使用Python进行绘图时,可以通过Matplotlib库来创建图例标题、添加图例和自定义图例样式。具体方法包括:使用plt.legend()函数、设置loc参数、使用title参数、调整bbox_to_anchor参数。
其中,使用plt.legend()函数是最常用的方法。plt.legend()函数可以在图表中添加图例,并通过参数设置图例的标题、位置、样式等。下面将详细描述如何使用plt.legend()函数来设置图例标题。
一、添加图例
在使用Matplotlib绘制图表时,添加图例是非常重要的一步。图例可以帮助观众更好地理解图表中的数据。我们可以通过plt.legend()函数来添加图例。以下是一个简单的例子:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
plt.plot(x, y1, label='y = x^2')
plt.plot(x, y2, label='y = x')
plt.legend()
plt.show()
在这个例子中,我们使用label参数为每条线条设置标签,然后通过plt.legend()函数来添加图例。
二、设置图例标题
除了添加图例之外,我们还可以为图例设置标题。可以使用plt.legend()函数的title参数来实现。以下是一个示例:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
plt.plot(x, y1, label='y = x^2')
plt.plot(x, y2, label='y = x')
plt.legend(title='Functions')
plt.show()
在这个例子中,我们通过设置plt.legend()函数的title参数,将图例的标题设置为“Functions”。
三、调整图例位置
有时我们需要将图例放置在特定的位置。可以使用plt.legend()函数的loc参数来设置图例的位置。以下是一个示例:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
plt.plot(x, y1, label='y = x^2')
plt.plot(x, y2, label='y = x')
plt.legend(title='Functions', loc='upper left')
plt.show()
在这个例子中,我们将图例的位置设置在图表的左上角。
四、自定义图例样式
我们还可以进一步自定义图例的样式,例如改变图例框的颜色、线条样式、透明度等。以下是一个示例:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
plt.plot(x, y1, label='y = x^2')
plt.plot(x, y2, label='y = x')
legend = plt.legend(title='Functions', loc='upper left')
legend.get_frame().set_edgecolor('red')
legend.get_frame().set_linewidth(2)
legend.get_frame().set_alpha(0.5)
plt.show()
在这个例子中,我们通过获取图例的框架(legend.get_frame()),然后设置框架的边框颜色、线条宽度和透明度。
五、更多图例自定义选项
Matplotlib提供了许多选项来自定义图例的外观和行为。以下是一些常用的选项:
- fontsize: 设置图例文本的字体大小。
- ncol: 设置图例的列数。
- frameon: 是否显示图例的边框。
- shadow: 是否在图例后面添加阴影。
- fancybox: 是否使用圆角边框。
- borderpad: 图例边框和内容之间的填充。
- labelspacing: 图例条目之间的垂直间距。
以下是一个示例,展示了如何使用这些选项:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
plt.plot(x, y1, label='y = x^2')
plt.plot(x, y2, label='y = x')
plt.legend(title='Functions', loc='upper left', fontsize='large', ncol=2, frameon=True, shadow=True, fancybox=True, borderpad=1, labelspacing=1)
plt.show()
在这个例子中,我们使用了多个选项来自定义图例的外观,包括字体大小、列数、边框、阴影、圆角边框、边框填充和条目间距。
六、图例与bbox_to_anchor参数
有时我们需要将图例放置在图表的特定位置,而不是使用预定义的位置。可以使用bbox_to_anchor参数来实现。这允许我们指定图例的边界框位置。以下是一个示例:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
plt.plot(x, y1, label='y = x^2')
plt.plot(x, y2, label='y = x')
plt.legend(title='Functions', bbox_to_anchor=(1.05, 1), loc='upper left')
plt.show()
在这个例子中,我们使用bbox_to_anchor参数将图例放置在图表的右上角之外。
七、多子图中的图例
当我们绘制多个子图时,可以为每个子图分别设置图例。以下是一个示例,展示了如何在多个子图中添加图例:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
fig, (ax1, ax2) = plt.subplots(1, 2)
ax1.plot(x, y1, label='y = x^2')
ax1.legend(title='Function 1', loc='upper left')
ax2.plot(x, y2, label='y = x')
ax2.legend(title='Function 2', loc='upper left')
plt.show()
在这个例子中,我们创建了两个子图,并分别为每个子图添加了图例。
八、图例与不同的绘图类型
无论是折线图、柱状图、散点图还是其他类型的图表,都可以使用plt.legend()函数来添加图例。以下是几个示例,展示了不同类型图表中的图例:
1. 折线图
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
plt.plot(x, y1, label='y = x^2')
plt.plot(x, y2, label='y = x')
plt.legend(title='Functions')
plt.show()
2. 柱状图
import matplotlib.pyplot as plt
x = ['A', 'B', 'C', 'D']
y1 = [10, 15, 20, 25]
y2 = [5, 10, 15, 20]
plt.bar(x, y1, label='Group 1')
plt.bar(x, y2, bottom=y1, label='Group 2')
plt.legend(title='Groups')
plt.show()
3. 散点图
import matplotlib.pyplot as plt
x1 = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
x2 = [1, 2, 3, 4, 5]
y2 = [1, 2, 3, 4, 5]
plt.scatter(x1, y1, label='Group 1')
plt.scatter(x2, y2, label='Group 2')
plt.legend(title='Groups')
plt.show()
九、图例与其他库的结合
Matplotlib可以与其他数据可视化库结合使用,如Seaborn和Pandas等。在这些库中,我们同样可以通过plt.legend()函数来添加图例。以下是一些示例:
1. Seaborn
import seaborn as sns
import matplotlib.pyplot as plt
tips = sns.load_dataset('tips')
sns.scatterplot(x='total_bill', y='tip', hue='day', data=tips)
plt.legend(title='Day')
plt.show()
2. Pandas
import pandas as pd
import matplotlib.pyplot as plt
data = {
'x': [1, 2, 3, 4, 5],
'y1': [1, 4, 9, 16, 25],
'y2': [1, 2, 3, 4, 5]
}
df = pd.DataFrame(data)
df.plot(x='x', y=['y1', 'y2'], kind='line')
plt.legend(title='Functions')
plt.show()
十、总结
通过以上内容,我们了解了如何在Python中使用Matplotlib库来添加图例标题、调整图例位置、自定义图例样式以及结合其他数据可视化库使用图例。掌握这些技巧可以帮助我们创建更加专业和美观的数据可视化图表。无论是简单的图例添加,还是复杂的图例自定义,都可以通过Matplotlib提供的丰富参数来实现。希望本文能够帮助你在数据可视化过程中更好地使用图例。
相关问答FAQs:
如何在Python中创建图例标题?
在Python中使用Matplotlib库时,可以通过在legend()
函数中使用title
参数来添加图例标题。例如,可以使用以下代码实现:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], label='数据1')
plt.plot([3, 2, 1], label='数据2')
plt.legend(title='图例标题')
plt.show()
这样,图例将会显示指定的标题。
可以自定义图例标题的样式吗?
确实可以。通过传递prop
参数,您可以自定义图例标题的字体、大小和颜色。例如:
plt.legend(title='图例标题', title_fontsize='13', title_fontweight='bold')
这将使图例标题更具视觉吸引力。
在多个子图中如何设置统一的图例标题?
在使用subplots
创建多个图形时,可以通过单独的legend
调用为每个子图设置相同的图例标题。可以在循环中实现,例如:
fig, axs = plt.subplots(2, 2)
for ax in axs.flat:
ax.plot([1, 2, 3], label='数据')
ax.legend(title='图例标题')
plt.show()
这样,即使在不同的子图中,每个图例都可以拥有相同的标题。
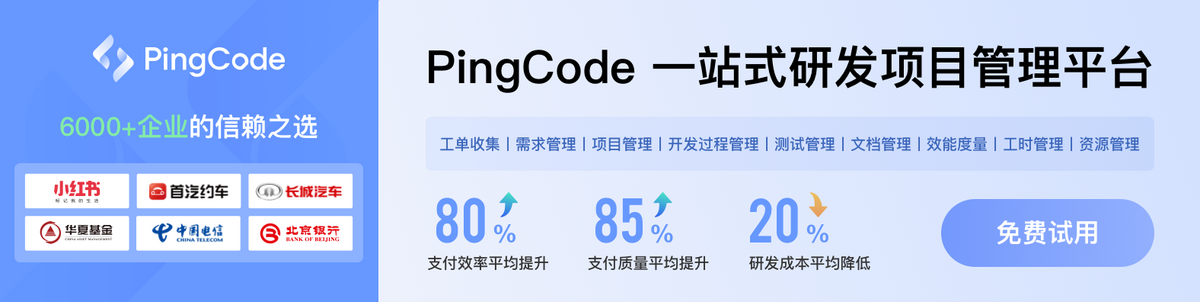