切换到目录的主要方法包括使用os模块、使用pathlib模块、使用subprocess模块。其中,使用os模块是最常见的方法。os模块提供了操作系统的接口,允许你与操作系统进行交互。通过os.chdir()函数,可以轻松地切换到指定的目录。
例如,要切换到/home/user/Documents
目录,使用以下代码:
import os
os.chdir('/home/user/Documents')
这行代码将当前工作目录更改为/home/user/Documents
,之后的文件操作都会在这个目录下进行。
接下来,我们将详细介绍这几种方法的实现和适用场景。
一、使用os模块
1.1 更改当前工作目录
os模块是Python标准库的一部分,提供了与操作系统进行交互的功能。通过os.chdir()
函数,可以更改当前工作目录。
import os
os.chdir('/desired/path')
这行代码将当前工作目录更改为/desired/path
。更改后,任何相对路径的文件操作都将在该目录下进行。
1.2 获取当前工作目录
可以使用os.getcwd()
函数获取当前工作目录:
current_directory = os.getcwd()
print(f'Current directory: {current_directory}')
这将打印当前工作目录的路径。
1.3 检查目录是否存在
在更改目录之前,通常需要检查目录是否存在。可以使用os.path.exists()
函数来执行此操作:
import os
if os.path.exists('/desired/path'):
os.chdir('/desired/path')
else:
print('Directory does not exist')
这种方法可以避免因目录不存在而引发的错误。
二、使用pathlib模块
2.1 更改当前工作目录
pathlib模块是Python 3.4引入的,用于处理文件系统路径的模块。它提供了更面向对象的接口。使用Path.cwd()
和Path.chdir()
函数,可以更改和获取当前工作目录:
from pathlib import Path
p = Path('/desired/path')
if p.exists():
p.chdir()
else:
print('Directory does not exist')
2.2 获取当前工作目录
可以使用Path.cwd()
函数获取当前工作目录:
from pathlib import Path
current_directory = Path.cwd()
print(f'Current directory: {current_directory}')
三、使用subprocess模块
3.1 使用子进程更改目录
subprocess模块允许你生成新的进程,连接到它们的输入/输出/错误管道,并获取它们的返回值。虽然它不直接提供更改当前工作目录的功能,但可以通过调用外部命令来实现:
import subprocess
subprocess.run(['cd', '/desired/path'], shell=True)
这种方法不常用,因为它启动了一个子进程,更改的目录对主进程没有影响。
四、错误处理和异常处理
在更改目录时,可能会遇到各种错误。例如,目录不存在、权限不足等。可以使用try-except块来处理这些错误:
import os
try:
os.chdir('/desired/path')
except FileNotFoundError:
print('Directory does not exist')
except PermissionError:
print('Permission denied')
except Exception as e:
print(f'An error occurred: {e}')
这种方法提高了代码的鲁棒性,使其在面对异常情况时更加健壮。
五、结合使用多种方法
在实际开发中,可能需要结合使用多种方法。例如,在更改目录之前,使用pathlib模块检查目录是否存在,然后使用os模块更改目录:
from pathlib import Path
import os
p = Path('/desired/path')
if p.exists():
try:
os.chdir(p)
except PermissionError:
print('Permission denied')
else:
print('Directory does not exist')
这种方法结合了两个模块的优点,使代码更简洁、可读性更高。
六、实践中的应用
6.1 自动备份脚本
假设我们需要编写一个自动备份脚本,将某个目录中的文件备份到另一个目录。可以使用os模块更改工作目录,使用shutil模块复制文件:
import os
import shutil
source_dir = '/path/to/source'
backup_dir = '/path/to/backup'
try:
os.chdir(source_dir)
for file_name in os.listdir():
full_file_name = os.path.join(source_dir, file_name)
if os.path.isfile(full_file_name):
shutil.copy(full_file_name, backup_dir)
print('Backup completed successfully')
except Exception as e:
print(f'An error occurred: {e}')
6.2 日志文件管理
在日志文件管理中,可能需要定期切换到特定目录,创建新的日志文件。可以使用pathlib模块创建日志目录,并使用os模块更改工作目录:
from pathlib import Path
import os
log_dir = Path('/path/to/logs')
log_dir.mkdir(parents=True, exist_ok=True)
try:
os.chdir(log_dir)
with open('logfile.log', 'a') as log_file:
log_file.write('New log entry\n')
print('Log entry added successfully')
except Exception as e:
print(f'An error occurred: {e}')
七、性能和优化
在某些情况下,频繁更改工作目录可能会对性能产生影响。可以通过减少不必要的目录切换,优化文件操作的效率。例如:
import os
def process_files_in_directory(directory):
original_directory = os.getcwd()
try:
os.chdir(directory)
for file_name in os.listdir():
full_file_name = os.path.join(directory, file_name)
if os.path.isfile(full_file_name):
# 处理文件
pass
finally:
os.chdir(original_directory)
process_files_in_directory('/path/to/directory')
这种方法减少了不必要的目录切换,提高了代码的执行效率。
八、总结
切换目录是Python编程中一个常见的任务,可以使用os、pathlib和subprocess模块来实现。每种方法都有其优缺点和适用场景。os模块是最常用的方法,提供了简单直接的接口。pathlib模块提供了更面向对象的接口,适合处理复杂路径操作。subprocess模块适用于调用外部命令。在实际开发中,可以结合使用多种方法,根据具体需求选择合适的工具。同时,注意处理异常情况,提高代码的鲁棒性。
通过上述方法和实践中的应用示例,希望能够帮助你更好地理解和掌握Python中切换目录的技巧。
相关问答FAQs:
如何在Python中更改当前工作目录?
在Python中,可以使用os
模块来更改当前工作目录。通过调用os.chdir(path)
,您可以将工作目录更改为指定的路径。确保所提供的路径是有效的,并且您具有访问权限。可以使用os.getcwd()
来验证当前的工作目录。
可以在Python中使用相对路径切换目录吗?
是的,Python允许使用相对路径来切换目录。例如,如果当前工作目录是/home/user
,您可以使用os.chdir('documents')
将目录切换到/home/user/documents
。相对路径是相对于当前工作目录的,因此在使用时请确保路径的正确性。
如何查看当前的工作目录?
要查看当前的工作目录,可以使用os.getcwd()
函数。这个函数会返回一个字符串,表示当前的工作目录路径。这在调试时非常有用,能够帮助您确认Python程序的工作环境。
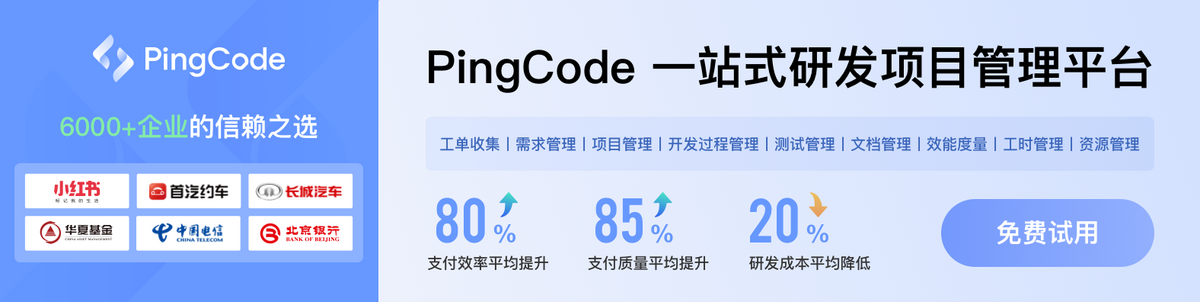