爬取淘宝数据的方法包括使用Selenium、使用Scrapy、使用Requests和BeautifulSoup、使用Pyppeteer。使用Selenium是最常见的方法之一,因为它可以模拟浏览器行为,解决反爬机制。在这一方法中,Selenium通过模拟用户操作,可以自动化整个浏览过程,加载JavaScript内容。以下是详细描述使用Selenium爬取淘宝数据的方法。
使用Selenium爬取淘宝数据需要安装Selenium库,并且下载与浏览器版本匹配的WebDriver。Selenium允许模拟用户操作,如点击、输入等,使得爬虫能够处理JavaScript动态加载内容。使用Selenium时,可以设置WebDriver的等待策略,使其在页面完全加载后再进行数据提取,从而提高爬虫的稳定性和准确性。
一、安装和配置
1. 安装Selenium
首先,你需要安装Selenium库。可以通过pip命令来安装:
pip install selenium
2. 下载WebDriver
Selenium需要一个WebDriver来控制浏览器。常用的WebDriver包括ChromeDriver(用于Google Chrome)和GeckoDriver(用于Mozilla Firefox)。下载WebDriver后,将其路径添加到系统环境变量中,或者在代码中指定路径。
3. 配置环境
确保浏览器和WebDriver版本匹配,这样可以避免兼容性问题。在代码中,可以通过设置选项来定制WebDriver的行为,比如无头模式、禁用图片加载等,以提高爬取效率。
二、使用Selenium爬取淘宝数据
1. 初始化WebDriver
首先,导入Selenium库,并初始化WebDriver:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
配置Chrome浏览器选项
chrome_options = Options()
chrome_options.add_argument('--headless') # 无头模式
chrome_options.add_argument('--disable-gpu') # 禁用GPU加速
chrome_options.add_argument('--no-sandbox') # 禁用沙盒模式
初始化Chrome WebDriver
driver = webdriver.Chrome(options=chrome_options)
2. 访问淘宝页面
使用WebDriver访问淘宝页面,并等待页面加载完成:
# 访问淘宝首页
url = "https://www.taobao.com"
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10) # 隐式等待,最长等待时间为10秒
3. 模拟用户操作
为了获取搜索结果,需要模拟用户在搜索框中输入关键词,并点击搜索按钮:
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
找到搜索框并输入关键词
search_box = driver.find_element(By.ID, "q")
search_box.send_keys("手机")
模拟点击搜索按钮
search_box.send_keys(Keys.RETURN)
等待搜索结果加载完成
driver.implicitly_wait(10)
4. 提取数据
在搜索结果页面中,提取商品信息,包括商品标题、价格、链接等:
# 提取商品信息
items = driver.find_elements(By.CSS_SELECTOR, ".item")
for item in items:
title = item.find_element(By.CSS_SELECTOR, ".title").text
price = item.find_element(By.CSS_SELECTOR, ".price").text
link = item.find_element(By.CSS_SELECTOR, ".link").get_attribute("href")
print(f"Title: {title}")
print(f"Price: {price}")
print(f"Link: {link}")
print("---------------")
5. 关闭WebDriver
完成数据提取后,关闭WebDriver:
driver.quit()
三、处理反爬机制
1. 添加头部信息
为了避免被反爬机制检测,可以在请求中添加头部信息,模拟真实用户访问:
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
caps = DesiredCapabilities().CHROME
caps["chrome.page.settings.userAgent"] = "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
driver = webdriver.Chrome(desired_capabilities=caps)
2. 设置代理
使用代理IP可以避免因频繁访问而被封禁:
from selenium.webdriver.common.proxy import Proxy, ProxyType
proxy = Proxy()
proxy.proxy_type = ProxyType.MANUAL
proxy.http_proxy = "http://your.proxy.ip:port"
proxy.ssl_proxy = "http://your.proxy.ip:port"
capabilities = webdriver.DesiredCapabilities.CHROME
proxy.add_to_capabilities(capabilities)
driver = webdriver.Chrome(desired_capabilities=capabilities)
3. 随机延迟
在每次请求之间添加随机延迟,模拟正常用户行为:
import time
import random
随机延迟
time.sleep(random.uniform(1, 3))
4. 处理验证码
有时,淘宝会要求输入验证码。可以通过Selenium截图功能,保存验证码图片,并使用OCR识别工具(如Tesseract)进行识别:
from PIL import Image
import pytesseract
截取验证码图片
captcha_image = driver.find_element(By.ID, "captcha_image")
captcha_image.screenshot("captcha.png")
识别验证码
captcha_text = pytesseract.image_to_string(Image.open("captcha.png"))
print(f"Captcha: {captcha_text}")
四、保存和处理数据
1. 保存数据到CSV文件
提取的数据可以保存到CSV文件中,便于后续分析:
import csv
打开CSV文件
with open("taobao_data.csv", "w", newline="", encoding="utf-8") as csvfile:
writer = csv.writer(csvfile)
writer.writerow(["Title", "Price", "Link"])
# 写入数据
for item in items:
title = item.find_element(By.CSS_SELECTOR, ".title").text
price = item.find_element(By.CSS_SELECTOR, ".price").text
link = item.find_element(By.CSS_SELECTOR, ".link").get_attribute("href")
writer.writerow([title, price, link])
2. 数据清洗与分析
爬取的数据可能存在冗余或不一致,需要进行清洗与规范化处理。可以使用Pandas库进行数据清洗和分析:
import pandas as pd
读取CSV文件
data = pd.read_csv("taobao_data.csv")
数据清洗与处理
data.drop_duplicates(inplace=True)
data["Price"] = data["Price"].str.replace("¥", "").astype(float)
数据分析
print(data.describe())
3. 可视化数据
使用Matplotlib或Seaborn库对数据进行可视化展示:
import matplotlib.pyplot as plt
import seaborn as sns
绘制价格分布图
plt.figure(figsize=(10, 6))
sns.histplot(data["Price"], bins=30, kde=True)
plt.title("Price Distribution")
plt.xlabel("Price")
plt.ylabel("Frequency")
plt.show()
五、扩展与优化
1. 分布式爬虫
在爬取大规模数据时,可以使用Scrapy框架和分布式爬虫技术(如Scrapy-Redis)来提高爬取效率和处理能力:
from scrapy import Spider
from scrapy_redis.spiders import RedisSpider
class TaobaoSpider(RedisSpider):
name = "taobao"
redis_key = "taobao:start_urls"
def parse(self, response):
for item in response.css(".item"):
yield {
"title": item.css(".title::text").get(),
"price": item.css(".price::text").get(),
"link": item.css(".link::attr(href)").get(),
}
2. 使用Pyppeteer
Pyppeteer是一个与Selenium类似的库,但它是基于Puppeteer的Python实现,具有更强的JavaScript处理能力:
import asyncio
from pyppeteer import launch
async def main():
browser = await launch(headless=True)
page = await browser.newPage()
await page.goto("https://www.taobao.com")
# 输入关键词并搜索
await page.type("#q", "手机")
await page.click(".btn-search")
await page.waitForSelector(".item")
# 提取数据
items = await page.querySelectorAll(".item")
for item in items:
title = await page.evaluate('(element) => element.querySelector(".title").innerText', item)
price = await page.evaluate('(element) => element.querySelector(".price").innerText', item)
link = await page.evaluate('(element) => element.querySelector(".link").href', item)
print(f"Title: {title}")
print(f"Price: {price}")
print(f"Link: {link}")
print("---------------")
await browser.close()
asyncio.get_event_loop().run_until_complete(main())
3. 使用API
如果淘宝提供官方API,可以通过API获取数据,这样可以避免反爬机制,提高爬取效率和稳定性:
import requests
api_url = "https://api.taobao.com/data"
params = {
"keyword": "手机",
"page": 1,
}
response = requests.get(api_url, params=params)
data = response.json()
for item in data["items"]:
print(f"Title: {item['title']}")
print(f"Price: {item['price']}")
print(f"Link: {item['link']}")
print("---------------")
六、总结
通过以上步骤,可以使用Selenium成功爬取淘宝数据,并处理反爬机制,保存与分析数据。使用Selenium是爬取淘宝数据的常见方法之一,但在实际应用中,还需根据具体情况选择合适的技术和策略,如使用代理IP、处理验证码、数据清洗与分析等。此外,可以考虑使用其他爬虫框架(如Scrapy)或浏览器自动化工具(如Pyppeteer)来提高爬取效率和处理复杂情况。无论选择哪种方法,都需要遵循相关法律法规,确保数据爬取的合法性和合理性。
相关问答FAQs:
如何使用Python爬取淘宝的数据?
使用Python爬取淘宝数据通常涉及几个步骤,包括选择合适的库、设置请求头、解析网页内容等。可以使用requests
库发送HTTP请求,使用BeautifulSoup
或lxml
解析HTML内容。需要注意的是,淘宝有反爬虫机制,建议使用代理IP和设置适当的请求间隔,以降低被封的风险。
爬取淘宝数据需要注意哪些法律法规?
在爬取淘宝数据时,务必遵守相关法律法规,特别是数据隐私和知识产权方面的法律。许多网站的使用条款中明确禁止未经授权的数据抓取行为,因此在进行爬取前,了解并遵守网站的robots.txt文件内容和相关法律是十分重要的。
是否有推荐的Python库用于爬取淘宝数据?
对于爬取淘宝数据,requests
和BeautifulSoup
是两个非常常用的库。Scrapy
也是一个强大的框架,适合进行复杂的数据爬取任务。此外,Selenium
可以用于处理动态加载的数据,尤其是在需要模拟用户操作时非常有用。根据具体需求选择合适的工具,可以提高效率和成功率。
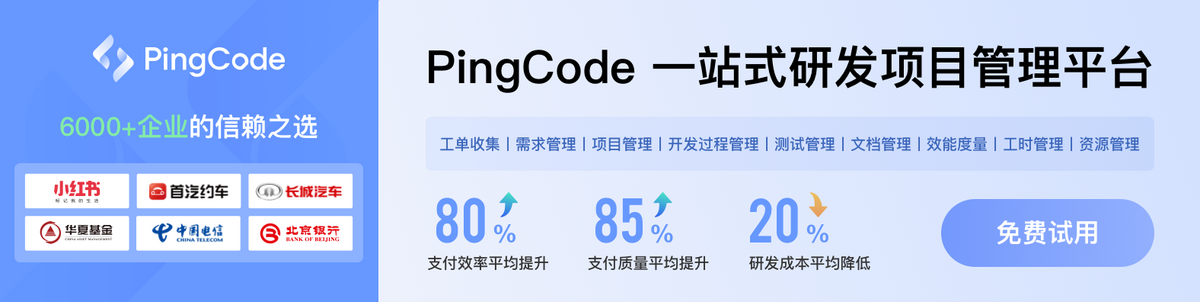