Python 修改文件名前4个字符串的方法
修改文件名前4个字符串的任务在Python中可以通过标准库os
和shutil
轻松完成。使用os
模块读取文件名、通过字符串操作更改前4个字符、使用os.rename
或shutil.move
进行重命名,是实现这个任务的标准方法。下面我们将详细讲解这个过程,并提供一个完整的代码示例。
一、导入必要的库
在开始之前,我们需要导入必要的Python库。主要用到的库是os
,它提供了一些函数来处理文件和目录。
import os
二、获取文件列表
在操作文件之前,我们需要获取目标目录下的所有文件名。这可以通过os.listdir
函数来实现,它返回指定目录中的文件和目录列表。
directory = 'your_directory_path'
files = os.listdir(directory)
三、修改文件名前4个字符
接下来,我们遍历文件列表,对每个文件名进行前4个字符的修改。我们可以使用字符串切片来完成这一操作。
for file in files:
if len(file) > 4:
new_name = 'new_prefix' + file[4:]
old_file_path = os.path.join(directory, file)
new_file_path = os.path.join(directory, new_name)
os.rename(old_file_path, new_file_path)
四、处理文件名冲突和异常
在重命名文件时,可能会遇到文件名冲突或其他异常情况。我们可以通过捕获异常来处理这些情况。
for file in files:
try:
if len(file) > 4:
new_name = 'new_prefix' + file[4:]
old_file_path = os.path.join(directory, file)
new_file_path = os.path.join(directory, new_name)
os.rename(old_file_path, new_file_path)
except Exception as e:
print(f"Error renaming file {file}: {e}")
五、完整代码示例
以下是一个完整的Python脚本示例,用于修改指定目录下所有文件名前4个字符:
import os
def rename_files_in_directory(directory, new_prefix):
# 获取指定目录下的所有文件
files = os.listdir(directory)
for file in files:
try:
# 检查文件名长度是否大于4
if len(file) > 4:
# 修改文件名前4个字符
new_name = new_prefix + file[4:]
old_file_path = os.path.join(directory, file)
new_file_path = os.path.join(directory, new_name)
# 重命名文件
os.rename(old_file_path, new_file_path)
print(f"Renamed {file} to {new_name}")
except Exception as e:
print(f"Error renaming file {file}: {e}")
使用示例
directory = 'your_directory_path'
new_prefix = 'new_prefix'
rename_files_in_directory(directory, new_prefix)
六、进一步优化和扩展
1、处理文件和目录的区分
在实际应用中,目录下不仅可能有文件,还有子目录。我们可以通过os.path.isfile
来区分文件和目录,只对文件进行重命名操作。
for file in files:
old_file_path = os.path.join(directory, file)
if os.path.isfile(old_file_path):
try:
if len(file) > 4:
new_name = 'new_prefix' + file[4:]
new_file_path = os.path.join(directory, new_name)
os.rename(old_file_path, new_file_path)
print(f"Renamed {file} to {new_name}")
except Exception as e:
print(f"Error renaming file {file}: {e}")
2、处理不同文件类型
有时我们可能只想修改特定类型的文件,例如只修改.txt
文件名。我们可以通过检查文件扩展名来实现这一点。
for file in files:
old_file_path = os.path.join(directory, file)
if os.path.isfile(old_file_path) and file.endswith('.txt'):
try:
if len(file) > 4:
new_name = 'new_prefix' + file[4:]
new_file_path = os.path.join(directory, new_name)
os.rename(old_file_path, new_file_path)
print(f"Renamed {file} to {new_name}")
except Exception as e:
print(f"Error renaming file {file}: {e}")
3、日志记录
为了更好地管理和监控文件重命名过程,我们可以添加日志记录,将重命名的文件信息记录到日志文件中。
import logging
设置日志配置
logging.basicConfig(filename='rename_log.txt', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
for file in files:
old_file_path = os.path.join(directory, file)
if os.path.isfile(old_file_path):
try:
if len(file) > 4:
new_name = 'new_prefix' + file[4:]
new_file_path = os.path.join(directory, new_name)
os.rename(old_file_path, new_file_path)
logging.info(f"Renamed {file} to {new_name}")
except Exception as e:
logging.error(f"Error renaming file {file}: {e}")
七、总结
通过以上步骤,我们详细讲解了如何使用Python修改文件名前4个字符串的具体方法。关键步骤包括导入必要库、获取文件列表、修改文件名前4个字符、处理文件名冲突和异常、进一步优化和扩展。希望这些内容能够帮助你更好地理解和实现文件重命名任务。如果你有其他需求或遇到问题,欢迎进一步探讨。
相关问答FAQs:
如何在Python中获取文件名的前四个字符?
在Python中,可以使用os
模块来获取文件名并提取前四个字符。首先,使用os.listdir()
获取目录中的文件列表,然后通过字符串切片获取文件名的前四个字符。例如:
import os
file_name = 'example.txt'
first_four_chars = file_name[:4]
print(first_four_chars)
这个代码将输出exam
,这是文件名的前四个字符。
在修改文件名时,如何确保文件路径的正确性?
为了确保在修改文件名时路径的正确性,建议使用os.path
模块来处理文件路径。可以使用os.path.join()
来组合目录和文件名,并使用os.rename()
来重命名文件。这样可以避免因路径问题导致的错误。例如:
import os
old_name = 'path/to/example.txt'
new_name = 'path/to/new_example.txt'
os.rename(old_name, new_name)
这种方法能有效避免路径错误。
是否可以批量修改多个文件的前四个字符?
是的,可以通过循环遍历目录中的所有文件,针对每个文件的名称进行修改。使用os.listdir()
获取文件列表后,可以结合字符串操作和os.rename()
进行批量修改。以下是一个示例代码:
import os
directory = 'path/to/directory'
for file_name in os.listdir(directory):
if file_name.endswith('.txt'):
new_name = 'new_' + file_name[4:] # 修改前四个字符
os.rename(os.path.join(directory, file_name), os.path.join(directory, new_name))
此代码将目录中所有以.txt
结尾的文件的前四个字符替换为new_
。
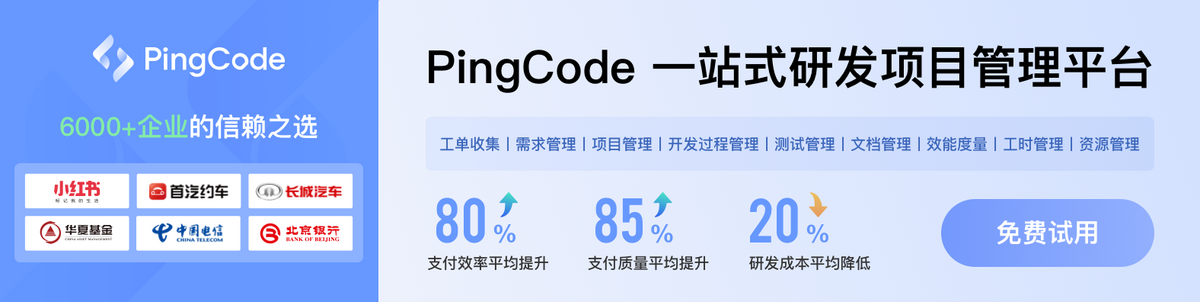