在Python中,可以通过多线程来并行执行多个任务,这对于提高程序的性能特别有用。要在Python中实现多线程,可以使用threading
模块、通过创建Thread对象、定义线程函数。其中使用threading
模块是最常用的方法。例如,threading.Thread
类使得多线程编程变得更加简单。具体步骤包括导入threading模块、定义线程函数、创建Thread对象并启动线程。下面将详细介绍这几种方法。
一、使用threading模块
导入threading模块
首先,需要导入threading模块,这是Python标准库的一部分,不需要额外安装。
import threading
定义线程函数
线程函数是每个线程执行的任务函数。
def print_numbers():
for i in range(5):
print(f"Number: {i}")
创建Thread对象
接下来,创建Thread对象,并将线程函数传递给它。
thread = threading.Thread(target=print_numbers)
启动线程
最后,启动线程使其开始执行。
thread.start()
主线程等待子线程完成
通常情况下,我们需要主线程等待所有子线程完成任务后再继续执行。这可以通过调用join()
方法实现。
thread.join()
以下是一个完整的示例:
import threading
def print_numbers():
for i in range(5):
print(f"Number: {i}")
thread = threading.Thread(target=print_numbers)
thread.start()
thread.join()
二、通过继承Thread类
定义子类
继承threading.Thread
类,重写其__init__
方法和run
方法。
class MyThread(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
print(f"Starting thread {self.name}")
for i in range(5):
print(f"Thread {self.name} Number: {i}")
print(f"Exiting thread {self.name}")
创建并启动线程
创建线程对象,并调用start()
方法启动线程。
thread1 = MyThread("A")
thread2 = MyThread("B")
thread1.start()
thread2.start()
thread1.join()
thread2.join()
完整示例如下:
import threading
class MyThread(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
print(f"Starting thread {self.name}")
for i in range(5):
print(f"Thread {self.name} Number: {i}")
print(f"Exiting thread {self.name}")
thread1 = MyThread("A")
thread2 = MyThread("B")
thread1.start()
thread2.start()
thread1.join()
thread2.join()
三、使用线程池
导入concurrent.futures模块
concurrent.futures
模块提供了一个高级接口来创建和管理线程池。
import concurrent.futures
定义线程函数
定义线程函数,线程池中的每个线程都会执行这个函数。
def print_number(number):
print(f"Number: {number}")
创建线程池并提交任务
创建线程池,并使用submit
方法提交任务。
with concurrent.futures.ThreadPoolExecutor(max_workers=3) as executor:
for i in range(5):
executor.submit(print_number, i)
完整示例如下:
import concurrent.futures
def print_number(number):
print(f"Number: {number}")
with concurrent.futures.ThreadPoolExecutor(max_workers=3) as executor:
for i in range(5):
executor.submit(print_number, i)
四、使用全局解释器锁(GIL)
GIL的影响
Python中的多线程受制于全局解释器锁(GIL),它会保证同一时间只有一个线程在执行Python字节码。这意味着即使是多线程,Python线程的并行执行能力是有限的。
如何应对GIL
为了应对GIL的限制,可以通过以下方法优化多线程程序:
- 多进程:使用
multiprocessing
模块代替threading
模块。 - C扩展:将计算密集型任务用C语言编写,并通过Python调用。
- 异步编程:使用异步编程模型(如
asyncio
)处理I/O密集型任务。
以下是一个使用multiprocessing
模块的示例:
import multiprocessing
def print_numbers():
for i in range(5):
print(f"Number: {i}")
process1 = multiprocessing.Process(target=print_numbers)
process2 = multiprocessing.Process(target=print_numbers)
process1.start()
process2.start()
process1.join()
process2.join()
五、线程间通信
使用Queue模块
线程间通信可以通过queue.Queue
类来实现。这个类提供了一个线程安全的FIFO队列。
创建队列
首先创建一个queue.Queue
对象。
import queue
q = queue.Queue()
将数据放入队列
一个线程将数据放入队列。
q.put(10)
从队列获取数据
另一个线程从队列中获取数据。
data = q.get()
print(data)
完整示例如下:
import threading
import queue
def producer(q):
for i in range(5):
q.put(i)
print(f"Produced: {i}")
def consumer(q):
while not q.empty():
item = q.get()
print(f"Consumed: {item}")
q = queue.Queue()
producer_thread = threading.Thread(target=producer, args=(q,))
consumer_thread = threading.Thread(target=consumer, args=(q,))
producer_thread.start()
consumer_thread.start()
producer_thread.join()
consumer_thread.join()
六、线程同步
使用Lock对象
threading.Lock
对象用于确保线程在访问共享资源时不会产生竞争条件。
创建Lock对象
创建一个threading.Lock
对象。
lock = threading.Lock()
获取锁
线程在访问共享资源之前需要获取锁。
lock.acquire()
try:
# 访问共享资源
finally:
lock.release()
使用上下文管理器
可以使用上下文管理器来自动获取和释放锁。
with lock:
# 访问共享资源
完整示例如下:
import threading
lock = threading.Lock()
counter = 0
def increment_counter():
global counter
with lock:
for _ in range(1000000):
counter += 1
thread1 = threading.Thread(target=increment_counter)
thread2 = threading.Thread(target=increment_counter)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
print(f"Final counter value: {counter}")
七、守护线程
设置守护线程
守护线程在主线程结束时会自动终止。可以通过设置daemon
属性来创建守护线程。
thread = threading.Thread(target=print_numbers)
thread.daemon = True
启动守护线程
启动守护线程与普通线程相同。
thread.start()
以下是一个示例:
import threading
import time
def print_numbers():
for i in range(5):
print(f"Number: {i}")
time.sleep(1)
thread = threading.Thread(target=print_numbers)
thread.daemon = True
thread.start()
time.sleep(2)
print("Main thread exiting")
在这个示例中,守护线程会在主线程退出时自动终止。
八、线程调度
线程优先级
Python的threading
模块不支持直接设置线程优先级。线程调度由操作系统决定。
控制线程执行顺序
可以通过使用事件、信号量等同步原语来控制线程的执行顺序。
以下是一个使用事件的示例:
import threading
event = threading.Event()
def wait_for_event():
print("Waiting for event")
event.wait()
print("Event received")
def set_event():
print("Setting event")
event.set()
wait_thread = threading.Thread(target=wait_for_event)
set_thread = threading.Thread(target=set_event)
wait_thread.start()
set_thread.start()
wait_thread.join()
set_thread.join()
在这个示例中,wait_for_event
线程会等待事件触发,而set_event
线程会触发事件。
九、线程安全的数据结构
使用线程安全的数据结构
Python提供了一些线程安全的数据结构,如queue.Queue
、queue.LifoQueue
、queue.PriorityQueue
等。
示例
以下是一个使用queue.Queue
的示例:
import threading
import queue
def producer(q):
for i in range(5):
q.put(i)
print(f"Produced: {i}")
def consumer(q):
while not q.empty():
item = q.get()
print(f"Consumed: {item}")
q = queue.Queue()
producer_thread = threading.Thread(target=producer, args=(q,))
consumer_thread = threading.Thread(target=consumer, args=(q,))
producer_thread.start()
consumer_thread.start()
producer_thread.join()
consumer_thread.join()
十、性能优化
避免过多的线程
过多的线程会导致上下文切换开销增加,从而降低程序性能。
使用线程池
使用线程池来管理线程,可以减少线程创建和销毁的开销。
尽量避免使用全局变量
尽量避免在线程间共享全局变量,因为这可能会导致竞争条件。
使用合适的同步原语
根据具体情况选择合适的同步原语,如锁、事件、信号量等,以确保线程安全。
优化线程任务
优化线程任务,尽量减少计算密集型操作,避免长时间占用CPU资源。
使用异步编程
对于I/O密集型任务,使用异步编程模型(如asyncio
)可以获得更好的性能。
以下是一个异步编程的示例:
import asyncio
async def print_number(number):
print(f"Number: {number}")
async def main():
tasks = [print_number(i) for i in range(5)]
await asyncio.gather(*tasks)
asyncio.run(main())
在这个示例中,asyncio
库用于异步执行任务,避免了线程切换的开销。
总之,Python提供了多种实现多线程的方法,从基本的threading
模块到高级的线程池和异步编程模型。根据具体需求选择合适的方法,可以有效提升程序的性能。通过合理使用线程间通信、同步原语和线程安全的数据结构,可以确保多线程程序的正确性和稳定性。
相关问答FAQs:
如何在Python中创建线程?
在Python中,创建线程通常使用threading
模块。你可以通过定义一个函数,并使用threading.Thread
类来创建一个新线程。以下是一个简单的示例:
import threading
def print_numbers():
for i in range(5):
print(i)
# 创建线程
thread = threading.Thread(target=print_numbers)
# 启动线程
thread.start()
# 等待线程完成
thread.join()
这个代码段展示了如何定义一个函数并在新线程中执行它。
多线程在Python中有什么优势和劣势?
多线程可以提高程序的响应性,尤其是在处理I/O密集型任务时,比如网络请求或文件操作。然而,由于Python的全局解释器锁(GIL),在CPU密集型任务中,多线程的效果可能不如多进程。此外,线程之间的共享状态可能导致竞争条件和死锁,因此需要谨慎管理共享资源。
如何处理Python多线程中的共享数据?
在多线程中处理共享数据时,可以使用threading.Lock
来确保线程安全。当一个线程访问共享资源时,锁可以阻止其他线程同时访问,从而避免数据冲突。以下是一个示例:
import threading
lock = threading.Lock()
shared_data = 0
def increment():
global shared_data
with lock:
for _ in range(100000):
shared_data += 1
threads = []
for _ in range(2):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(shared_data)
在这个例子中,通过锁确保了对shared_data
的安全访问。
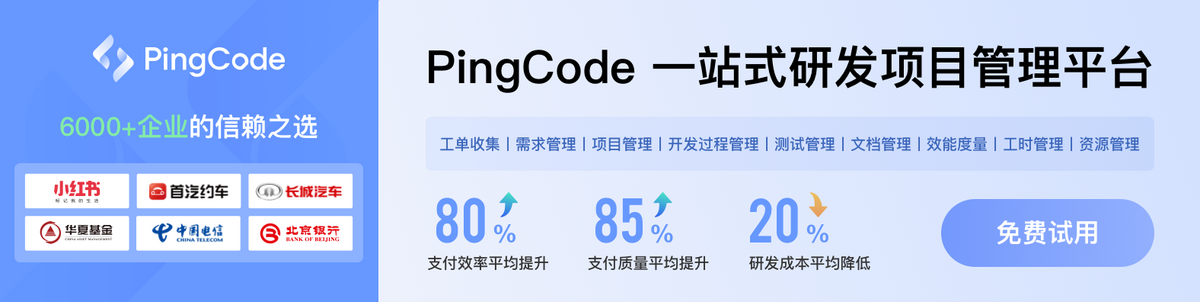