在Python中,添加和使用cfg(configuration)模块主要是为了处理配置文件。常见的配置文件格式包括INI、YAML、JSON等。本文将直接回答如何在Python中添加cfg模块,并详细介绍如何使用各类配置文件格式。
在Python中,可以使用configparser模块、PyYAML库、json模块来处理配置文件。其中,configparser模块主要用于处理INI文件,PyYAML库用于处理YAML文件,json模块用于处理JSON文件。我们将详细介绍如何使用configparser模块来处理INI配置文件。
一、CONFIGPARSER 模块(INI 文件)
1. 安装和导入
configparser是Python内置模块,无需额外安装。直接导入即可:
import configparser
2. 创建和读取配置文件
首先,我们需要一个配置文件,假设文件名为config.ini
,内容如下:
[DEFAULT]
ServerAliveInterval = 45
Compression = yes
CompressionLevel = 9
ForwardX11 = yes
[bitbucket.org]
User = hg
[topsecret.server.com]
Port = 50022
ForwardX11 = no
读取该配置文件可以使用以下代码:
config = configparser.ConfigParser()
config.read('config.ini')
访问配置值
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
user = config['bitbucket.org']['User']
port = config['topsecret.server.com']['Port']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"User: {user}")
print(f"Port: {port}")
3. 修改和写入配置文件
可以修改配置文件中的值,并将其写回文件:
config['DEFAULT']['ServerAliveInterval'] = '60'
config['bitbucket.org']['User'] = 'new_user'
with open('config.ini', 'w') as configfile:
config.write(configfile)
二、PYAML 库(YAML 文件)
1. 安装和导入
如果要处理YAML文件,需要安装PyYAML库:
pip install pyyaml
导入库:
import yaml
2. 创建和读取YAML文件
假设有一个YAML配置文件,内容如下:
default:
server_alive_interval: 45
compression: yes
compression_level: 9
forward_x11: yes
bitbucket.org:
user: hg
topsecret.server.com:
port: 50022
forward_x11: no
读取该配置文件可以使用以下代码:
with open('config.yaml', 'r') as file:
config = yaml.safe_load(file)
访问配置值
server_alive_interval = config['default']['server_alive_interval']
compression = config['default']['compression']
user = config['bitbucket.org']['user']
port = config['topsecret.server.com']['port']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"User: {user}")
print(f"Port: {port}")
3. 修改和写入YAML文件
可以修改配置文件中的值,并将其写回文件:
config['default']['server_alive_interval'] = 60
config['bitbucket.org']['user'] = 'new_user'
with open('config.yaml', 'w') as file:
yaml.safe_dump(config, file)
三、JSON 模块(JSON 文件)
1. 导入
json是Python内置模块,无需额外安装。直接导入即可:
import json
2. 创建和读取JSON文件
假设有一个JSON配置文件,内容如下:
{
"default": {
"server_alive_interval": 45,
"compression": "yes",
"compression_level": 9,
"forward_x11": "yes"
},
"bitbucket.org": {
"user": "hg"
},
"topsecret.server.com": {
"port": 50022,
"forward_x11": "no"
}
}
读取该配置文件可以使用以下代码:
with open('config.json', 'r') as file:
config = json.load(file)
访问配置值
server_alive_interval = config['default']['server_alive_interval']
compression = config['default']['compression']
user = config['bitbucket.org']['user']
port = config['topsecret.server.com']['port']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"User: {user}")
print(f"Port: {port}")
3. 修改和写入JSON文件
可以修改配置文件中的值,并将其写回文件:
config['default']['server_alive_interval'] = 60
config['bitbucket.org']['user'] = 'new_user'
with open('config.json', 'w') as file:
json.dump(config, file, indent=4)
四、总结
在Python中,处理配置文件非常常见,不同的配置文件格式有不同的处理方法。configparser模块、PyYAML库和json模块分别用于处理INI、YAML和JSON文件。根据具体需求选择合适的模块和文件格式,可以有效管理和维护应用的配置。通过学习如何创建、读取、修改和写入配置文件,可以让你的Python应用更加灵活和易于维护。
相关问答FAQs:
如何在Python项目中使用cfg模块进行配置管理?
cfg模块提供了一种方便的方式来管理项目的配置文件。您可以通过安装相关的库(例如configparser或pycfg)来实现配置管理。首先,确保您已经安装了所需的模块,然后创建一个配置文件,最后在代码中读取这些配置项,从而轻松管理项目的不同环境设置。
cfg模块与其他配置管理工具相比有哪些优势?
cfg模块的主要优势在于其简单易用和灵活性。与复杂的框架相比,cfg模块允许开发者以简单的方式处理配置。它通常支持多种格式(如INI、YAML等),并且可以根据项目需求自定义配置项,使得管理不同环境的设置变得更为高效。
如何处理cfg模块中的错误和异常?
在使用cfg模块时,处理错误和异常是确保程序稳定运行的关键。可以通过try-except语句捕获读取配置文件时可能出现的IOError或KeyError等异常。此外,建议在加载配置文件之前检查文件是否存在,并在程序中提供默认值,以确保应用在缺少特定配置项时仍能正常运行。
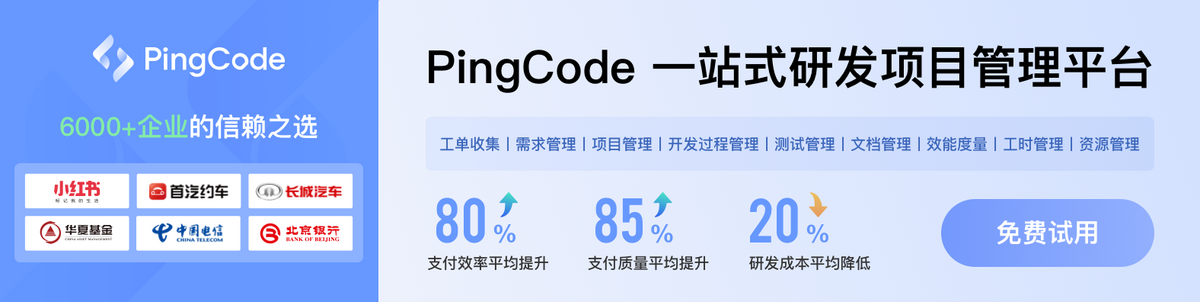