在Python中,将格式化字符串转换成字典的核心方法是:使用json.loads()
、使用正则表达式解析、使用split()
和strip()
方法。其中,使用json.loads()
是最常见的方法,因为它能够自动处理大多数简单的格式化字符串,并将其转换为字典形式。
使用json.loads()
可以非常方便地将格式化字符串转换成字典形式。这个方法依赖于Python的内置json
模块,它能够解析符合JSON格式的字符串并将其转换为Python字典。下面我们将详细描述如何使用这些方法来实现这一任务。
一、使用json.loads()
方法
1、导入json
模块
首先,我们需要导入Python的json
模块。这个模块包含了我们将要使用的loads
函数,它能够将JSON格式的字符串解析为字典。
import json
2、将格式化字符串转换为字典
假设我们有一个格式化字符串,类似于以下内容:
formatted_string = '{"name": "John", "age": 30, "city": "New York"}'
我们可以使用json.loads()
方法将其转换为字典:
data_dict = json.loads(formatted_string)
print(data_dict)
输出将会是:
{'name': 'John', 'age': 30, 'city': 'New York'}
3、处理复杂的JSON字符串
有时候,字符串可能包含嵌套的JSON对象。json.loads()
同样可以处理这些复杂的字符串。例如:
formatted_string = '{"name": "John", "age": 30, "address": {"city": "New York", "zipcode": "10001"}}'
data_dict = json.loads(formatted_string)
print(data_dict)
输出将会是:
{'name': 'John', 'age': 30, 'address': {'city': 'New York', 'zipcode': '10001'}}
二、使用正则表达式解析
1、导入re
模块
正则表达式可以用来手动解析复杂的字符串。首先,我们需要导入re
模块:
import re
2、定义解析函数
我们可以定义一个函数,使用正则表达式解析格式化字符串并将其转换为字典:
def parse_to_dict(formatted_string):
pairs = re.findall(r'\"(.*?)\"\s*:\s*\"(.*?)\"', formatted_string)
return {key: value for key, value in pairs}
3、解析字符串
使用我们定义的函数解析格式化字符串:
formatted_string = '{"name": "John", "age": "30", "city": "New York"}'
data_dict = parse_to_dict(formatted_string)
print(data_dict)
输出将会是:
{'name': 'John', 'age': '30', 'city': 'New York'}
三、使用split()
和strip()
方法
1、定义解析函数
我们还可以通过手动分割字符串并去除空白字符来解析格式化字符串。定义一个解析函数:
def split_to_dict(formatted_string):
items = formatted_string.strip('{}').split(',')
data_dict = {}
for item in items:
key, value = item.split(':')
data_dict[key.strip().strip('"')] = value.strip().strip('"')
return data_dict
2、解析字符串
使用我们的解析函数:
formatted_string = '{"name": "John", "age": "30", "city": "New York"}'
data_dict = split_to_dict(formatted_string)
print(data_dict)
输出将会是:
{'name': 'John', 'age': '30', 'city': 'New York'}
四、处理复杂情况
1、处理嵌套结构
在一些情况下,字符串可能包含嵌套的结构。我们需要递归地解析这些嵌套的部分。例如:
def recursive_parse(json_string):
def inner_parse(s):
items = re.findall(r'\"(.*?)\"\s*:\s*(\{.*?\}|\".*?\"|\d+)', s)
result = {}
for key, value in items:
if value.startswith('{') and value.endswith('}'):
result[key] = inner_parse(value[1:-1])
else:
result[key] = value.strip('"')
return result
return inner_parse(json_string)
2、解析复杂字符串
使用我们的递归解析函数:
formatted_string = '{"name": "John", "age": 30, "address": {"city": "New York", "zipcode": "10001"}}'
data_dict = recursive_parse(formatted_string)
print(data_dict)
输出将会是:
{'name': 'John', 'age': '30', 'address': {'city': 'New York', 'zipcode': '10001'}}
五、错误处理
1、捕获解析错误
在实际应用中,字符串可能包含错误或不完整的部分。我们需要捕获这些错误并进行处理。例如:
import json
def safe_parse(json_string):
try:
return json.loads(json_string)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
return None
2、处理错误的字符串
尝试解析错误的字符串:
formatted_string = '{"name": "John", "age": 30, "city": "New York"' # Missing closing brace
data_dict = safe_parse(formatted_string)
print(data_dict) # Output will be None and print an error message
总结
在Python中,将格式化字符串转换成字典的主要方法有:使用json.loads()
、正则表达式解析、split()
和strip()
方法。使用json.loads()
是最常见和最简单的方法,适用于大多数符合JSON格式的字符串。对于复杂或不标准的字符串,可以使用正则表达式或手动解析的方法。处理嵌套结构和错误处理是解析格式化字符串时需要特别注意的两个方面。通过灵活运用这些方法,我们可以高效地将各种格式化字符串转换为Python字典,并在实际应用中加以利用。
相关问答FAQs:
如何在Python中将格式化字符串转换为字典?
在Python中,可以使用json
模块来将格式化字符串转换为字典。首先,确保字符串的格式符合JSON标准,例如使用双引号包围字符串。然后,使用json.loads()
函数进行转换。例如:
import json
formatted_string = '{"name": "Alice", "age": 30, "city": "New York"}'
dictionary = json.loads(formatted_string)
print(dictionary)
这样就可以得到一个字典对象。
格式化字符串的哪些特征使其适合转换为字典?
适合转换为字典的格式化字符串通常具有键值对的结构,键和值之间用冒号:
分隔,多个键值对之间用逗号,
分隔,并且所有的键和字符串值都应当使用双引号或单引号包围。确保遵循JSON格式规范是成功转换的关键。
如果格式化字符串不符合JSON标准,如何处理?
在处理不符合JSON标准的格式化字符串时,可以手动修改其格式,使其符合要求。你可以使用字符串的替换方法或者正则表达式来调整字符串结构。也可以编写自定义解析函数,将格式化字符串解析成字典,这需要对字符串的结构有深入理解。
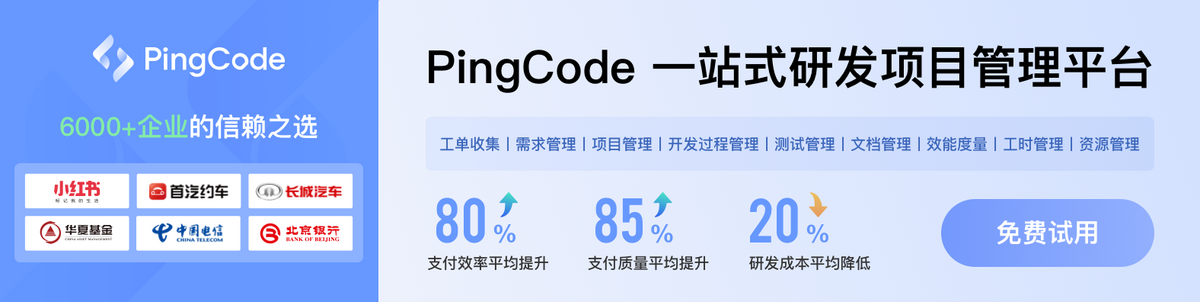