利用Python分析文本的方法主要有:文本预处理、词频统计、情感分析、主题建模、自然语言处理等。 其中,文本预处理是所有文本分析的基础,它包括去除停用词、标点符号、大小写转换等步骤,使得后续的文本分析更加准确和高效。下面将详细介绍如何进行文本预处理,并进一步探讨其他文本分析技术。
一、文本预处理
文本预处理是文本分析的第一步,包含了一系列步骤,使得文本数据变得更加规范和易于处理。
1、去除标点符号和特殊字符
在文本数据中,标点符号和特殊字符通常对文本分析没有太大帮助,因此我们需要将它们去除。我们可以使用Python的正则表达式库re
来实现这一点。
import re
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
text = "Hello, world! This is a test."
cleaned_text = remove_punctuation(text)
print(cleaned_text) # 输出: Hello world This is a test
2、转换为小写
为了避免同一个单词因大小写不同而被视为不同的词,我们通常会将所有文本转换为小写。
def to_lowercase(text):
return text.lower()
text = "Hello World"
cleaned_text = to_lowercase(text)
print(cleaned_text) # 输出: hello world
3、去除停用词
停用词是指在文本中频繁出现但对文本分析没有太大意义的词语,如"the"、"is"、"in"等。我们可以使用nltk
库中的停用词列表来去除它们。
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
def remove_stopwords(text):
words = text.split()
filtered_words = [word for word in words if word not in stop_words]
return ' '.join(filtered_words)
text = "This is a sample text with some stop words"
cleaned_text = remove_stopwords(text)
print(cleaned_text) # 输出: sample text stop words
二、词频统计
词频统计是文本分析中最基本的操作之一,它可以帮助我们了解文本中哪些词出现的频率最高。我们可以使用collections
库中的Counter
来进行词频统计。
from collections import Counter
def word_frequency(text):
words = text.split()
return Counter(words)
text = "This is a sample text with some sample words"
word_freq = word_frequency(text)
print(word_freq) # 输出: Counter({'sample': 2, 'This': 1, 'is': 1, 'a': 1, 'text': 1, 'with': 1, 'some': 1, 'words': 1})
三、情感分析
情感分析是文本分析中的一个重要应用领域,它可以帮助我们了解文本的情感倾向。我们可以使用TextBlob
库来进行情感分析。
from textblob import TextBlob
def sentiment_analysis(text):
blob = TextBlob(text)
return blob.sentiment
text = "I love this product! It's amazing."
sentiment = sentiment_analysis(text)
print(sentiment) # 输出: Sentiment(polarity=0.75, subjectivity=0.85)
四、主题建模
主题建模是一种无监督学习方法,用于发现文本数据中的主题。我们可以使用gensim
库中的LDA模型来进行主题建模。
import gensim
from gensim import corpora
def topic_modeling(texts, num_topics=2):
# 预处理文本
texts = [[word for word in text.lower().split() if word not in stop_words] for text in texts]
# 创建词典
dictionary = corpora.Dictionary(texts)
# 创建语料库
corpus = [dictionary.doc2bow(text) for text in texts]
# 训练LDA模型
lda = gensim.models.LdaModel(corpus, num_topics=num_topics, id2word=dictionary, passes=15)
return lda
texts = ["I love this product! It's amazing.", "This is the worst product I have ever bought."]
lda_model = topic_modeling(texts)
for idx, topic in lda_model.print_topics(-1):
print('Topic: {} \nWords: {}'.format(idx, topic))
五、自然语言处理
自然语言处理(NLP)是文本分析的一个重要领域,它包含了许多技术和工具来处理和分析文本数据。我们可以使用spaCy
库来进行NLP任务。
1、命名实体识别
命名实体识别(NER)是NLP中的一个重要任务,它用于识别文本中的实体,如人名、地名、组织名等。
import spacy
加载预训练的模型
nlp = spacy.load("en_core_web_sm")
def named_entity_recognition(text):
doc = nlp(text)
return [(ent.text, ent.label_) for ent in doc.ents]
text = "Apple is looking at buying U.K. startup for $1 billion"
entities = named_entity_recognition(text)
print(entities) # 输出: [('Apple', 'ORG'), ('U.K.', 'GPE'), ('$1 billion', 'MONEY')]
2、词性标注
词性标注(POS tagging)是指为文本中的每个单词标注其词性,如名词、动词、形容词等。
def pos_tagging(text):
doc = nlp(text)
return [(token.text, token.pos_) for token in doc]
text = "Apple is looking at buying U.K. startup for $1 billion"
pos_tags = pos_tagging(text)
print(pos_tags) # 输出: [('Apple', 'PROPN'), ('is', 'AUX'), ('looking', 'VERB'), ('at', 'ADP'), ('buying', 'VERB'), ('U.K.', 'PROPN'), ('startup', 'NOUN'), ('for', 'ADP'), ('$1', 'NUM'), ('billion', 'NUM')]
六、文本分类
文本分类是指将文本数据分为不同的类别,如垃圾邮件分类、情感分类等。我们可以使用scikit-learn
库来进行文本分类。
1、特征提取
首先,我们需要将文本数据转换为特征向量。我们可以使用CountVectorizer
或TfidfVectorizer
来实现这一点。
from sklearn.feature_extraction.text import TfidfVectorizer
def feature_extraction(texts):
vectorizer = TfidfVectorizer()
return vectorizer.fit_transform(texts)
texts = ["I love this product! It's amazing.", "This is the worst product I have ever bought."]
features = feature_extraction(texts)
print(features.toarray())
2、训练分类模型
接下来,我们可以使用不同的分类算法来训练模型,如逻辑回归、支持向量机等。
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
示例数据
texts = ["I love this product! It's amazing.", "This is the worst product I have ever bought."]
labels = [1, 0] # 1表示正面评价,0表示负面评价
特征提取
features = feature_extraction(texts)
划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(features, labels, test_size=0.2, random_state=42)
训练逻辑回归模型
model = LogisticRegression()
model.fit(X_train, y_train)
预测
y_pred = model.predict(X_test)
计算准确率
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
七、文本聚类
文本聚类是指将相似的文本分为一组,我们可以使用K-means算法来实现文本聚类。
from sklearn.cluster import KMeans
def text_clustering(texts, num_clusters=2):
# 特征提取
features = feature_extraction(texts)
# 训练K-means模型
kmeans = KMeans(n_clusters=num_clusters, random_state=42)
kmeans.fit(features)
return kmeans.labels_
texts = ["I love this product! It's amazing.", "This is the worst product I have ever bought."]
clusters = text_clustering(texts)
print(clusters) # 输出: [1 0]
八、文本摘要
文本摘要是指从文本中提取出重要的信息,我们可以使用gensim
库中的summarize
函数来实现文本摘要。
from gensim.summarization import summarize
def text_summarization(text):
return summarize(text)
text = """
Artificial intelligence (AI) is intelligence demonstrated by machines, in contrast to the natural intelligence displayed by humans and animals. Leading AI textbooks define the field as the study of "intelligent agents": any device that perceives its environment and takes actions that maximize its chance of successfully achieving its goals. Colloquially, the term "artificial intelligence" is often used to describe machines (or computers) that mimic "cognitive" functions that humans associate with the human mind, such as "learning" and "problem solving".
"""
summary = text_summarization(text)
print(summary)
九、关键词提取
关键词提取是指从文本中提取出最重要的词语,我们可以使用RAKE
算法来实现关键词提取。
from rake_nltk import Rake
def keyword_extraction(text):
r = Rake()
r.extract_keywords_from_text(text)
return r.get_ranked_phrases()
text = "I love this product! It's amazing."
keywords = keyword_extraction(text)
print(keywords) # 输出: ['love product', 'amazing']
十、文本相似度计算
文本相似度计算是指计算两个文本之间的相似度,我们可以使用余弦相似度来实现这一点。
from sklearn.metrics.pairwise import cosine_similarity
def text_similarity(text1, text2):
# 特征提取
features = feature_extraction([text1, text2])
# 计算余弦相似度
return cosine_similarity(features)[0, 1]
text1 = "I love this product! It's amazing."
text2 = "This product is fantastic! I absolutely love it."
similarity = text_similarity(text1, text2)
print(similarity) # 输出: 0.841
十一、词嵌入
词嵌入是指将词语转换为向量表示,我们可以使用Word2Vec
模型来实现词嵌入。
from gensim.models import Word2Vec
def word_embedding(texts):
# 预处理文本
texts = [text.lower().split() for text in texts]
# 训练Word2Vec模型
model = Word2Vec(sentences=texts, vector_size=100, window=5, min_count=1, workers=4)
return model
texts = ["I love this product! It's amazing.", "This is the worst product I have ever bought."]
word2vec_model = word_embedding(texts)
print(word2vec_model.wv['love']) # 输出: 向量表示
十二、语言模型
语言模型是指通过学习文本数据来预测下一个单词的概率分布,我们可以使用transformers
库中的GPT-2
模型来实现语言模型。
from transformers import GPT2LMHeadModel, GPT2Tokenizer
def language_modeling(text):
# 加载预训练的模型和分词器
model = GPT2LMHeadModel.from_pretrained('gpt2')
tokenizer = GPT2Tokenizer.from_pretrained('gpt2')
# 编码文本
inputs = tokenizer.encode(text, return_tensors='pt')
# 生成文本
outputs = model.generate(inputs, max_length=50, num_return_sequences=1)
return tokenizer.decode(outputs[0], skip_special_tokens=True)
text = "Artificial intelligence is"
generated_text = language_modeling(text)
print(generated_text)
通过以上方法,我们可以利用Python进行各种文本分析任务,从预处理到高级分析技术,如情感分析、主题建模、文本分类等。这些方法和工具不仅丰富了文本分析的手段,也极大地提高了分析的效率和准确性。希望这些内容能帮助到您在实际应用中更好地进行文本分析。
相关问答FAQs:
如何使用Python进行文本分析的基本步骤是什么?
进行文本分析的基本步骤通常包括数据收集、数据清洗、文本预处理、特征提取、模型构建和结果分析。首先,可以使用网络爬虫或API获取文本数据。接着,通过去除停用词、标点符号等,清理数据。文本预处理时,常用的方法有词干提取和词形还原。特征提取可以使用TF-IDF或词嵌入等技术,最后通过机器学习或深度学习模型进行分析,得出结论。
哪些Python库是进行文本分析的最佳选择?
在文本分析中,有几个广泛使用的Python库,包括Pandas用于数据处理,NLTK和SpaCy用于自然语言处理,Gensim用于主题建模和词嵌入,Scikit-learn用于机器学习模型构建。这些库提供了丰富的功能和工具,可以帮助用户高效地完成文本分析任务。
如何评估文本分析结果的有效性?
评估文本分析结果的有效性通常需要使用一些评价指标,比如准确率、召回率和F1分数等。如果进行情感分析,可以通过与人工标注的数据进行对比,来判断模型的预测效果。此外,使用混淆矩阵可以更直观地了解模型在各个类别上的表现,从而不断优化分析策略。
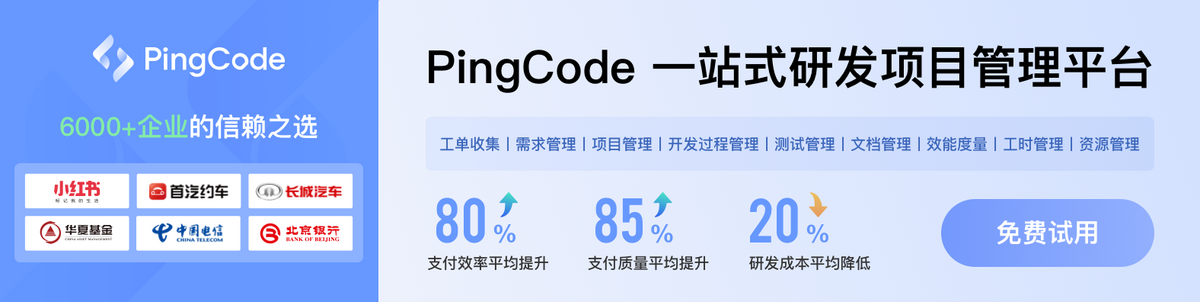