Python写入错误可以通过检查代码逻辑、捕获异常、使用事务管理、重试机制、日志记录等方式解决。
一、检查代码逻辑
在编写代码时,确保逻辑正确是避免写入错误的首要步骤。详细检查代码,确保每个步骤都按预期执行,特别是文件路径、文件模式和数据格式。
- 文件路径和权限
确保文件路径正确,且有相应的读写权限。如果路径错误或权限不足,会导致写入失败。
import os
file_path = 'path/to/file.txt'
if not os.path.exists(os.path.dirname(file_path)):
os.makedirs(os.path.dirname(file_path))
with open(file_path, 'w') as file:
file.write('Hello, World!')
- 数据格式
确保要写入的数据格式正确。例如,写入字符串到文本文件,而非直接写入对象。
data = {'key': 'value'}
with open(file_path, 'w') as file:
file.write(str(data))
二、捕获异常
使用异常处理机制,捕获并处理潜在的写入错误。这样可以避免程序崩溃,并记录错误信息以便后续排查。
- 基本异常处理
try:
with open(file_path, 'w') as file:
file.write('Hello, World!')
except IOError as e:
print(f'An IOError occurred: {e.strerror}')
- 详细异常处理
捕获更具体的异常,并做出相应处理。
try:
with open(file_path, 'w') as file:
file.write('Hello, World!')
except FileNotFoundError:
print('File not found.')
except PermissionError:
print('Permission denied.')
except IOError as e:
print(f'An IOError occurred: {e.strerror}')
三、使用事务管理
在涉及多个文件操作时,使用事务管理确保所有操作成功后才写入文件。如果其中任何一步失败,可以回滚操作,避免部分写入带来的数据不一致问题。
- 实现简单事务管理
class FileTransaction:
def __init__(self, file_path):
self.file_path = file_path
self.temp_path = file_path + '.tmp'
def __enter__(self):
self.file = open(self.temp_path, 'w')
return self.file
def __exit__(self, exc_type, exc_val, exc_tb):
self.file.close()
if exc_type is None:
os.rename(self.temp_path, self.file_path)
else:
os.remove(self.temp_path)
with FileTransaction(file_path) as file:
file.write('Hello, World!')
四、重试机制
在某些情况下,写入错误可能是暂时的。引入重试机制,可以在一定次数内重新尝试写入操作,提高成功率。
- 实现简单重试机制
import time
def write_with_retry(file_path, data, retries=3, delay=2):
for attempt in range(retries):
try:
with open(file_path, 'w') as file:
file.write(data)
return
except IOError as e:
print(f'Attempt {attempt + 1} failed: {e.strerror}')
time.sleep(delay)
print('All attempts failed.')
write_with_retry(file_path, 'Hello, World!')
五、日志记录
记录写入操作和错误信息,有助于排查问题。使用Python的logging
模块,可以方便地记录日志信息。
- 配置日志记录
import logging
logging.basicConfig(filename='file_operations.log', level=logging.INFO)
def write_to_file(file_path, data):
try:
with open(file_path, 'w') as file:
file.write(data)
logging.info(f'Write successful: {file_path}')
except IOError as e:
logging.error(f'Write failed: {file_path}, Error: {e.strerror}')
write_to_file(file_path, 'Hello, World!')
六、文件锁定机制
在多进程或多线程环境下,确保文件操作的原子性,避免多个进程或线程同时写入同一个文件。
- 使用文件锁
from filelock import FileLock
lock_path = file_path + '.lock'
lock = FileLock(lock_path)
with lock:
with open(file_path, 'w') as file:
file.write('Hello, World!')
七、清理和恢复机制
在发生写入错误后,清理和恢复机制可以确保文件状态一致,避免数据损坏。
- 实现清理机制
def clean_up(file_path):
try:
os.remove(file_path)
print(f'Cleaned up: {file_path}')
except OSError as e:
print(f'Cleanup failed: {file_path}, Error: {e.strerror}')
try:
with open(file_path, 'w') as file:
file.write('Hello, World!')
except IOError as e:
print(f'Write failed: {e.strerror}')
clean_up(file_path)
八、验证数据完整性
写入操作完成后,验证数据完整性,确保数据正确写入。可以通过读取文件并校验内容的方式进行验证。
- 验证写入数据
def verify_data(file_path, expected_data):
with open(file_path, 'r') as file:
actual_data = file.read()
return actual_data == expected_data
data = 'Hello, World!'
with open(file_path, 'w') as file:
file.write(data)
if verify_data(file_path, data):
print('Data verified successfully.')
else:
print('Data verification failed.')
九、使用高级库
使用高级库和框架,可以简化文件操作,并提供更多的功能和错误处理机制。例如,使用pandas
进行数据处理和写入CSV文件。
- 使用pandas写入文件
import pandas as pd
data = {'col1': [1, 2], 'col2': [3, 4]}
df = pd.DataFrame(data)
try:
df.to_csv(file_path, index=False)
print('Write successful.')
except IOError as e:
print(f'Write failed: {e.strerror}')
十、定期备份和版本控制
定期备份文件,使用版本控制系统(如Git)管理文件变更,可以在发生写入错误时,快速恢复到正确版本。
- 实现文件备份
import shutil
def backup_file(file_path):
backup_path = file_path + '.bak'
shutil.copy(file_path, backup_path)
print(f'Backup created: {backup_path}')
try:
backup_file(file_path)
with open(file_path, 'w') as file:
file.write('Hello, World!')
except IOError as e:
print(f'Write failed: {e.strerror}')
shutil.copy(file_path + '.bak', file_path)
print('Restored from backup.')
总结
写入错误是常见的编程问题,通过检查代码逻辑、捕获异常、使用事务管理、引入重试机制、记录日志、使用文件锁、清理和恢复机制、验证数据完整性、使用高级库、定期备份和版本控制等方法,可以有效解决和预防写入错误,确保数据安全和程序稳定运行。在实际开发中,结合多种方法,针对具体问题进行处理,可以更好地保障文件操作的可靠性和安全性。
相关问答FAQs:
如何检测Python代码中的写入错误?
在Python中,常见的写入错误可能源于文件权限、路径错误或数据格式问题。使用try-except块可以捕捉这些错误,并通过输出错误信息来帮助你定位问题。例如,试图写入一个只读文件时,可以捕获PermissionError
并进行相应处理。
Python中如何安全地删除文件?
要安全地删除文件,可以使用os
模块中的remove()
函数。在删除之前,建议先检查文件是否存在,可以使用os.path.exists()
进行验证。这样可以避免因文件不存在而引发的异常。
如果我误删了文件,如何恢复它?
误删文件后,恢复的可能性取决于操作系统和文件系统的特性。在Windows中,可以检查回收站;在Linux中,可以使用extundelete
等工具。定期备份重要文件也是预防数据丢失的有效方法。
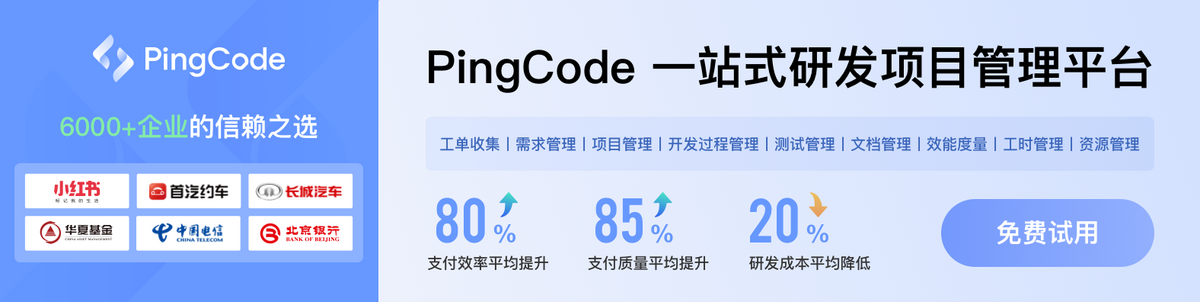