在Python项目中,配置管理对于项目的稳定性和可维护性至关重要。可以通过使用环境变量、配置文件、命令行参数和配置管理工具来改Python项目配置。其中,使用配置文件是最常见的方法。使用配置文件能够将配置与代码分离、便于版本控制、增强灵活性。下面详细介绍如何使用配置文件来改Python项目配置。
一、配置文件的使用
配置文件可以使用多种格式,比如INI、YAML、JSON、TOML等。每种格式都有其优点和适用场景。INI文件简单易读,适用于小型项目;YAML和JSON适合复杂配置,结构化且支持嵌套;TOML用于高性能需求下的配置。
1、INI文件
a. 创建INI文件
INI文件使用“键=值”对来存储配置,文件名通常以“.ini”结尾。创建一个名为config.ini
的文件:
[database]
host = localhost
port = 3306
user = root
password = root
[application]
debug = True
secret_key = your_secret_key
b. 读取INI文件
Python标准库提供了configparser
模块来读取INI文件。下面是一个示例代码:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
db_host = config['database']['host']
db_port = config['database'].getint('port')
db_user = config['database']['user']
db_password = config['database']['password']
app_debug = config['application'].getboolean('debug')
app_secret_key = config['application']['secret_key']
print(f"Database Host: {db_host}")
print(f"Database Port: {db_port}")
print(f"Application Debug Mode: {app_debug}")
2、YAML文件
a. 创建YAML文件
YAML文件结构化且支持嵌套,文件名通常以“.yaml”或“.yml”结尾。创建一个名为config.yaml
的文件:
database:
host: localhost
port: 3306
user: root
password: root
application:
debug: true
secret_key: your_secret_key
b. 读取YAML文件
Python的PyYAML
库可以读取YAML文件。首先安装PyYAML
库:
pip install pyyaml
然后使用下面的代码读取YAML文件:
import yaml
with open('config.yaml', 'r') as file:
config = yaml.safe_load(file)
db_host = config['database']['host']
db_port = config['database']['port']
db_user = config['database']['user']
db_password = config['database']['password']
app_debug = config['application']['debug']
app_secret_key = config['application']['secret_key']
print(f"Database Host: {db_host}")
print(f"Database Port: {db_port}")
print(f"Application Debug Mode: {app_debug}")
3、JSON文件
a. 创建JSON文件
JSON文件广泛应用于配置管理,文件名通常以“.json”结尾。创建一个名为config.json
的文件:
{
"database": {
"host": "localhost",
"port": 3306,
"user": "root",
"password": "root"
},
"application": {
"debug": true,
"secret_key": "your_secret_key"
}
}
b. 读取JSON文件
Python标准库提供了json
模块来读取JSON文件。下面是一个示例代码:
import json
with open('config.json', 'r') as file:
config = json.load(file)
db_host = config['database']['host']
db_port = config['database']['port']
db_user = config['database']['user']
db_password = config['database']['password']
app_debug = config['application']['debug']
app_secret_key = config['application']['secret_key']
print(f"Database Host: {db_host}")
print(f"Database Port: {db_port}")
print(f"Application Debug Mode: {app_debug}")
4、TOML文件
a. 创建TOML文件
TOML文件简单易读且具有较高的解析性能,文件名通常以“.toml”结尾。创建一个名为config.toml
的文件:
[database]
host = "localhost"
port = 3306
user = "root"
password = "root"
[application]
debug = true
secret_key = "your_secret_key"
b. 读取TOML文件
Python的toml
库可以读取TOML文件。首先安装toml
库:
pip install toml
然后使用下面的代码读取TOML文件:
import toml
config = toml.load('config.toml')
db_host = config['database']['host']
db_port = config['database']['port']
db_user = config['database']['user']
db_password = config['database']['password']
app_debug = config['application']['debug']
app_secret_key = config['application']['secret_key']
print(f"Database Host: {db_host}")
print(f"Database Port: {db_port}")
print(f"Application Debug Mode: {app_debug}")
二、环境变量的使用
环境变量是另一种常见的配置管理方式,适用于敏感数据和不同环境下的配置。通过环境变量,可以在不修改代码的情况下更改配置。
1、设置环境变量
在操作系统中设置环境变量,以Linux为例:
export DATABASE_HOST=localhost
export DATABASE_PORT=3306
export DATABASE_USER=root
export DATABASE_PASSWORD=root
export APPLICATION_DEBUG=true
export APPLICATION_SECRET_KEY=your_secret_key
2、读取环境变量
Python标准库提供了os
模块来读取环境变量。下面是一个示例代码:
import os
db_host = os.getenv('DATABASE_HOST')
db_port = int(os.getenv('DATABASE_PORT'))
db_user = os.getenv('DATABASE_USER')
db_password = os.getenv('DATABASE_PASSWORD')
app_debug = os.getenv('APPLICATION_DEBUG').lower() == 'true'
app_secret_key = os.getenv('APPLICATION_SECRET_KEY')
print(f"Database Host: {db_host}")
print(f"Database Port: {db_port}")
print(f"Application Debug Mode: {app_debug}")
三、命令行参数的使用
命令行参数适用于需要灵活配置的场景,通过命令行参数,可以在运行时动态传递配置。
1、解析命令行参数
Python标准库提供了argparse
模块来解析命令行参数。下面是一个示例代码:
import argparse
parser = argparse.ArgumentParser(description='Project Configuration')
parser.add_argument('--db-host', type=str, required=True, help='Database Host')
parser.add_argument('--db-port', type=int, required=True, help='Database Port')
parser.add_argument('--db-user', type=str, required=True, help='Database User')
parser.add_argument('--db-password', type=str, required=True, help='Database Password')
parser.add_argument('--app-debug', type=bool, required=True, help='Application Debug Mode')
parser.add_argument('--app-secret-key', type=str, required=True, help='Application Secret Key')
args = parser.parse_args()
db_host = args.db_host
db_port = args.db_port
db_user = args.db_user
db_password = args.db_password
app_debug = args.app_debug
app_secret_key = args.app_secret_key
print(f"Database Host: {db_host}")
print(f"Database Port: {db_port}")
print(f"Application Debug Mode: {app_debug}")
运行命令:
python script.py --db-host localhost --db-port 3306 --db-user root --db-password root --app-debug true --app-secret-key your_secret_key
四、配置管理工具的使用
配置管理工具能够更好地管理和维护配置,常用的工具有ConfigParser
、Cerberus
、Pydantic
等。
1、使用ConfigParser
ConfigParser是Python标准库提供的工具,适用于简单的配置管理。
a. 安装ConfigParser
ConfigParser是Python标准库,不需要额外安装。
b. 使用ConfigParser
import configparser
config = configparser.ConfigParser()
config['database'] = {
'host': 'localhost',
'port': 3306,
'user': 'root',
'password': 'root'
}
config['application'] = {
'debug': True,
'secret_key': 'your_secret_key'
}
with open('config.ini', 'w') as configfile:
config.write(configfile)
2、使用Cerberus
Cerberus是一个轻量级的数据验证工具,适用于配置验证。
a. 安装Cerberus
pip install cerberus
b. 使用Cerberus
from cerberus import Validator
schema = {
'database': {
'type': 'dict',
'schema': {
'host': {'type': 'string', 'required': True},
'port': {'type': 'integer', 'required': True},
'user': {'type': 'string', 'required': True},
'password': {'type': 'string', 'required': True}
}
},
'application': {
'type': 'dict',
'schema': {
'debug': {'type': 'boolean', 'required': True},
'secret_key': {'type': 'string', 'required': True}
}
}
}
config = {
'database': {
'host': 'localhost',
'port': 3306,
'user': 'root',
'password': 'root'
},
'application': {
'debug': True,
'secret_key': 'your_secret_key'
}
}
v = Validator(schema)
if v.validate(config):
print("Configuration is valid")
else:
print("Configuration is invalid")
print(v.errors)
3、使用Pydantic
Pydantic是一个基于数据模型的验证库,适用于复杂配置管理。
a. 安装Pydantic
pip install pydantic
b. 使用Pydantic
from pydantic import BaseModel, Field, ValidationError
class DatabaseConfig(BaseModel):
host: str
port: int
user: str
password: str
class ApplicationConfig(BaseModel):
debug: bool
secret_key: str
class Config(BaseModel):
database: DatabaseConfig
application: ApplicationConfig
config_data = {
'database': {
'host': 'localhost',
'port': 3306,
'user': 'root',
'password': 'root'
},
'application': {
'debug': True,
'secret_key': 'your_secret_key'
}
}
try:
config = Config(config_data)
print("Configuration is valid")
except ValidationError as e:
print("Configuration is invalid")
print(e.json())
五、总结
以上介绍了使用配置文件、环境变量、命令行参数和配置管理工具来管理Python项目配置的方法。使用配置文件能够将配置与代码分离、便于版本控制、增强灵活性,是最常见的方法。具体选择哪种方法取决于项目需求和复杂度。在实际开发中,可以综合使用多种方法,以确保配置管理的灵活性和可靠性。
相关问答FAQs:
如何在Python项目中更改配置文件的格式?
在Python项目中,配置文件通常使用JSON、YAML或INI格式。要更改配置文件的格式,可以创建一个新的文件并将现有配置转换为所需格式。使用Python的内置库,如json
、yaml
或configparser
,可以轻松读取和写入不同格式的配置文件。确保在代码中相应地修改读取配置的部分,以适应新的文件格式。
如何动态加载Python项目中的配置?
动态加载配置意味着在程序运行时根据需要更改配置。可以使用环境变量、命令行参数或外部配置管理工具(如dotenv或configparser)来实现这一点。通过这种方式,您可以避免硬编码配置在项目中,使其更加灵活和易于管理。
如何确保Python项目的配置安全?
保护配置文件尤其重要,特别是当它们包含敏感信息(如API密钥或数据库凭证)时。可以考虑将敏感信息存储在环境变量中,而不是直接在配置文件中。使用Python的os
库可以轻松访问这些环境变量。此外,确保配置文件的权限设置正确,避免未授权的访问也是非常重要的。
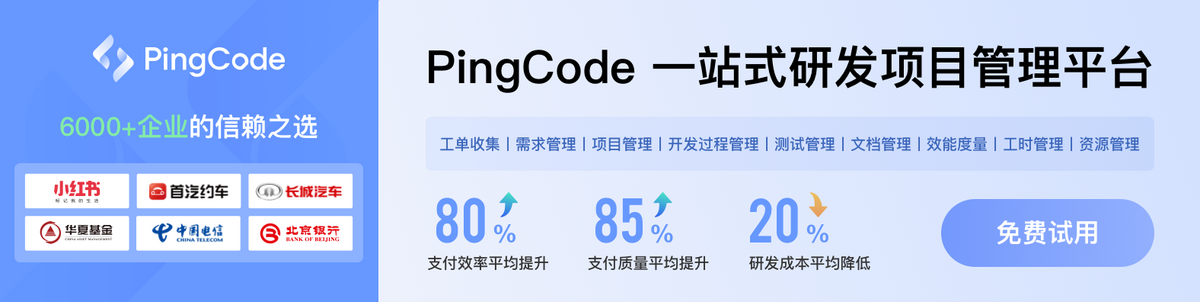