Python 文件复制文件可以通过使用shutil
模块、手动读取和写入文件、以及使用os
模块来实现。 其中,shutil
模块最常用、操作最简单、功能全面,它不仅可以复制文件,还可以复制整个目录以及文件的元数据等。下面我们就来详细介绍这些方法。
一、使用 shutil
模块
shutil
模块是 Python 标准库中的一个模块,提供了许多高级的文件操作,包括复制文件和目录。
1.1 shutil.copyfile()
复制文件内容
shutil.copyfile()
函数用于复制文件内容,不包括文件权限等元数据。
import shutil
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file.txt'
shutil.copyfile(source_file, destination_file)
1.2 shutil.copy()
复制文件和权限
shutil.copy()
函数不仅复制文件内容,还复制文件的权限。
import shutil
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file.txt'
shutil.copy(source_file, destination_file)
1.3 shutil.copy2()
复制文件和所有元数据
shutil.copy2()
函数复制文件内容、权限、最后访问时间和修改时间等所有元数据。
import shutil
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file.txt'
shutil.copy2(source_file, destination_file)
二、手动读取和写入文件
如果你希望对文件内容进行一些处理后再复制,可以手动读取和写入文件。
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file.txt'
with open(source_file, 'rb') as f_src:
with open(destination_file, 'wb') as f_dst:
f_dst.write(f_src.read())
三、使用 os
模块
os
模块也可以用于文件复制操作,尽管它不如 shutil
模块那么方便。os
模块主要用于低级别的文件操作。
3.1 使用 os.open
和 os.write
import os
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file.txt'
with open(source_file, 'rb') as f_src:
with open(destination_file, 'wb') as f_dst:
while True:
chunk = f_src.read(1024)
if not chunk:
break
f_dst.write(chunk)
3.2 使用 os.system
调用系统命令
import os
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file.txt'
os.system(f'cp {source_file} {destination_file}')
四、比较三种方法
4.1 shutil
模块的优点
- 操作简单:只需调用一个函数即可完成复制操作。
- 功能全面:提供了多种复制方法,可以满足不同需求。
- 可靠性高:
shutil
是 Python 标准库的一部分,经过广泛测试和使用,可靠性高。
4.2 手动读取和写入的优点
- 灵活性高:可以对文件内容进行任意处理后再写入目标文件。
- 细粒度控制:可以逐块读取和写入文件,适用于大文件处理。
4.3 os
模块的优点
- 低级别控制:可以直接调用操作系统命令,适用于特殊需求。
- 跨平台性差:不同操作系统的命令不同,脚本的跨平台性差。
五、实际应用场景
5.1 备份文件
在实际应用中,文件复制操作经常用于文件备份。可以通过 shutil.copy2()
函数将文件备份到指定目录,并保留文件的所有元数据。
import shutil
import os
source_file = 'path/to/source/file.txt'
backup_dir = 'path/to/backup/dir'
if not os.path.exists(backup_dir):
os.makedirs(backup_dir)
shutil.copy2(source_file, os.path.join(backup_dir, os.path.basename(source_file)))
5.2 批量复制文件
有时需要批量复制多个文件,可以使用 os.listdir()
获取目录中的所有文件,然后逐个复制。
import shutil
import os
source_dir = 'path/to/source/dir'
destination_dir = 'path/to/destination/dir'
if not os.path.exists(destination_dir):
os.makedirs(destination_dir)
for filename in os.listdir(source_dir):
source_file = os.path.join(source_dir, filename)
destination_file = os.path.join(destination_dir, filename)
if os.path.isfile(source_file):
shutil.copy2(source_file, destination_file)
5.3 监控文件变化并复制
可以使用 watchdog
库监控目录中的文件变化,一旦有文件新增或修改,就自动复制到目标目录。
import shutil
import os
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class FileCopyHandler(FileSystemEventHandler):
def __init__(self, source_dir, destination_dir):
self.source_dir = source_dir
self.destination_dir = destination_dir
def on_modified(self, event):
if event.is_directory:
return
source_file = event.src_path
destination_file = os.path.join(self.destination_dir, os.path.basename(source_file))
shutil.copy2(source_file, destination_file)
def on_created(self, event):
self.on_modified(event)
source_dir = 'path/to/source/dir'
destination_dir = 'path/to/destination/dir'
if not os.path.exists(destination_dir):
os.makedirs(destination_dir)
event_handler = FileCopyHandler(source_dir, destination_dir)
observer = Observer()
observer.schedule(event_handler, source_dir, recursive=True)
observer.start()
try:
while True:
pass
except KeyboardInterrupt:
observer.stop()
observer.join()
六、总结
通过以上内容,我们详细介绍了 Python 中实现文件复制的几种方法,并对每种方法进行了比较,shutil
模块是最常用、最方便的选择,尤其是对于简单的文件复制操作。手动读取和写入文件则适用于需要对文件内容进行处理的场景,而 os
模块虽然不常用,但在某些特殊需求下仍然可以发挥作用。
实际应用中,选择合适的方法可以提高代码的可读性和维护性,同时满足具体需求。掌握这些方法后,你可以根据不同的需求灵活选择和组合使用,从而高效地完成文件复制任务。
相关问答FAQs:
如何在Python中实现文件复制的操作?
在Python中,可以使用内置的shutil
模块进行文件复制。具体方法是导入shutil
,然后使用shutil.copy()
函数,该函数可以复制文件到目标路径。示例代码如下:
import shutil
shutil.copy('source_file.txt', 'destination_file.txt')
这段代码将source_file.txt
复制到destination_file.txt
,如果目标文件已存在,则会被覆盖。
文件复制时可以保留元数据吗?
是的,使用shutil.copy2()
函数可以在复制文件时保留文件的元数据,包括创建时间和修改时间等。下面是一个简单的例子:
import shutil
shutil.copy2('source_file.txt', 'destination_file.txt')
这样复制后,目标文件会保留源文件的所有元数据。
遇到文件复制失败时该如何处理?
在进行文件复制时,可能会遇到文件不存在、权限不足等情况,可以通过异常处理来应对这些问题。使用try
和except
语句可以捕获并处理这些异常。例如:
import shutil
try:
shutil.copy('source_file.txt', 'destination_file.txt')
except FileNotFoundError:
print("源文件不存在,请检查路径。")
except PermissionError:
print("没有权限复制文件,请检查权限设置。")
通过这样的方式,能够有效提升代码的健壮性并提供用户友好的错误提示。
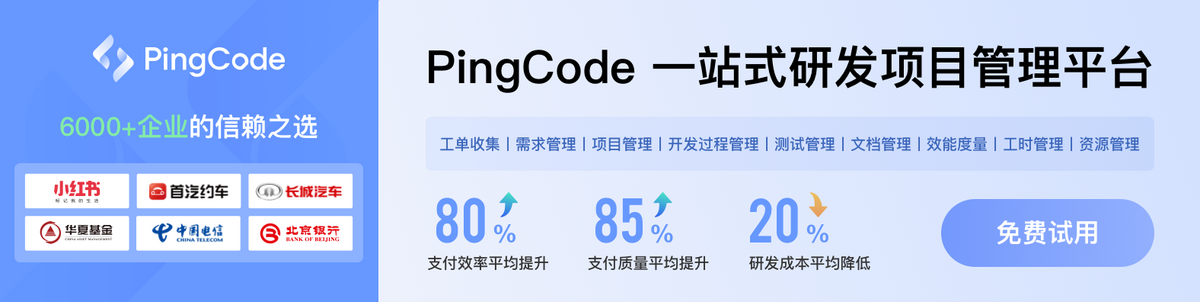