Python函数实现输出的方法有多种,包括:使用print()函数、返回值、日志记录、写入文件等。其中,最常见的方式是使用print()
函数和返回值。使用print()
函数可以直接在控制台输出结果,适用于调试和简单的输出需求;而返回值则能将结果传递给调用者,适合函数间调用和进一步处理。在此,我们详细讨论下使用print()
函数的实现方法。
print()
函数是Python中最基本的输出函数,能够将任何数据类型的内容输出到控制台。它的使用非常简单,只需要将需要输出的内容作为参数传递给print()
函数即可。例如:
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
在上述代码中,greet
函数通过print()
函数输出了一条问候语。这种方式适合快速查看函数的输出结果,但在更复杂的场景中,可能需要结合其他方法如返回值或日志记录来实现更灵活的输出。
一、使用 print()
函数
print()
函数是Python中最常见的输出方法之一。它可以将任何数据类型的内容输出到控制台,并且支持多种格式化输出方式。以下是一些常用的用法。
1. 基本用法
最简单的用法是直接将字符串、整数、浮点数等数据类型作为参数传递给 print()
函数。例如:
def display_basic():
print("Hello, World!")
print(42)
print(3.14159)
display_basic()
这段代码将输出:
Hello, World!
42
3.14159
2. 多参数输出
print()
函数可以接受多个参数,并将它们依次输出,参数之间用空格分隔。例如:
def display_multiple():
print("The answer is", 42, "and the value of pi is", 3.14159)
display_multiple()
这段代码将输出:
The answer is 42 and the value of pi is 3.14159
3. 格式化输出
为了提高输出的可读性,print()
函数支持多种格式化输出方法,包括使用 %
操作符、str.format()
方法和 f-string(格式化字符串)等。
def display_formatted():
name = "Alice"
age = 30
height = 1.75
# 使用 % 操作符
print("Name: %s, Age: %d, Height: %.2f" % (name, age, height))
# 使用 str.format() 方法
print("Name: {}, Age: {}, Height: {:.2f}".format(name, age, height))
# 使用 f-string
print(f"Name: {name}, Age: {age}, Height: {height:.2f}")
display_formatted()
这段代码将输出:
Name: Alice, Age: 30, Height: 1.75
Name: Alice, Age: 30, Height: 1.75
Name: Alice, Age: 30, Height: 1.75
二、返回值
除了使用 print()
函数直接输出外,函数还可以通过返回值将结果传递给调用者,以便进一步处理或输出。返回值可以是任何数据类型,包括字符串、整数、浮点数、列表、字典等。
1. 单个返回值
函数可以返回一个单一的值,如下例所示:
def add(a, b):
return a + b
result = add(10, 5)
print(result)
这段代码将输出:
15
2. 多个返回值
函数还可以返回多个值,返回的多个值会被打包成一个元组。例如:
def calculate(a, b):
sum_result = a + b
diff_result = a - b
return sum_result, diff_result
sum_result, diff_result = calculate(10, 5)
print("Sum:", sum_result)
print("Difference:", diff_result)
这段代码将输出:
Sum: 15
Difference: 5
三、日志记录
在实际开发中,直接使用 print()
函数输出信息虽然方便,但在复杂项目中不够灵活。因此,Python提供了 logging
模块来记录日志信息,支持多种日志级别和输出方式。
1. 基本用法
logging
模块提供了 debug()
, info()
, warning()
, error()
, critical()
等方法用于记录不同级别的日志信息。例如:
import logging
def log_example():
logging.basicConfig(level=logging.DEBUG)
logging.debug("This is a debug message")
logging.info("This is an info message")
logging.warning("This is a warning message")
logging.error("This is an error message")
logging.critical("This is a critical message")
log_example()
这段代码将输出:
DEBUG:root:This is a debug message
INFO:root:This is an info message
WARNING:root:This is a warning message
ERROR:root:This is an error message
CRITICAL:root:This is a critical message
2. 配置日志输出
可以通过配置文件或代码来设置日志输出格式、日志级别、日志文件等。例如:
import logging
def configure_logging():
logging.basicConfig(filename='app.log', filemode='w', format='%(name)s - %(levelname)s - %(message)s', level=logging.DEBUG)
logging.debug("This is a debug message")
logging.info("This is an info message")
logging.warning("This is a warning message")
logging.error("This is an error message")
logging.critical("This is a critical message")
configure_logging()
这段代码将日志信息输出到 app.log
文件中。
四、写入文件
除了使用 print()
函数和返回值外,还可以将函数的输出写入文件。这在需要保存输出结果以供后续分析时非常有用。
1. 写入文本文件
可以使用 open()
函数打开一个文件,并使用 write()
方法将内容写入文件。例如:
def write_to_file():
with open('output.txt', 'w') as file:
file.write("Hello, World!\n")
file.write("This is a test.\n")
write_to_file()
这段代码将在当前目录下创建一个名为 output.txt
的文件,并将内容写入其中。
2. 写入CSV文件
对于结构化数据,可以使用 csv
模块将输出写入CSV文件。例如:
import csv
def write_to_csv():
data = [
["Name", "Age", "Height"],
["Alice", 30, 1.75],
["Bob", 25, 1.80]
]
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
write_to_csv()
这段代码将在当前目录下创建一个名为 output.csv
的文件,并将数据写入其中。
五、结合多种方法
在实际开发中,可能需要结合多种方法来实现灵活的输出。例如,可以先将函数的结果返回给调用者,然后由调用者决定是直接输出、记录日志还是写入文件。
import logging
def calculate(a, b):
sum_result = a + b
diff_result = a - b
return sum_result, diff_result
def main():
logging.basicConfig(level=logging.DEBUG, filename='app.log', filemode='w', format='%(name)s - %(levelname)s - %(message)s')
a, b = 10, 5
sum_result, diff_result = calculate(a, b)
print("Sum:", sum_result)
print("Difference:", diff_result)
logging.info(f"Sum: {sum_result}")
logging.info(f"Difference: {diff_result}")
with open('output.txt', 'w') as file:
file.write(f"Sum: {sum_result}\n")
file.write(f"Difference: {diff_result}\n")
if __name__ == "__main__":
main()
这段代码将:
- 计算两个数的和和差。
- 将结果输出到控制台。
- 将结果记录到日志文件
app.log
。 - 将结果写入文本文件
output.txt
。
六、总结
Python函数实现输出的方法多种多样,包括使用 print()
函数、返回值、日志记录和写入文件等。具体选择哪种方法,取决于具体需求和应用场景。对于简单的输出需求,可以直接使用 print()
函数;对于函数间调用和进一步处理,返回值是更好的选择;对于需要记录日志和保存输出结果,可以使用 logging
模块和文件操作。结合多种方法,可以实现灵活且高效的输出。
相关问答FAQs:
如何在Python中定义一个函数来输出信息?
在Python中,可以使用def
关键字定义一个函数。函数体内可以使用print()
语句来输出信息。例如,定义一个简单的函数可以如下所示:
def greet(name):
print(f"Hello, {name}!")
调用此函数时,只需传入一个参数:
greet("Alice") # 输出: Hello, Alice!
在Python函数中可以输出哪些类型的数据?
Python函数可以输出多种数据类型,包括字符串、数字、列表、字典等。你可以通过将这些数据转换为字符串格式来使用print()
函数进行输出。例如:
def display_data(data):
print(f"Data: {data}")
无论传入何种类型的数据,函数都能够将其输出。
如何使用返回值而不是直接输出?
在Python中,函数可以使用return
语句返回值,而不是直接使用print()
进行输出。返回的值可以在函数外部进行处理。例如:
def add(a, b):
return a + b
result = add(5, 3) # result 现在包含8
print(result) # 输出: 8
这种方法使得函数更灵活,可以在不同的上下文中使用返回值。
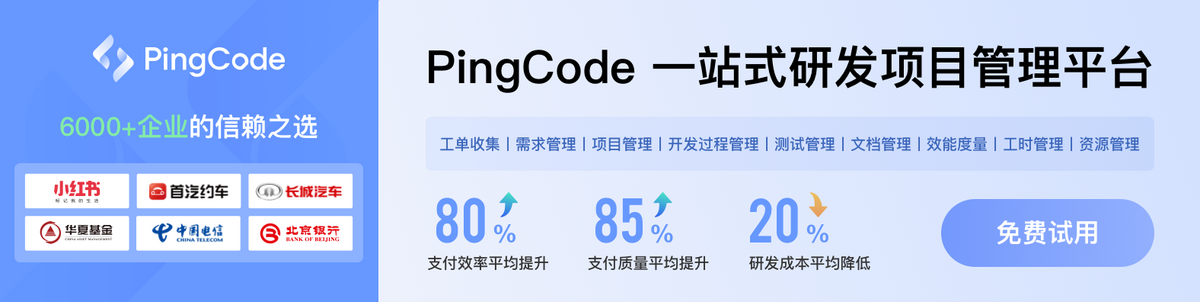