在Python中提取字符串中的数字,可以使用多种方法,如正则表达式、列表解析、字符串方法等。本文将详细探讨这些方法,并提供具体的代码示例。其中,最常用且最灵活的方法是使用正则表达式,因为它能够匹配复杂的字符串模式。下面将详细介绍正则表达式的应用。
一、正则表达式提取数字
正则表达式(Regular Expressions, regex)是一种强大的字符串匹配工具,在Python中可以通过re
模块来使用。正则表达式允许我们定义复杂的模式,并在字符串中搜索这些模式。以下是使用正则表达式提取字符串中所有数字的示例:
import re
def extract_numbers(text):
# 定义一个正则表达式模式,用于匹配数字
pattern = r'\d+'
# 使用findall方法找到所有匹配的数字
numbers = re.findall(pattern, text)
return numbers
text = "The price is 45 dollars, and the discount is 5 dollars."
numbers = extract_numbers(text)
print(numbers) # 输出: ['45', '5']
在上面的例子中,正则表达式模式\d+
用于匹配一个或多个数字字符。re.findall
方法返回所有匹配的子字符串组成的列表。
二、列表解析提取数字
列表解析是Python中一种简洁且高效的列表生成方式。我们可以结合字符串的isdigit
方法来提取字符串中的所有数字。尽管这种方法不如正则表达式灵活,但对于简单的需求非常有效。
def extract_numbers(text):
# 使用列表解析和isdigit方法提取所有数字字符
numbers = [char for char in text if char.isdigit()]
return numbers
text = "The price is 45 dollars, and the discount is 5 dollars."
numbers = extract_numbers(text)
print(numbers) # 输出: ['4', '5', '5']
在这个例子中,我们遍历字符串的每个字符,并使用isdigit
方法检查它是否是一个数字字符。如果是,将其添加到列表中。
三、字符串方法提取数字
除了正则表达式和列表解析,我们还可以使用字符串方法来提取数字。例如,使用字符串的split
方法结合列表解析,可以提取包含数字的子字符串。
def extract_numbers(text):
# 使用split方法按空格分割字符串,并检查每个子字符串是否包含数字
words = text.split()
numbers = [word for word in words if any(char.isdigit() for char in word)]
return numbers
text = "The price is 45 dollars, and the discount is 5 dollars."
numbers = extract_numbers(text)
print(numbers) # 输出: ['45', '5']
在这个示例中,我们首先使用split
方法将字符串按空格分割成单词,然后使用列表解析检查每个单词是否包含数字字符。
四、使用filter
和lambda
函数
Python的filter
函数结合lambda
表达式也可以用于提取字符串中的数字。filter
函数可以在提供的可迭代对象中,筛选出满足条件的元素。
def extract_numbers(text):
# 使用filter和lambda表达式筛选出数字字符
numbers = list(filter(lambda char: char.isdigit(), text))
return numbers
text = "The price is 45 dollars, and the discount is 5 dollars."
numbers = extract_numbers(text)
print(numbers) # 输出: ['4', '5', '5']
在这个例子中,filter
函数会遍历字符串的每个字符,并使用lambda
表达式检查它是否是数字字符。
五、将提取的数字转换为整数或浮点数
在许多情况下,我们需要将提取的数字转换为整数或浮点数以进行进一步的计算。以下是如何将提取的数字转换为整数列表的示例:
import re
def extract_numbers(text):
pattern = r'\d+'
numbers = re.findall(pattern, text)
# 将提取的数字字符串转换为整数
numbers = [int(num) for num in numbers]
return numbers
text = "The price is 45 dollars, and the discount is 5 dollars."
numbers = extract_numbers(text)
print(numbers) # 输出: [45, 5]
在这个例子中,我们使用列表解析将数字字符串转换为整数。类似地,如果要处理浮点数,可以使用float
函数。
六、处理复杂的字符串模式
有时,我们需要提取不仅仅是单纯的数字,还包括带有小数点或负号的数字。我们可以使用更复杂的正则表达式模式来处理这些情况。
import re
def extract_numbers(text):
# 匹配整数和浮点数,包括负数
pattern = r'-?\d+\.?\d*'
numbers = re.findall(pattern, text)
# 将提取的数字字符串转换为浮点数
numbers = [float(num) for num in numbers]
return numbers
text = "The price is -45.50 dollars, and the discount is 5.25 dollars."
numbers = extract_numbers(text)
print(numbers) # 输出: [-45.5, 5.25]
在这个例子中,正则表达式模式-?\d+\.?\d*
用于匹配可能带有负号的小数或整数。
七、处理包含单位的数字
在某些情况下,数字可能包含单位,例如"45kg"或"5m"。我们可以使用正则表达式来提取数字并忽略单位。
import re
def extract_numbers(text):
# 匹配带单位的数字
pattern = r'(\d+\.?\d*)\s*(kg|m|cm|mm)?'
matches = re.findall(pattern, text)
# 只提取数字部分
numbers = [float(match[0]) for match in matches if match[0]]
return numbers
text = "The weights are 45kg and 5.5m."
numbers = extract_numbers(text)
print(numbers) # 输出: [45.0, 5.5]
在这个示例中,我们使用正则表达式模式(\d+\.?\d*)\s*(kg|m|cm|mm)?
匹配带单位的数字,并提取数字部分。
八、实战应用:从文件中提取数字
在实际应用中,可能需要从文件中提取数字。以下是如何从文本文件中提取所有数字的示例:
import re
def extract_numbers_from_file(file_path):
with open(file_path, 'r') as file:
text = file.read()
pattern = r'\d+'
numbers = re.findall(pattern, text)
numbers = [int(num) for num in numbers]
return numbers
file_path = 'data.txt'
numbers = extract_numbers_from_file(file_path)
print(numbers)
在这个例子中,我们读取文件内容,并使用正则表达式提取数字。
九、总结
在Python中提取字符串中的数字有多种方法,包括正则表达式、列表解析、字符串方法、filter
和lambda
函数等。正则表达式是最灵活和强大的方法,适用于复杂的字符串模式。根据具体需求,可以选择最适合的方法进行数字提取。
无论是处理简单的数字提取,还是处理复杂的字符串模式,Python都提供了强大的工具和方法来满足各种需求。通过合理选择和组合这些方法,可以高效地完成数字提取任务。
相关问答FAQs:
如何在Python中提取字符串中的所有数字?
在Python中,可以使用正则表达式来提取字符串中的所有数字。可以借助re
模块,通过re.findall()
函数来实现。例如,re.findall(r'\d+', your_string)
将返回一个列表,包含字符串中的所有数字。
如果我只想提取字符串中的第一个数字,该怎么做?
如果只需要提取字符串中的第一个数字,可以使用re.search()
函数来实现。使用re.search(r'\d+', your_string)
将返回一个匹配对象,从中提取第一个数字。如果匹配成功,可以通过match.group()
方法获取该数字。
在提取数字时,我需要考虑负数和小数吗?
是的,如果需要提取负数或小数,可以使用更复杂的正则表达式。例如,要提取负数和小数,可以使用re.findall(r'-?\d+\.?\d*', your_string)
。这个表达式可以匹配带有负号的整数、小数和正整数。这样可以确保从字符串中提取出所有的数字形式。
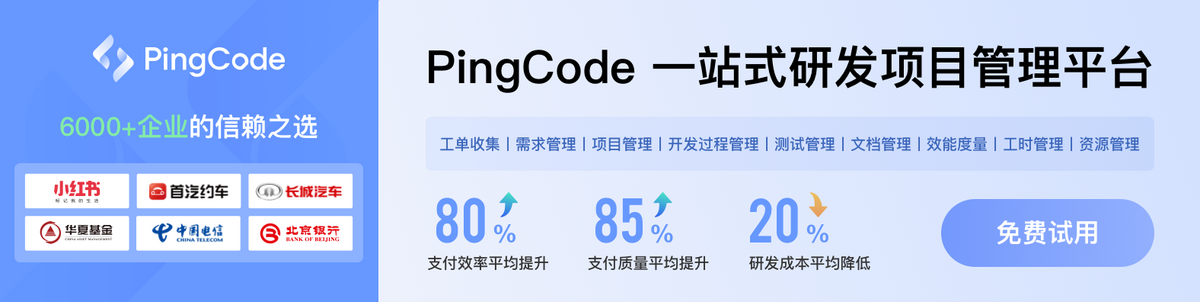