace_func, original_string)
print(new_string) # 输出: HELLO WORLD
三、通过字符串拼接
在某些情况下,你可能需要手动拼接字符串来实现更复杂的替换逻辑。这种方法虽然不如前两种方法高效,但在某些特定场景下可能更加灵活。
3.1、基本用法
你可以使用字符串切片和拼接来手动替换子字符串:
original_string = "abcdef"
start_index = original_string.find("cd")
end_index = start_index + len("cd")
new_string = original_string[:start_index] + "XY" + original_string[end_index:]
print(new_string) # 输出: abXYef
3.2、结合条件判断
你还可以结合条件判断来实现更复杂的替换逻辑。例如,根据子字符串的某些特征来决定是否替换:
original_string = "I have 2 apples and 3 oranges."
new_parts = []
for word in original_string.split():
if word.isdigit():
new_parts.append(str(int(word) * 2))
else:
new_parts.append(word)
new_string = " ".join(new_parts)
print(new_string) # 输出: I have 4 apples and 6 oranges.
四、使用字符串模板
Python的string
模块提供了模板字符串的功能,可以用来进行复杂的字符串替换。模板字符串允许你使用占位符,并在运行时进行替换。
4.1、基本用法
from string import Template
template = Template("Hello, $name!")
new_string = template.safe_substitute(name="Alice")
print(new_string) # 输出: Hello, Alice!
4.2、使用字典进行批量替换
你可以使用字典来进行批量替换:
template = Template("Hello, $name! You have $messages new messages.")
data = {
"name": "Bob",
"messages": 5
}
new_string = template.safe_substitute(data)
print(new_string) # 输出: Hello, Bob! You have 5 new messages.
五、字符串映射与翻译表
Python提供了str.translate()
方法,可以结合翻译表进行字符级别的替换。这个方法非常高效,适用于需要替换多个字符的情况。
5.1、创建翻译表
你可以使用str.maketrans()
方法来创建一个翻译表,然后使用str.translate()
方法进行替换:
original_string = "abcdef"
translation_table = str.maketrans("abc", "123")
new_string = original_string.translate(translation_table)
print(new_string) # 输出: 123def
5.2、删除字符
你还可以在翻译表中指定删除字符:
original_string = "hello world"
translation_table = str.maketrans("", "", "aeiou")
new_string = original_string.translate(translation_table)
print(new_string) # 输出: hll wrld
六、结合多种方法
在实际应用中,你可能需要结合多种方法来完成复杂的替换任务。例如,先使用正则表达式匹配特定模式,然后使用字符串拼接或模板字符串进行替换。
6.1、结合使用re.sub()和str.replace()
import re
original_string = "My email is example@example.com."
使用正则表达式匹配电子邮件地址
email_pattern = re.compile(r"\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b")
替换电子邮件地址中的域名
new_string = email_pattern.sub(lambda x: x.group().replace("example.com", "domain.com"), original_string)
print(new_string) # 输出: My email is example@domain.com.
6.2、结合使用模板字符串和条件判断
from string import Template
original_string = "User: $user, Status: $status"
template = Template(original_string)
data = {"user": "Alice", "status": "active"}
条件判断替换
if data["status"] == "active":
data["status"] = "online"
new_string = template.safe_substitute(data)
print(new_string) # 输出: User: Alice, Status: online
七、性能考虑
在选择替换方法时,性能是一个需要考虑的重要因素。一般来说,str.replace()
方法在处理简单替换任务时效率最高,而正则表达式和其他方法可能会在处理复杂任务时表现更好。
7.1、性能测试
下面是一个简单的性能测试,比较str.replace()
和re.sub()
的速度:
import time
import re
original_string = "a" * 1000000
start_time = time.time()
new_string = original_string.replace("a", "b")
print("str.replace()耗时:", time.time() - start_time)
start_time = time.time()
new_string = re.sub("a", "b", original_string)
print("re.sub()耗时:", time.time() - start_time)
一般来说,str.replace()
在简单替换任务中表现较好,而re.sub()
在处理复杂模式匹配时更为强大。
八、实际应用示例
8.1、替换文件中的特定字符串
在实际项目中,你可能需要替换文件中的特定字符串:
file_path = "example.txt"
with open(file_path, "r") as file:
content = file.read()
new_content = content.replace("old_string", "new_string")
with open(file_path, "w") as file:
file.write(new_content)
8.2、批量替换多个文件中的字符串
在大规模项目中,你可能需要批量替换多个文件中的字符串:
import os
directory = "path/to/directory"
old_string = "old_string"
new_string = "new_string"
for filename in os.listdir(directory):
if filename.endswith(".txt"):
file_path = os.path.join(directory, filename)
with open(file_path, "r") as file:
content = file.read()
new_content = content.replace(old_string, new_string)
with open(file_path, "w") as file:
file.write(new_content)
九、总结
替换字符串是Python编程中的常见任务。通过掌握str.replace()
、正则表达式、字符串拼接、模板字符串以及翻译表等多种方法,你可以应对各种复杂的字符串替换需求。在实际应用中,根据具体需求选择合适的方法,以确保替换操作的高效和准确。
相关问答FAQs:
如何在Python中使用内置函数替换字符串?
在Python中,使用字符串的replace()
方法可以轻松替换字符串中的指定部分。该方法接受两个参数,第一个是要被替换的子字符串,第二个是用于替换的新字符串。例如,string.replace("old", "new")
会将字符串string
中的所有"old"替换为"new"。如果想要限制替换的次数,可以添加第三个参数,指定替换的最大次数。
Python中是否可以使用正则表达式来替换字符串?
是的,Python的re
模块提供了强大的正则表达式支持,允许用户进行复杂的字符串替换。使用re.sub()
函数,可以根据指定的模式替换字符串中的内容。例如,re.sub(r'\d+', 'number', string)
会将字符串中的所有数字替换为“number”。这为处理模式匹配和复杂替换提供了灵活性。
在Python中如何替换字符串的同时保留原始字符串?
如果希望在替换字符串的同时保留原始字符串,可以简单地将替换后的结果赋值给一个新的变量。例如,可以使用new_string = original_string.replace("old", "new")
来实现。这种方法不会改变原始字符串original_string
,而是创建一个新的字符串new_string
,其内容为替换后的结果。这样,原始数据依然可以在后续操作中使用。
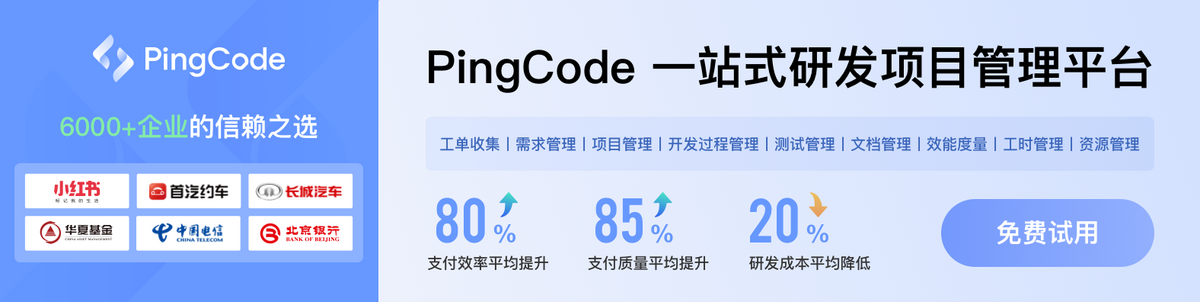