在Python中删除项目的方法有多种,可以使用os
模块、shutil
模块、以及使用Pip工具管理Python包。本文将详细介绍这些方法,并结合实例展示实际操作。
一、使用os模块
os
模块是Python标准库中用于与操作系统进行交互的模块,os
模块中的os.remove()
和os.rmdir()
可以用于删除文件和目录。
1. 删除文件
使用os.remove()
可以删除指定的文件。如果文件不存在,将会引发FileNotFoundError
异常。
import os
file_path = 'path/to/your/file.txt'
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
2. 删除空目录
使用os.rmdir()
可以删除空目录。如果目录不为空,将会引发OSError
异常。
import os
dir_path = 'path/to/your/empty_directory'
try:
os.rmdir(dir_path)
print(f"{dir_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{dir_path} does not exist.")
except OSError:
print(f"{dir_path} is not empty or permission denied.")
二、使用shutil模块
shutil
模块提供了一些高级的文件操作功能,包括复制、移动、删除文件和目录。特别是shutil.rmtree()
函数,可以递归删除目录及其下的所有内容。
1. 递归删除目录
使用shutil.rmtree()
可以递归删除目录及其下的所有文件和子目录。
import shutil
dir_path = 'path/to/your/directory'
try:
shutil.rmtree(dir_path)
print(f"{dir_path} and all its contents have been deleted successfully.")
except FileNotFoundError:
print(f"{dir_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {dir_path}.")
三、使用Pip工具管理Python包
使用Pip工具来管理Python包是最常用的方法之一。Pip工具可以安装、升级和卸载Python包。通过命令行可以使用pip uninstall
命令来卸载已安装的Python包。
1. 卸载Python包
使用pip uninstall
命令可以卸载指定的Python包。
pip uninstall package_name
可以在Python脚本中使用subprocess
模块调用pip uninstall
命令来卸载Python包。
import subprocess
package_name = 'your_package_name'
try:
subprocess.check_call(['pip', 'uninstall', '-y', package_name])
print(f"{package_name} has been uninstalled successfully.")
except subprocess.CalledProcessError:
print(f"Failed to uninstall {package_name}.")
四、删除项目中的特定内容
在项目中删除特定内容可以使用多种方法,根据具体需求选择合适的方法。例如,删除项目中的某些类型的文件,或者根据文件名模式删除文件。
1. 删除特定类型的文件
使用os
模块结合glob
模块可以删除特定类型的文件。
import os
import glob
file_pattern = 'path/to/your/project/*.log'
for file_path in glob.glob(file_pattern):
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
2. 删除特定模式的文件
可以使用正则表达式匹配文件名,并删除符合模式的文件。
import os
import re
dir_path = 'path/to/your/project'
pattern = re.compile(r'file_pattern.*\.log')
for root, dirs, files in os.walk(dir_path):
for file in files:
if pattern.match(file):
file_path = os.path.join(root, file)
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
五、删除项目中的敏感信息
在项目中删除敏感信息是一个非常重要的任务,可以通过遍历文件内容并使用正则表达式匹配来删除敏感信息。
1. 替换敏感信息
可以使用正则表达式匹配敏感信息并将其替换为空字符串或其它字符。
import os
import re
dir_path = 'path/to/your/project'
pattern = re.compile(r'sensitive_information_pattern')
for root, dirs, files in os.walk(dir_path):
for file in files:
file_path = os.path.join(root, file)
try:
with open(file_path, 'r') as f:
content = f.read()
new_content = pattern.sub('REPLACEMENT', content)
with open(file_path, 'w') as f:
f.write(new_content)
print(f"Sensistive information in {file_path} has been replaced successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to modify {file_path}.")
六、删除项目中的空文件夹
项目中可能会存在一些空文件夹,可以通过递归遍历目录并删除空文件夹。
1. 递归删除空文件夹
可以编写一个递归函数删除项目中的空文件夹。
import os
def remove_empty_folders(path):
if not os.path.isdir(path):
return
# 递归删除子目录中的空文件夹
for folder in os.listdir(path):
folder_path = os.path.join(path, folder)
remove_empty_folders(folder_path)
# 删除当前目录如果它是空的
if not os.listdir(path):
try:
os.rmdir(path)
print(f"{path} has been deleted successfully.")
except PermissionError:
print(f"Permission denied to delete {path}.")
dir_path = 'path/to/your/project'
remove_empty_folders(dir_path)
七、删除项目中的临时文件
项目中可能会存在一些临时文件,可以通过匹配文件名或文件扩展名来删除这些临时文件。
1. 删除特定扩展名的临时文件
可以使用os
模块结合glob
模块删除特定扩展名的临时文件。
import os
import glob
file_pattern = 'path/to/your/project/*.tmp'
for file_path in glob.glob(file_pattern):
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
八、删除项目中的日志文件
项目中可能会存在大量的日志文件,可以通过匹配文件名或文件扩展名来删除这些日志文件。
1. 删除特定扩展名的日志文件
可以使用os
模块结合glob
模块删除特定扩展名的日志文件。
import os
import glob
file_pattern = 'path/to/your/project/*.log'
for file_path in glob.glob(file_pattern):
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
2. 删除特定时间之前的日志文件
可以根据文件的修改时间删除特定时间之前的日志文件。
import os
import time
dir_path = 'path/to/your/project'
days_threshold = 30
current_time = time.time()
for root, dirs, files in os.walk(dir_path):
for file in files:
if file.endswith('.log'):
file_path = os.path.join(root, file)
file_mtime = os.path.getmtime(file_path)
file_age_days = (current_time - file_mtime) / (24 * 60 * 60)
if file_age_days > days_threshold:
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
九、删除项目中的备份文件
项目中可能会存在一些备份文件,可以通过匹配文件名或文件扩展名来删除这些备份文件。
1. 删除特定扩展名的备份文件
可以使用os
模块结合glob
模块删除特定扩展名的备份文件。
import os
import glob
file_pattern = 'path/to/your/project/*.bak'
for file_path in glob.glob(file_pattern):
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
十、删除项目中的大文件
项目中可能会存在一些大文件,可以根据文件大小删除这些大文件。
1. 删除超过特定大小的文件
可以根据文件的大小删除超过特定大小的文件。
import os
dir_path = 'path/to/your/project'
size_threshold = 100 * 1024 * 1024 # 100 MB
for root, dirs, files in os.walk(dir_path):
for file in files:
file_path = os.path.join(root, file)
file_size = os.path.getsize(file_path)
if file_size > size_threshold:
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} does not exist.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
总结
本文详细介绍了在Python中删除项目的多种方法,包括使用os
模块、shutil
模块、Pip工具管理Python包,以及删除项目中的特定内容、敏感信息、空文件夹、临时文件、日志文件、备份文件和大文件。希望这些方法能帮助您更好地管理和维护您的项目。
通过以上的方法,您可以灵活地删除项目中的各种文件和目录,从而更好地管理您的项目。如果在实际操作中遇到问题,可以参考本文中的代码示例,并根据具体需求进行适当的修改和调整。
相关问答FAQs:
如何在Python中删除文件或目录?
在Python中,可以使用os
模块来删除文件和目录。要删除文件,可以使用os.remove(file_path)
,而要删除空目录,则可以使用os.rmdir(directory_path)
。如果要删除非空目录,需使用shutil
模块的shutil.rmtree(directory_path)
方法。确保在执行删除操作时,文件或目录的路径正确,以免误删重要数据。
使用Python删除项目时,有哪些注意事项?
在删除项目时,必须确认您要删除的文件或目录不再需要。建议在删除之前备份重要数据,以防止意外丢失。同时,检查文件的权限设置,确保您具有删除该文件或目录的权限。此外,使用try
和except
语句捕获可能发生的异常,以增强代码的稳健性。
如何安全地删除Python中的项目文件?
为了安全删除项目文件,可以先通过os.path.exists(file_path)
检查文件是否存在,确保文件在删除前是有效的。还可以实现用户确认机制,例如在删除前提示用户确认是否继续操作。同时,考虑使用版本控制系统(如Git)来管理项目文件,便于恢复误删的文件。
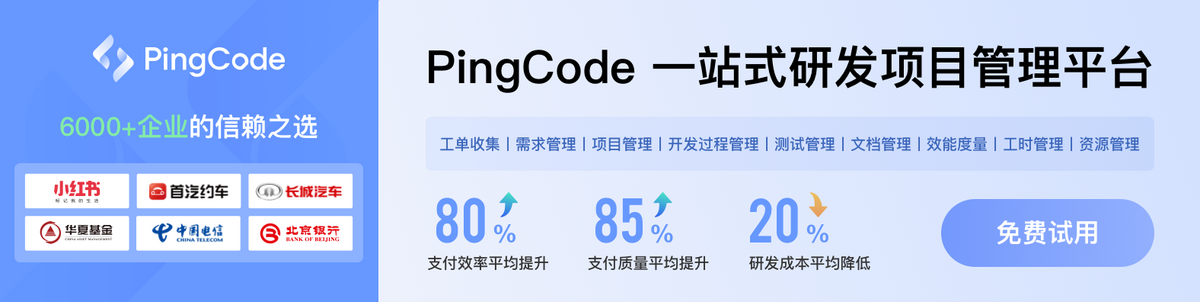