新版Python使用Tkinter的方法主要包括安装Tkinter、导入Tkinter模块、创建主窗口、添加控件、事件处理等。下面将详细介绍其中的安装Tkinter。
安装Tkinter
Tkinter通常已经包含在Python的标准库中,因此在大多数情况下不需要单独安装。如果使用的是标准的Python发行版,只需确保Python已经安装并且版本是最新的。可以通过命令行输入python --version
来检查Python的版本。
一、导入Tkinter模块
在使用Tkinter之前,首先需要导入Tkinter模块。新版Python中,Tkinter模块已经被重命名为tkinter
(小写),并且其子模块ttk
(Themed Tk)也需要导入。示例如下:
import tkinter as tk
from tkinter import ttk
二、创建主窗口
主窗口是Tkinter GUI程序的基础。可以使用Tk()
类来创建一个主窗口。示例如下:
root = tk.Tk()
root.title("My Tkinter Application")
root.geometry("800x600")
在上述代码中,title
方法用于设置窗口的标题,geometry
方法用于设置窗口的大小。
三、添加控件
控件是Tkinter GUI的基本元素,包括按钮、标签、文本框等。每个控件都有一个对应的类,例如Button
、Label
、Entry
等。示例如下:
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
button = tk.Button(root, text="Click Me", command=lambda: print("Button Clicked"))
button.pack()
在上述代码中,Label
控件用于显示文本,Button
控件用于创建一个按钮,并且通过command
参数绑定一个事件处理函数。
四、布局管理
布局管理器用于控制控件在窗口中的位置。Tkinter提供了三种布局管理器:pack
、grid
和place
。下面介绍每种布局管理器的基本用法。
1、Pack布局管理器
pack
布局管理器是最简单的一种布局管理器。它按照顺序将控件添加到窗口中,可以使用side
参数指定控件的排列方向(top
、bottom
、left
、right
)。示例如下:
label1 = tk.Label(root, text="Label 1")
label1.pack(side=tk.TOP)
label2 = tk.Label(root, text="Label 2")
label2.pack(side=tk.BOTTOM)
2、Grid布局管理器
grid
布局管理器将控件放置在一个二维网格中,可以使用row
和column
参数指定控件的位置。示例如下:
label1 = tk.Label(root, text="Label 1")
label1.grid(row=0, column=0)
label2 = tk.Label(root, text="Label 2")
label2.grid(row=1, column=1)
3、Place布局管理器
place
布局管理器允许精确控制控件的位置和大小,可以使用x
、y
、width
和height
参数。示例如下:
label1 = tk.Label(root, text="Label 1")
label1.place(x=50, y=50, width=100, height=30)
label2 = tk.Label(root, text="Label 2")
label2.place(x=200, y=100, width=100, height=30)
五、事件处理
事件处理是指在用户与GUI控件交互时,程序对事件作出响应。例如,用户点击按钮时,程序执行特定的操作。在Tkinter中,可以使用command
参数或bind
方法来绑定事件处理函数。
1、使用command参数
大多数控件都支持command
参数,例如按钮控件。示例如下:
def on_button_click():
print("Button Clicked")
button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack()
2、使用bind方法
bind
方法可以绑定更多类型的事件,例如键盘事件、鼠标事件等。示例如下:
def on_key_press(event):
print(f"Key Pressed: {event.keysym}")
root.bind("<KeyPress>", on_key_press)
在上述代码中,"<KeyPress>"
表示键盘按下事件,event
对象包含了事件的详细信息,例如按下的键。
六、常用控件介绍
Tkinter提供了丰富的控件,下面介绍一些常用的控件及其用法。
1、Label控件
Label
控件用于显示文本或图像。示例如下:
label = tk.Label(root, text="This is a Label", font=("Arial", 14), fg="blue")
label.pack()
在上述代码中,font
参数用于设置字体,fg
参数用于设置前景色。
2、Button控件
Button
控件用于创建按钮。示例如下:
button = tk.Button(root, text="Submit", command=lambda: print("Submitted"))
button.pack()
3、Entry控件
Entry
控件用于创建单行文本框。示例如下:
entry = tk.Entry(root)
entry.pack()
def get_entry_value():
value = entry.get()
print(f"Entry Value: {value}")
button = tk.Button(root, text="Get Value", command=get_entry_value)
button.pack()
4、Text控件
Text
控件用于创建多行文本框。示例如下:
text = tk.Text(root, height=10, width=40)
text.pack()
def get_text_value():
value = text.get("1.0", tk.END)
print(f"Text Value: {value}")
button = tk.Button(root, text="Get Text", command=get_text_value)
button.pack()
5、Listbox控件
Listbox
控件用于创建列表框。示例如下:
listbox = tk.Listbox(root)
listbox.pack()
listbox.insert(tk.END, "Item 1")
listbox.insert(tk.END, "Item 2")
listbox.insert(tk.END, "Item 3")
def get_selected_item():
selected_index = listbox.curselection()
if selected_index:
value = listbox.get(selected_index)
print(f"Selected Item: {value}")
button = tk.Button(root, text="Get Selected Item", command=get_selected_item)
button.pack()
七、对话框和文件对话框
Tkinter提供了多种对话框,例如消息对话框、文件对话框等,方便用户进行交互操作。
1、消息对话框
消息对话框用于显示信息、警告或错误消息。可以使用messagebox
模块来创建消息对话框。示例如下:
from tkinter import messagebox
def show_info():
messagebox.showinfo("Information", "This is an info message")
button = tk.Button(root, text="Show Info", command=show_info)
button.pack()
2、文件对话框
文件对话框用于打开或保存文件。可以使用filedialog
模块来创建文件对话框。示例如下:
from tkinter import filedialog
def open_file():
file_path = filedialog.askopenfilename()
if file_path:
print(f"Selected File: {file_path}")
button = tk.Button(root, text="Open File", command=open_file)
button.pack()
八、菜单和工具栏
菜单和工具栏是GUI应用程序中常见的元素,用于提供快捷操作。
1、菜单
可以使用Menu
类来创建菜单。示例如下:
menu = tk.Menu(root)
root.config(menu=menu)
file_menu = tk.Menu(menu, tearoff=0)
menu.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="Open", command=lambda: print("Open File"))
file_menu.add_command(label="Save", command=lambda: print("Save File"))
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.quit)
2、工具栏
可以使用Frame
和Button
控件来创建工具栏。示例如下:
toolbar = tk.Frame(root, bd=1, relief=tk.RAISED)
open_button = tk.Button(toolbar, text="Open", command=lambda: print("Open File"))
open_button.pack(side=tk.LEFT, padx=2, pady=2)
save_button = tk.Button(toolbar, text="Save", command=lambda: print("Save File"))
save_button.pack(side=tk.LEFT, padx=2, pady=2)
toolbar.pack(side=tk.TOP, fill=tk.X)
九、Tkinter高级功能
Tkinter还提供了一些高级功能,例如定时器、拖放操作、自定义控件等。
1、定时器
可以使用after
方法来创建定时器。示例如下:
def update_label():
label.config(text="Updated!")
root.after(1000, update_label)
label = tk.Label(root, text="Waiting...")
label.pack()
root.after(1000, update_label)
在上述代码中,每隔1秒钟(1000毫秒)更新一次标签的文本。
2、拖放操作
可以使用dnd
模块来实现拖放操作。示例如下:
from tkinterdnd2 import TkinterDnD, DND_FILES
def drop(event):
print(f"Dropped File: {event.data}")
root = TkinterDnD.Tk()
label = tk.Label(root, text="Drag and Drop a File Here")
label.pack(padx=10, pady=10)
label.drop_target_register(DND_FILES)
label.dnd_bind('<<Drop>>', drop)
十、Tkinter应用程序实例
下面是一个完整的Tkinter应用程序实例,展示了如何使用上述内容创建一个简单的文本编辑器。
import tkinter as tk
from tkinter import filedialog, messagebox, ttk
class TextEditor:
def __init__(self, root):
self.root = root
self.root.title("Text Editor")
self.root.geometry("800x600")
self.create_menu()
self.create_toolbar()
self.create_text_widget()
def create_menu(self):
menu = tk.Menu(self.root)
self.root.config(menu=menu)
file_menu = tk.Menu(menu, tearoff=0)
menu.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="New", command=self.new_file)
file_menu.add_command(label="Open", command=self.open_file)
file_menu.add_command(label="Save", command=self.save_file)
file_menu.add_command(label="Save As", command=self.save_as_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=self.root.quit)
edit_menu = tk.Menu(menu, tearoff=0)
menu.add_cascade(label="Edit", menu=edit_menu)
edit_menu.add_command(label="Cut", command=lambda: self.root.focus_get().event_generate('<<Cut>>'))
edit_menu.add_command(label="Copy", command=lambda: self.root.focus_get().event_generate('<<Copy>>'))
edit_menu.add_command(label="Paste", command=lambda: self.root.focus_get().event_generate('<<Paste>>'))
def create_toolbar(self):
toolbar = tk.Frame(self.root, bd=1, relief=tk.RAISED)
new_button = tk.Button(toolbar, text="New", command=self.new_file)
new_button.pack(side=tk.LEFT, padx=2, pady=2)
open_button = tk.Button(toolbar, text="Open", command=self.open_file)
open_button.pack(side=tk.LEFT, padx=2, pady=2)
save_button = tk.Button(toolbar, text="Save", command=self.save_file)
save_button.pack(side=tk.LEFT, padx=2, pady=2)
toolbar.pack(side=tk.TOP, fill=tk.X)
def create_text_widget(self):
self.text_widget = tk.Text(self.root, wrap='word')
self.text_widget.pack(expand=1, fill='both')
scrollbar = ttk.Scrollbar(self.root, command=self.text_widget.yview)
scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
self.text_widget.config(yscrollcommand=scrollbar.set)
def new_file(self):
self.text_widget.delete(1.0, tk.END)
def open_file(self):
file_path = filedialog.askopenfilename(filetypes=[("Text files", "*.txt"), ("All files", "*.*")])
if file_path:
with open(file_path, 'r') as file:
content = file.read()
self.text_widget.delete(1.0, tk.END)
self.text_widget.insert(tk.INSERT, content)
def save_file(self):
file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text files", "*.txt"), ("All files", "*.*")])
if file_path:
with open(file_path, 'w') as file:
content = self.text_widget.get(1.0, tk.END)
file.write(content)
def save_as_file(self):
self.save_file()
if __name__ == "__main__":
root = tk.Tk()
app = TextEditor(root)
root.mainloop()
在上述代码中,TextEditor
类封装了文本编辑器的功能,包括菜单、工具栏和文本框的创建,以及文件操作的实现。通过实例化TextEditor
类并传递root
窗口对象,完成了整个应用程序的构建。
总结
通过以上内容的介绍,新版Python中使用Tkinter创建GUI应用程序的基本方法和技巧已经涵盖。无论是基本的控件创建、布局管理,还是高级功能的应用,都可以帮助开发者快速上手并构建出功能丰富的GUI应用程序。希望这篇文章能为您提供实用的参考和帮助。
相关问答FAQs:
新版Python中如何安装tkinter库?
tkinter是Python的标准GUI库,通常在Python安装时就已经包含。如果在你的Python环境中找不到tkinter,可以通过以下方式安装:在终端或命令行中运行pip install tk
。确保你的Python版本支持tkinter,通常Python 3.x版本都自带此库。
使用tkinter创建简单窗口的基本步骤是什么?
创建一个简单的tkinter窗口可以通过以下步骤实现:首先,导入tkinter库。接着,创建一个主窗口实例。然后,可以设置窗口的标题和大小。最后,调用主循环mainloop()
以启动事件处理。示例代码如下:
import tkinter as tk
root = tk.Tk()
root.title("我的第一个窗口")
root.geometry("400x300")
root.mainloop()
如何在tkinter中添加按钮和处理按钮事件?
在tkinter中添加按钮非常简单。首先,使用Button
类创建按钮并设置其文本和命令。命令是指当按钮被点击时所执行的函数。以下是一个示例:
import tkinter as tk
def on_button_click():
print("按钮被点击了!")
root = tk.Tk()
button = tk.Button(root, text="点击我", command=on_button_click)
button.pack()
root.mainloop()
通过这种方式,用户点击按钮时,on_button_click
函数将被调用,控制台会输出相应的信息。
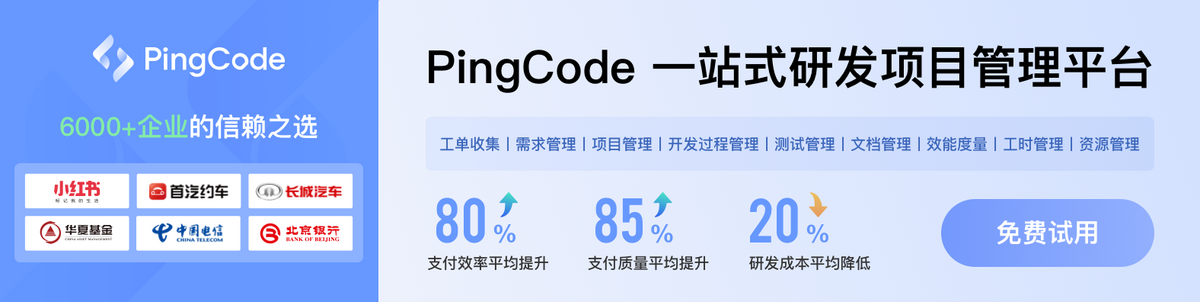