在Python中统计页码的方法有多种,包括使用PDF处理库、OCR技术、以及文本解析等。常用的库有PyPDF2、pdfplumber、和Tesseract OCR。下面将详细描述如何使用这些库中的PyPDF2来统计页码。
PyPDF2 是一个纯Python库,用于处理PDF文件,它提供了读取、拆分、合并、裁剪、加密和解密PDF文件的功能。使用PyPDF2可以轻松地统计PDF文件的页码。首先,我们需要安装PyPDF2库,可以使用以下命令进行安装:
pip install PyPDF2
然后,我们可以通过简单的Python脚本来统计PDF文件的页码。以下是一个示例代码:
import PyPDF2
def count_pages(pdf_path):
with open(pdf_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
num_pages = reader.numPages
return num_pages
pdf_path = 'path/to/your/pdf/file.pdf'
print(f'The number of pages in the PDF is: {count_pages(pdf_path)}')
这段代码实现了一个简单的函数 count_pages
,它接收PDF文件的路径作为参数,然后使用PyPDF2库读取文件并统计页码。下面我们详细讲解这个过程。
一、安装和导入库
首先,我们需要安装并导入PyPDF2库。PyPDF2是一个功能强大的PDF处理库,支持多种PDF操作,包括读取、拆分、合并等。可以使用以下命令安装PyPDF2:
pip install PyPDF2
安装完成后,在Python脚本中导入PyPDF2库:
import PyPDF2
二、打开和读取PDF文件
为了读取PDF文件,我们需要使用Python内置的 open
函数以二进制模式 ('rb'
) 打开文件。这样可以确保文件内容被正确读取,不会因为文本模式读取导致的数据损坏。以下是打开PDF文件的示例代码:
with open('path/to/your/pdf/file.pdf', 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
在 with
语句块中,文件会在代码块结束时自动关闭,这是一个良好的实践,可以避免文件资源泄露。
三、统计页码
PyPDF2库提供了一个名为 PdfFileReader
的类,我们可以使用它读取PDF文件并获取文件的信息。通过 PdfFileReader
实例的 numPages
属性,我们可以轻松获得PDF文件的页码数:
num_pages = reader.numPages
四、完整的示例代码
将上述步骤整合在一起,我们可以得到一个完整的示例代码,用于统计PDF文件的页码:
import PyPDF2
def count_pages(pdf_path):
with open(pdf_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
num_pages = reader.numPages
return num_pages
pdf_path = 'path/to/your/pdf/file.pdf'
print(f'The number of pages in the PDF is: {count_pages(pdf_path)}')
五、处理异常情况
在实际使用过程中,我们可能会遇到一些异常情况,例如文件不存在、文件格式不正确等。为了提高代码的健壮性,我们可以添加异常处理来捕获和处理这些错误:
import PyPDF2
def count_pages(pdf_path):
try:
with open(pdf_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
if reader.isEncrypted:
reader.decrypt('')
num_pages = reader.numPages
return num_pages
except FileNotFoundError:
print("Error: The file was not found.")
except PyPDF2.utils.PdfReadError:
print("Error: The file could not be read as a PDF.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
pdf_path = 'path/to/your/pdf/file.pdf'
pages = count_pages(pdf_path)
if pages is not None:
print(f'The number of pages in the PDF is: {pages}')
在这个示例中,我们使用了 try-except
语句来捕获并处理不同类型的异常,从而确保代码在遇到错误时不会崩溃,并且可以给出相应的提示信息。
六、读取多个PDF文件
如果我们需要统计多个PDF文件的页码,可以使用一个循环来遍历文件列表,并调用 count_pages
函数处理每个文件。例如:
import PyPDF2
def count_pages(pdf_path):
try:
with open(pdf_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
if reader.isEncrypted:
reader.decrypt('')
num_pages = reader.numPages
return num_pages
except FileNotFoundError:
print(f"Error: The file '{pdf_path}' was not found.")
return None
except PyPDF2.utils.PdfReadError:
print(f"Error: The file '{pdf_path}' could not be read as a PDF.")
return None
except Exception as e:
print(f"An unexpected error occurred with file '{pdf_path}': {e}")
return None
pdf_paths = ['file1.pdf', 'file2.pdf', 'file3.pdf']
for path in pdf_paths:
pages = count_pages(path)
if pages is not None:
print(f'The number of pages in {path} is: {pages}')
在这个示例中,我们定义了一个包含多个PDF文件路径的列表 pdf_paths
,然后使用一个 for
循环遍历这个列表,并调用 count_pages
函数来统计每个文件的页码数。
七、合并和拆分PDF文件
除了统计页码,PyPDF2还可以用于合并和拆分PDF文件。例如,以下代码演示了如何将两个PDF文件合并为一个文件:
import PyPDF2
def merge_pdfs(pdf_paths, output_path):
pdf_writer = PyPDF2.PdfFileWriter()
for path in pdf_paths:
pdf_reader = PyPDF2.PdfFileReader(path)
for page_num in range(pdf_reader.numPages):
page = pdf_reader.getPage(page_num)
pdf_writer.addPage(page)
with open(output_path, 'wb') as output_file:
pdf_writer.write(output_file)
pdf_paths = ['file1.pdf', 'file2.pdf']
output_path = 'merged.pdf'
merge_pdfs(pdf_paths, output_path)
print(f'The PDF files have been merged into {output_path}')
在这个示例中,我们定义了一个 merge_pdfs
函数,它接收一个PDF文件路径的列表和一个输出文件路径。我们使用 PdfFileWriter
类来创建一个新的PDF文件,并将每个输入文件的页面添加到新的PDF文件中。最后,将合并后的PDF文件写入输出路径。
八、提取PDF文件中的文本
PyPDF2也可以用于提取PDF文件中的文本内容。以下代码演示了如何提取PDF文件的文本:
import PyPDF2
def extract_text(pdf_path):
text = ""
try:
with open(pdf_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
if reader.isEncrypted:
reader.decrypt('')
for page_num in range(reader.numPages):
page = reader.getPage(page_num)
text += page.extract_text()
except Exception as e:
print(f"An error occurred: {e}")
return text
pdf_path = 'path/to/your/pdf/file.pdf'
pdf_text = extract_text(pdf_path)
print(f'The extracted text from the PDF is:\n{pdf_text}')
在这个示例中,我们定义了一个 extract_text
函数,用于提取PDF文件的文本内容。我们使用 PdfFileReader
类读取PDF文件,并遍历每个页面,调用 extract_text
方法提取页面中的文本。
九、总结
通过以上介绍,我们详细讲解了如何使用PyPDF2库在Python中统计页码、合并和拆分PDF文件、以及提取PDF文件中的文本。PyPDF2是一个功能强大的PDF处理库,提供了丰富的功能,能够满足大多数PDF处理需求。
在实际使用过程中,我们还可以结合其他库和工具,如pdfplumber、Tesseract OCR等,进一步扩展PDF处理的能力。例如,pdfplumber可以更精细地控制页面布局和提取内容,而Tesseract OCR可以用于处理扫描的PDF文件。
通过灵活运用这些工具和技术,我们可以高效地处理和分析PDF文件,提升工作效率和数据处理能力。希望本文能对你在Python中处理PDF文件提供有价值的参考和帮助。
相关问答FAQs:
如何在Python中获取文档的总页码数量?
在Python中,获取文档的总页码通常依赖于使用特定的库,比如PyPDF2或pdfplumber。这些库可以读取PDF文件并提取页码信息。使用PyPDF2,您可以通过以下代码获取页码数量:
import PyPDF2
with open('your_document.pdf', 'rb') as file:
reader = PyPDF2.PdfReader(file)
total_pages = len(reader.pages)
print(f"总页码数量: {total_pages}")
在Python中如何计算文本文件的页码?
对于文本文件,页码的计算通常依赖于每页的内容限制。您可以定义每页的行数或字符数,然后根据文件的总行数或字符数进行计算。例如:
def calculate_pages(file_path, lines_per_page):
with open(file_path, 'r') as file:
lines = file.readlines()
total_pages = len(lines) // lines_per_page + (1 if len(lines) % lines_per_page > 0 else 0)
return total_pages
pages = calculate_pages('your_text_file.txt', 50)
print(f"总页码数量: {pages}")
如何在Python中动态生成带页码的文档?
如果希望在生成的文档中添加页码,可以使用报告实验室(ReportLab)库。此库允许您创建PDF文件并添加页码。以下是一个简单的示例:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
def create_pdf_with_page_numbers(filename, total_pages):
c = canvas.Canvas(filename, pagesize=letter)
width, height = letter
for page in range(total_pages):
c.drawString(100, height - 50, f'Page {page + 1}')
c.showPage() # 生成新的一页
c.save()
create_pdf_with_page_numbers('output.pdf', 5)
这种方法可以有效地在每一页上添加页码,满足您文档生成的需求。
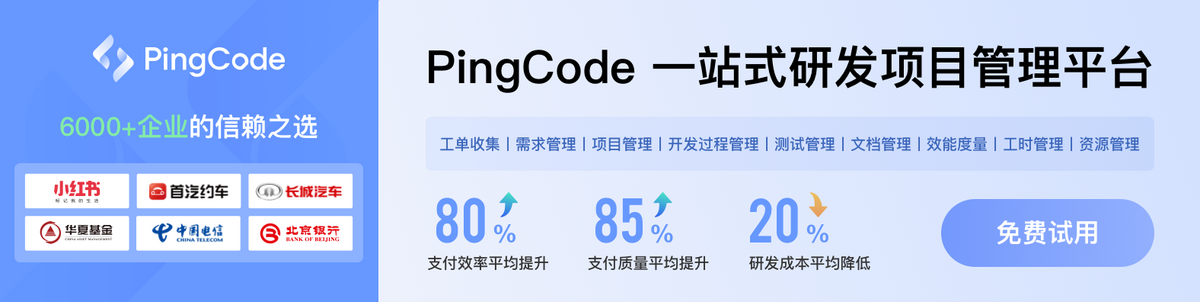