Python爬虫可以通过使用合适的HTTP库、解析HTML的库、模拟浏览器行为的库来查找和提取网页上的销量信息。例如,可以使用Requests库发送HTTP请求获取网页内容,使用BeautifulSoup解析HTML结构,使用Selenium模拟浏览器操作。下面我们将详细介绍如何使用这些工具进行爬虫查销量。
一、使用HTTP库获取网页内容
1、Requests库
Requests是一个简单且功能强大的HTTP库,可以轻松地发送HTTP请求并接收响应。要使用Requests库,首先需要安装它:
pip install requests
然后可以通过以下代码发送一个HTTP GET请求获取网页内容:
import requests
url = 'https://www.example.com/product-page'
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print('Failed to retrieve the page')
2、Headers和Cookies
有些网站可能会检测请求的Headers和Cookies,以防止爬虫访问。可以通过设置请求头和携带Cookies来模拟浏览器行为:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
}
cookies = {
'session_id': 'your_session_id_here'
}
response = requests.get(url, headers=headers, cookies=cookies)
二、解析HTML内容
1、BeautifulSoup库
BeautifulSoup是一个用于解析HTML和XML文档的库,可以轻松地提取网页上的数据。首先需要安装BeautifulSoup库:
pip install beautifulsoup4
然后可以使用BeautifulSoup解析网页内容:
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
2、查找元素
使用BeautifulSoup可以通过标签、类名、ID等方式查找网页中的元素。例如,查找包含销量信息的元素:
sales_info = soup.find('span', class_='sales-number')
if sales_info:
sales_number = sales_info.text
print(f'Sales number: {sales_number}')
else:
print('Sales information not found')
三、模拟浏览器行为
1、Selenium库
Selenium是一个用于自动化Web浏览器操作的库,可以模拟用户在浏览器中的操作,适用于需要执行JavaScript动态加载内容的网页。首先安装Selenium库和对应的浏览器驱动(如ChromeDriver):
pip install selenium
下载ChromeDriver后,将其路径添加到系统环境变量中。
2、使用Selenium获取网页内容
from selenium import webdriver
driver = webdriver.Chrome()
driver.get(url)
等待页面加载完成,可以使用显式等待
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
try:
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, 'sales-number'))
)
sales_number = element.text
print(f'Sales number: {sales_number}')
finally:
driver.quit()
四、处理反爬虫机制
1、使用代理IP
有些网站会通过检测IP地址的频繁访问来阻止爬虫,可以使用代理IP来避免这种情况:
proxies = {
'http': 'http://your_proxy_ip:port',
'https': 'http://your_proxy_ip:port',
}
response = requests.get(url, headers=headers, cookies=cookies, proxies=proxies)
2、随机等待时间
通过在每次请求之间添加随机的等待时间,可以模拟人类用户的行为,减少被检测到的概率:
import time
import random
wait_time = random.uniform(1, 5) # 随机等待1到5秒
time.sleep(wait_time)
五、实际案例
1、爬取电商网站的销量信息
以某电商网站为例,假设我们要爬取某个产品的销量信息:
import requests
from bs4 import BeautifulSoup
import time
import random
url = 'https://www.example.com/product-page'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
}
cookies = {
'session_id': 'your_session_id_here'
}
response = requests.get(url, headers=headers, cookies=cookies)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
sales_info = soup.find('span', class_='sales-number')
if sales_info:
sales_number = sales_info.text
print(f'Sales number: {sales_number}')
else:
print('Sales information not found')
else:
print('Failed to retrieve the page')
随机等待时间
wait_time = random.uniform(1, 5)
time.sleep(wait_time)
2、使用Selenium处理动态加载内容
有些网站的销量信息是通过JavaScript动态加载的,可以使用Selenium来处理:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import time
import random
url = 'https://www.example.com/product-page'
driver = webdriver.Chrome()
try:
driver.get(url)
# 等待页面加载完成
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, 'sales-number'))
)
sales_number = element.text
print(f'Sales number: {sales_number}')
finally:
driver.quit()
随机等待时间
wait_time = random.uniform(1, 5)
time.sleep(wait_time)
六、数据存储
1、存储到CSV文件
可以将爬取到的销量信息存储到CSV文件中,方便后续处理:
import csv
with open('sales_data.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Product', 'Sales'])
writer.writerow(['Product A', sales_number])
2、存储到数据库
可以将爬取到的数据存储到数据库中,例如MySQL:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
cursor.execute("INSERT INTO sales_data (product, sales) VALUES (%s, %s)", ('Product A', sales_number))
connection.commit()
cursor.close()
connection.close()
七、处理异常和错误
在实际爬虫过程中,可能会遇到各种异常和错误,需要处理这些情况以确保程序的稳定运行:
try:
response = requests.get(url, headers=headers, cookies=cookies)
response.raise_for_status() # 检查HTTP状态码
soup = BeautifulSoup(response.text, 'html.parser')
sales_info = soup.find('span', class_='sales-number')
if sales_info:
sales_number = sales_info.text
print(f'Sales number: {sales_number}')
else:
print('Sales information not found')
except requests.exceptions.RequestException as e:
print(f'An error occurred: {e}')
八、优化和扩展
1、多线程爬虫
为了提高爬取效率,可以使用多线程来同时爬取多个页面:
import threading
def crawl_page(url):
response = requests.get(url, headers=headers, cookies=cookies)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
sales_info = soup.find('span', class_='sales-number')
if sales_info:
sales_number = sales_info.text
print(f'Sales number for {url}: {sales_number}')
else:
print(f'Sales information not found for {url}')
else:
print(f'Failed to retrieve the page for {url}')
urls = ['https://www.example.com/product-page1', 'https://www.example.com/product-page2', ...]
threads = []
for url in urls:
thread = threading.Thread(target=crawl_page, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2、分布式爬虫
对于更大规模的爬虫任务,可以使用分布式爬虫框架,如Scrapy,并结合分布式任务队列,如Celery或RabbitMQ。
九、总结
通过使用Python的Requests库、BeautifulSoup库、Selenium库以及处理反爬虫机制,可以有效地爬取网页上的销量信息。可以根据具体需求选择合适的工具和方法,并通过多线程或分布式爬虫提高爬取效率。最后,将爬取到的数据存储到CSV文件或数据库中,以便后续分析和处理。
相关问答FAQs:
如何使用Python爬虫获取电商产品的销量数据?
使用Python爬虫获取电商平台的销量数据通常需要访问网页并提取特定信息。可以使用库如Requests和BeautifulSoup进行网页请求和数据解析。首先,确保你熟悉目标网站的结构,找到销量信息的位置。接着,编写代码进行数据抓取,并注意遵循网站的robots.txt文件以避免违反使用条款。
在爬虫中如何处理反爬机制以获取销量信息?
很多电商网站会实施反爬措施,例如IP封禁、验证码等。为了有效绕过这些措施,可以采用代理IP、设置请求头模拟浏览器行为,或利用时间间隔控制请求频率。此外,使用selenium库可以模拟用户浏览行为,帮助抓取动态加载的内容。
如何存储和分析爬取到的销量数据?
在爬取到销量数据后,存储和分析是重要的步骤。可以将数据存储在CSV文件、数据库(如SQLite、MySQL)或使用Pandas库进行数据处理和分析。利用数据可视化工具(如Matplotlib或Seaborn)可以帮助你更好地理解销量趋势和变化,从而为后续决策提供依据。
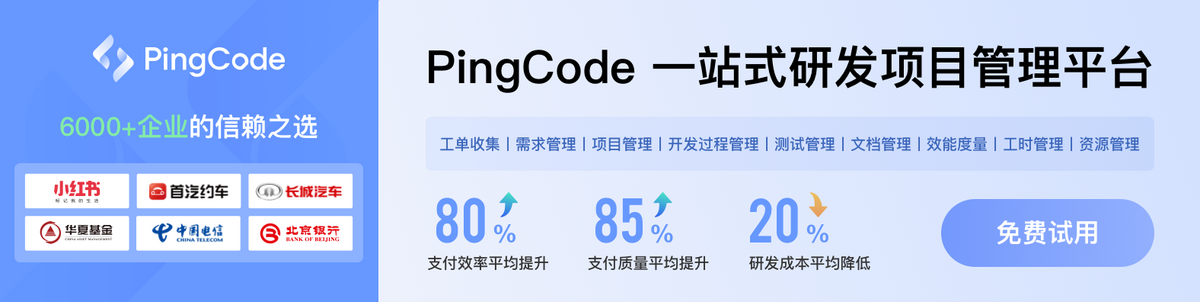