在Python中,找到文件路径的方法有很多,可以使用os模块、pathlib模块、glob模块、以及第三方库like scandir等。其中,最常用的方法是通过os模块和pathlib模块。下面我们将详细介绍这些方法,并给出一些示例代码。
一、使用os模块
os模块是Python标准库中的一部分,用于与操作系统进行交互。它提供了丰富的函数来操作文件和目录。以下是如何使用os模块来查找文件路径的方法。
1. 获取当前工作目录
import os
current_directory = os.getcwd()
print("当前工作目录:", current_directory)
2. 构建文件路径
file_name = "example.txt"
file_path = os.path.join(current_directory, file_name)
print("文件路径:", file_path)
3. 检查文件是否存在
if os.path.exists(file_path):
print(f"{file_name} 存在")
else:
print(f"{file_name} 不存在")
4. 列出目录中的文件
files = os.listdir(current_directory)
print("目录中的文件:", files)
二、使用pathlib模块
pathlib模块是在Python 3.4中引入的,它提供了面向对象的路径操作方法。以下是如何使用pathlib模块来查找文件路径的方法。
1. 获取当前工作目录
from pathlib import Path
current_directory = Path.cwd()
print("当前工作目录:", current_directory)
2. 构建文件路径
file_name = "example.txt"
file_path = current_directory / file_name
print("文件路径:", file_path)
3. 检查文件是否存在
if file_path.exists():
print(f"{file_name} 存在")
else:
print(f"{file_name} 不存在")
4. 列出目录中的文件
files = list(current_directory.iterdir())
print("目录中的文件:", files)
三、使用glob模块
glob模块用于查找符合特定模式的文件名或目录名。以下是如何使用glob模块来查找文件路径的方法。
1. 导入glob模块
import glob
2. 查找特定模式的文件
pattern = "*.txt"
files = glob.glob(pattern)
print("匹配的文件:", files)
3. 查找子目录中的文件
pattern = "/*.txt"
files = glob.glob(pattern, recursive=True)
print("匹配的文件:", files)
四、使用第三方库
除了标准库外,还有一些第三方库可以用来查找文件路径。例如,scandir库提供了更高效的目录扫描功能。
1. 安装scandir库
pip install scandir
2. 使用scandir库查找文件
from scandir import scandir
def list_files(directory):
for entry in scandir(directory):
if entry.is_file():
print("文件:", entry.name)
elif entry.is_dir():
print("目录:", entry.name)
current_directory = os.getcwd()
list_files(current_directory)
五、综合示例
为了更好地理解这些方法,下面我们将综合使用这些模块来实现一个查找文件的示例。
import os
from pathlib import Path
import glob
from scandir import scandir
def find_files(directory, pattern):
# 使用os模块
print("使用os模块:")
for root, dirs, files in os.walk(directory):
for file in files:
if file.endswith(pattern):
print(os.path.join(root, file))
# 使用pathlib模块
print("使用pathlib模块:")
current_directory = Path(directory)
for file_path in current_directory.rglob(pattern):
print(file_path)
# 使用glob模块
print("使用glob模块:")
pattern = os.path.join(directory, "", pattern)
for file_path in glob.glob(pattern, recursive=True):
print(file_path)
# 使用scandir库
print("使用scandir库:")
def scan_directory(directory, pattern):
for entry in scandir(directory):
if entry.is_file() and entry.name.endswith(pattern):
print(entry.path)
elif entry.is_dir():
scan_directory(entry.path, pattern)
scan_directory(directory, pattern)
示例调用
directory = os.getcwd()
pattern = ".txt"
find_files(directory, pattern)
结论
通过以上方法,可以轻松地在Python中找到文件路径。os模块适用于大多数基本操作、pathlib模块提供了更现代的面向对象接口、glob模块适用于模式匹配、scandir库则提供了更高效的目录扫描。根据具体需求选择合适的方法,可以提高代码的可读性和执行效率。通过这些方法,你可以在Python中方便地查找和操作文件路径,从而更好地管理文件和目录。
相关问答FAQs:
如何在Python中获取当前工作目录的路径?
在Python中,可以使用os
模块的getcwd()
函数来获取当前工作目录的路径。示例代码如下:
import os
current_directory = os.getcwd()
print("当前工作目录:", current_directory)
这个方法非常适合在需要知道当前运行脚本位置的情况下使用。
如何查找特定文件的绝对路径?
如果想要找到特定文件的绝对路径,可以使用os.path.abspath()
函数。通过传入文件名或相对路径,可以返回文件的绝对路径。例如:
import os
file_path = 'example.txt'
absolute_path = os.path.abspath(file_path)
print("文件的绝对路径:", absolute_path)
确保文件存在于指定路径下,这样才能获取正确的绝对路径。
在Python中如何遍历目录以查找文件?
可以使用os
模块的walk()
函数来遍历目录并查找特定的文件。例如,下面的代码将遍历指定目录并打印所有文件的路径:
import os
for dirpath, dirnames, filenames in os.walk('your_directory'):
for filename in filenames:
print(os.path.join(dirpath, filename))
这种方法非常适合在大文件夹中搜索特定类型的文件。
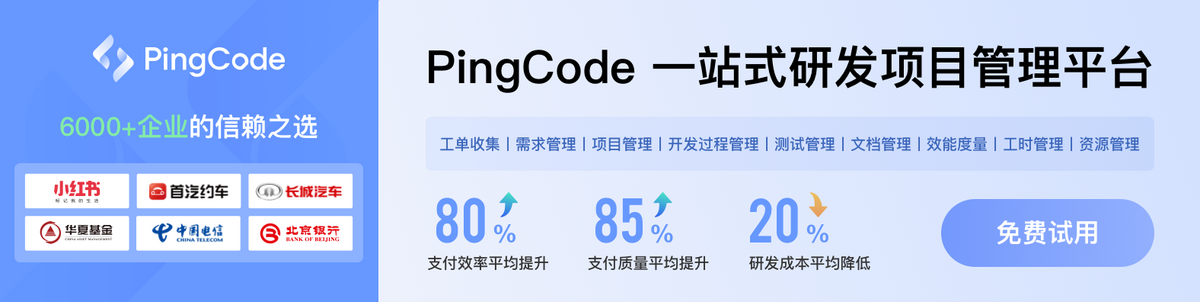