要编写弹窗代码,可以使用 Python 的 Tkinter 库、PyQt 库、Kivy 库等。常用的库有Tkinter、PyQt和Kivy。这里我们将详细讨论如何使用 Tkinter 来创建弹窗,并展示一些代码示例。
一、Tkinter
1. Tkinter库的基础介绍
Tkinter 是 Python 的标准 GUI 库。它提供了一个快速和简洁的方式创建图形用户界面。它是非常适合初学者和小型应用程序的开发。
2. 安装Tkinter
在大多数 Python 安装中,Tkinter 默认是包含的。如果没有,可以通过以下命令安装:
pip install tk
3. 基本的Tkinter弹窗
创建一个基本的 Tkinter 弹窗是非常简单的。以下是一个简单的示例:
import tkinter as tk
from tkinter import messagebox
def show_popup():
messagebox.showinfo("Popup", "This is a popup window")
root = tk.Tk()
root.title("Main Window")
button = tk.Button(root, text="Show Popup", command=show_popup)
button.pack(pady=20)
root.mainloop()
在这个示例中,我们创建了一个基本的窗口,并在其中放置了一个按钮。按下按钮时,将会显示一个弹窗。
4. 自定义弹窗
Tkinter 还允许创建更加复杂的、自定义的弹窗。以下是一个示例:
import tkinter as tk
from tkinter import simpledialog
def show_custom_popup():
user_input = simpledialog.askstring("Input", "Please enter some text:")
if user_input:
tk.messagebox.showinfo("Popup", f"You entered: {user_input}")
root = tk.Tk()
root.title("Main Window")
button = tk.Button(root, text="Show Custom Popup", command=show_custom_popup)
button.pack(pady=20)
root.mainloop()
在这个示例中,我们创建了一个自定义弹窗,它要求用户输入一些文本,并在用户输入后显示输入的内容。
二、PyQt
1. PyQt库的基础介绍
PyQt 是一个功能强大的 GUI 库,基于 Qt 库。它适用于更复杂的 GUI 应用程序开发。
2. 安装PyQt
可以通过以下命令安装 PyQt:
pip install PyQt5
3. 基本的PyQt弹窗
以下是使用 PyQt 创建一个基本弹窗的示例:
from PyQt5.QtWidgets import QApplication, QPushButton, QMessageBox, QWidget
def show_popup():
msg = QMessageBox()
msg.setWindowTitle("Popup")
msg.setText("This is a popup window")
msg.exec_()
app = QApplication([])
window = QWidget()
window.setWindowTitle('Main Window')
button = QPushButton('Show Popup', window)
button.clicked.connect(show_popup)
button.resize(button.sizeHint())
button.move(50, 50)
window.show()
app.exec_()
在这个示例中,我们创建了一个基本的窗口,并在其中放置了一个按钮。按下按钮时,将会显示一个弹窗。
4. 自定义弹窗
PyQt 也允许创建更加复杂的、自定义的弹窗。以下是一个示例:
from PyQt5.QtWidgets import QApplication, QPushButton, QInputDialog, QMessageBox, QWidget
def show_custom_popup():
text, ok = QInputDialog.getText(window, 'Input', 'Please enter some text:')
if ok and text:
QMessageBox.information(window, "Popup", f"You entered: {text}")
app = QApplication([])
window = QWidget()
window.setWindowTitle('Main Window')
button = QPushButton('Show Custom Popup', window)
button.clicked.connect(show_custom_popup)
button.resize(button.sizeHint())
button.move(50, 50)
window.show()
app.exec_()
在这个示例中,我们创建了一个自定义弹窗,它要求用户输入一些文本,并在用户输入后显示输入的内容。
三、Kivy
1. Kivy库的基础介绍
Kivy 是一个开源的 Python 库,用于开发跨平台应用程序和多点触控应用程序。
2. 安装Kivy
可以通过以下命令安装 Kivy:
pip install kivy
3. 基本的Kivy弹窗
以下是使用 Kivy 创建一个基本弹窗的示例:
from kivy.app import App
from kivy.uix.button import Button
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.popup import Popup
from kivy.uix.label import Label
class MainApp(App):
def build(self):
layout = BoxLayout(orientation='vertical')
button = Button(text='Show Popup')
button.bind(on_press=self.show_popup)
layout.add_widget(button)
return layout
def show_popup(self, instance):
content = BoxLayout(orientation='vertical')
content.add_widget(Label(text='This is a popup window'))
popup = Popup(title='Popup', content=content, size_hint=(0.6, 0.4))
popup.open()
if __name__ == '__main__':
MainApp().run()
在这个示例中,我们创建了一个基本的窗口,并在其中放置了一个按钮。按下按钮时,将会显示一个弹窗。
4. 自定义弹窗
Kivy 也允许创建更加复杂的、自定义的弹窗。以下是一个示例:
from kivy.app import App
from kivy.uix.button import Button
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.popup import Popup
from kivy.uix.label import Label
from kivy.uix.textinput import TextInput
class MainApp(App):
def build(self):
layout = BoxLayout(orientation='vertical')
button = Button(text='Show Custom Popup')
button.bind(on_press=self.show_custom_popup)
layout.add_widget(button)
return layout
def show_custom_popup(self, instance):
content = BoxLayout(orientation='vertical')
self.text_input = TextInput(hint_text='Please enter some text')
content.add_widget(self.text_input)
submit_button = Button(text='Submit')
submit_button.bind(on_press=self.submit_text)
content.add_widget(submit_button)
self.popup = Popup(title='Popup', content=content, size_hint=(0.6, 0.4))
self.popup.open()
def submit_text(self, instance):
text = self.text_input.text
if text:
self.popup.dismiss()
popup = Popup(title='Popup', content=Label(text=f'You entered: {text}'), size_hint=(0.6, 0.4))
popup.open()
if __name__ == '__main__':
MainApp().run()
在这个示例中,我们创建了一个自定义弹窗,它要求用户输入一些文本,并在用户输入后显示输入的内容。
四、总结
Tkinter、PyQt和Kivy 都是非常强大的库,可以用来创建各种弹窗和复杂的图形用户界面。选择哪个库取决于您的具体需求和偏好。Tkinter 是最简单和最轻量的,适合初学者和小型项目。PyQt 是功能最强大的,适合复杂和大型项目。Kivy 是最适合跨平台和多点触控应用程序的。通过本文的介绍,希望您能对如何使用这些库创建弹窗有一个清晰的理解,并能够根据自己的需求选择合适的库进行开发。
相关问答FAQs:
如何在Python中创建弹窗?
在Python中,可以使用多种库来创建弹窗,比如Tkinter、PyQt或wxPython。Tkinter是Python的标准GUI库,使用起来相对简单。以下是一个使用Tkinter创建简单弹窗的示例代码:
import tkinter as tk
from tkinter import messagebox
def show_popup():
messagebox.showinfo("提示", "这是一个弹窗示例")
root = tk.Tk()
root.withdraw() # 隐藏主窗口
show_popup() # 显示弹窗
root.mainloop()
可以自定义弹窗的内容和样式吗?
是的,使用Tkinter或其他GUI库,您可以自定义弹窗的标题、内容、按钮样式等。Tkinter允许您通过不同的消息框类型(如showinfo
, showwarning
, showerror
等)来改变弹窗的外观和功能。此外,您还可以使用自定义窗口来设计更复杂的弹窗界面。
使用弹窗时需要注意哪些事项?
在使用弹窗时,需考虑用户体验,避免频繁出现的弹窗打扰用户。应确保弹窗的信息简洁明了,并提供明确的操作选项。同时,考虑到不同操作系统的界面风格,适当调整弹窗的外观,以保持一致性和美观性。确保在应用程序中合理使用弹窗,以增强功能性而非增加干扰。
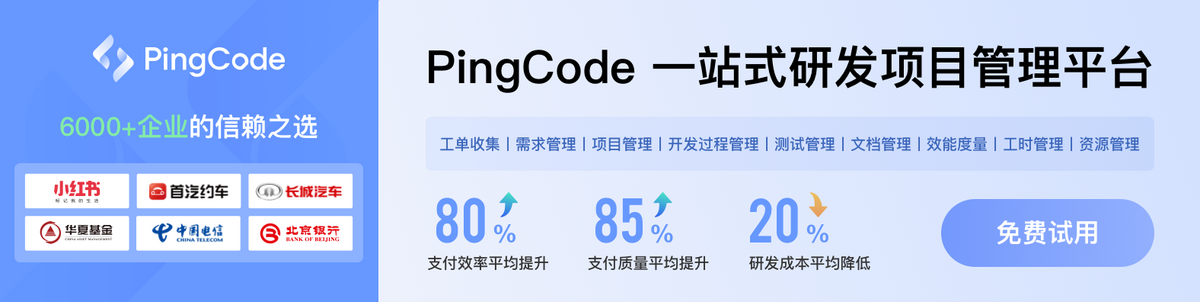